adding multiple entries to a HashMap at once in one statement
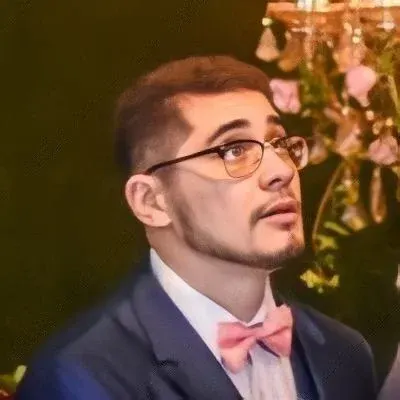
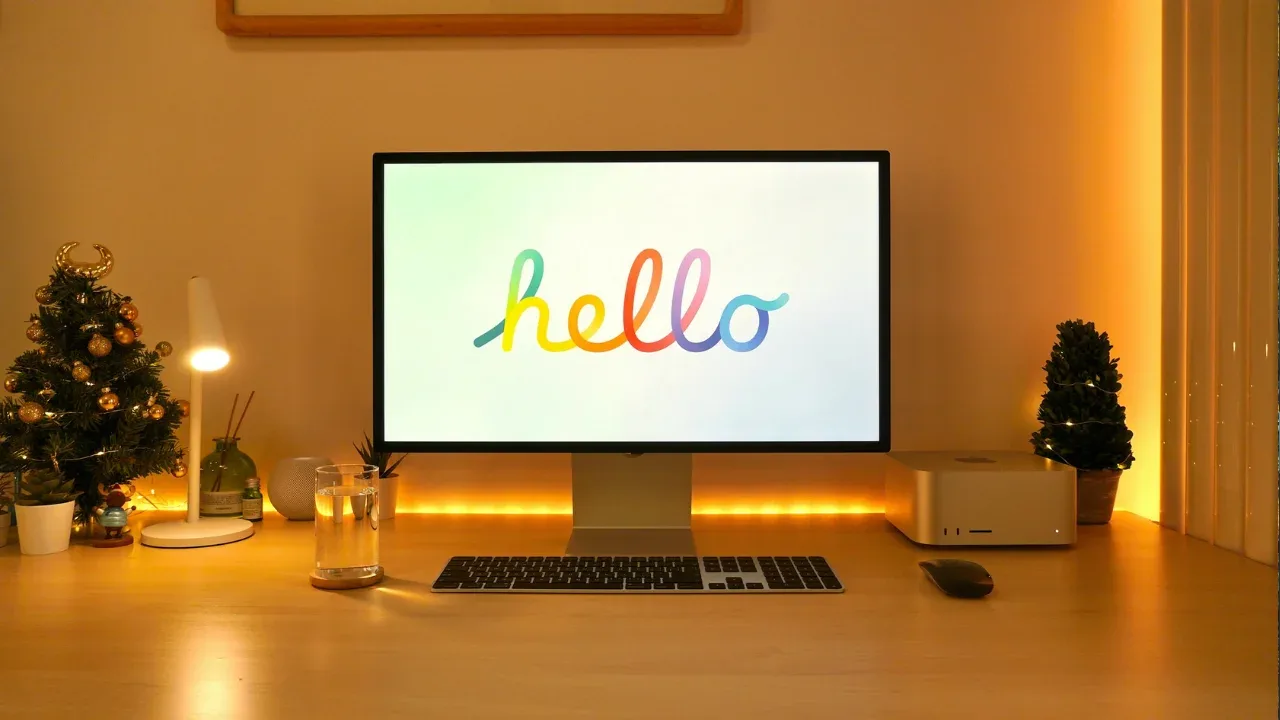
How to Add Multiple Entries to a HashMap in One Statement
Are you tired of adding multiple entries to a HashMap one by one? Do you want a more efficient way to initialize your constant HashMap in just one line? Look no further! In this blog post, we will explore different solutions to this problem and provide you with an easy, one-line statement to add multiple entries to a HashMap.
The Common Problem
Let's start by understanding the problem at hand. Imagine you have a HashMap that you want to populate with multiple entries. Traditionally, you would write individual put
statements for each entry, like this:
hashMap.put("One", new Integer(1));
hashMap.put("Two", new Integer(2));
hashMap.put("Three", new Integer(3));
But what if you have tens or even hundreds of entries? This approach becomes cumbersome and time-consuming. You need a more efficient way to initialize your HashMap with multiple entries.
The Objective-C Inspiration
Interestingly, in Objective-C, you can achieve this in a single line using NSDictionary
and NSNumber
. Here's an example of how it is done:
[NSDictionary dictionaryWithObjectsAndKeys:
@"w",[NSNumber numberWithInt:1],
@"K",[NSNumber numberWithInt:2],
@"e",[NSNumber numberWithInt:4],
@"z",[NSNumber numberWithInt:5],
@"l",[NSNumber numberWithInt:6],
nil]
Unfortunately, in Java, there is no built-in method to do this directly with HashMaps. However, fear not! We have a few clever solutions to offer.
Solution 1: Using Guava
Guava is a powerful library that provides many useful utilities for Java developers. One of its features is the ImmutableMap
class, which allows you to create a HashMap in a concise and readable way.
First, you need to include the Guava library in your project. You can do this by adding the following dependency to your pom.xml
:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.1-jre</version>
</dependency>
Once you have Guava set up, you can create your HashMap in a single statement, like this:
import com.google.common.collect.ImmutableMap;
Map<String, Integer> hashMap = ImmutableMap.<String, Integer>builder()
.put("One", 1)
.put("Two", 2)
.put("Three", 3)
.build();
Voila! You have added multiple entries to your HashMap in just one statement using Guava's ImmutableMap
.
Solution 2: Creating a Utility Method
If you don't want to introduce an external library like Guava, you can create a utility method that simplifies the process for you. This method encapsulates the repetitive put
statements, making your code cleaner and more maintainable.
Here's an example of how you can create a utility method to add multiple entries to a HashMap:
import java.util.HashMap;
import java.util.Map;
public class HashMapUtils {
public static <K, V> Map<K, V> createHashMap(Object... keyValues) {
if (keyValues.length % 2 != 0) {
throw new IllegalArgumentException("Invalid number of arguments. Key-value pairs are expected.");
}
Map<K, V> hashMap = new HashMap<>();
for (int i = 0; i < keyValues.length; i += 2) {
K key = (K) keyValues[i];
V value = (V) keyValues[i + 1];
hashMap.put(key, value);
}
return hashMap;
}
}
With this utility method in place, you can now add multiple entries to your HashMap in one line, like this:
Map<String, Integer> hashMap = HashMapUtils.createHashMap(
"One", 1,
"Two", 2,
"Three", 3
);
Conclusion
Adding multiple entries to a HashMap in one statement can greatly improve code readability and maintainability. In this blog post, we explored two solutions: using Guava's ImmutableMap
or creating a utility method. Whether you choose to leverage an external library or create your own utility method, both approaches provide a convenient way to initialize your HashMap efficiently.
So why waste time with repetitive put
statements when you can embrace a more concise and elegant solution? Give these methods a try in your next project, and let us know what you think!
Don't forget to share this blog post with your fellow developers and spread the knowledge. Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
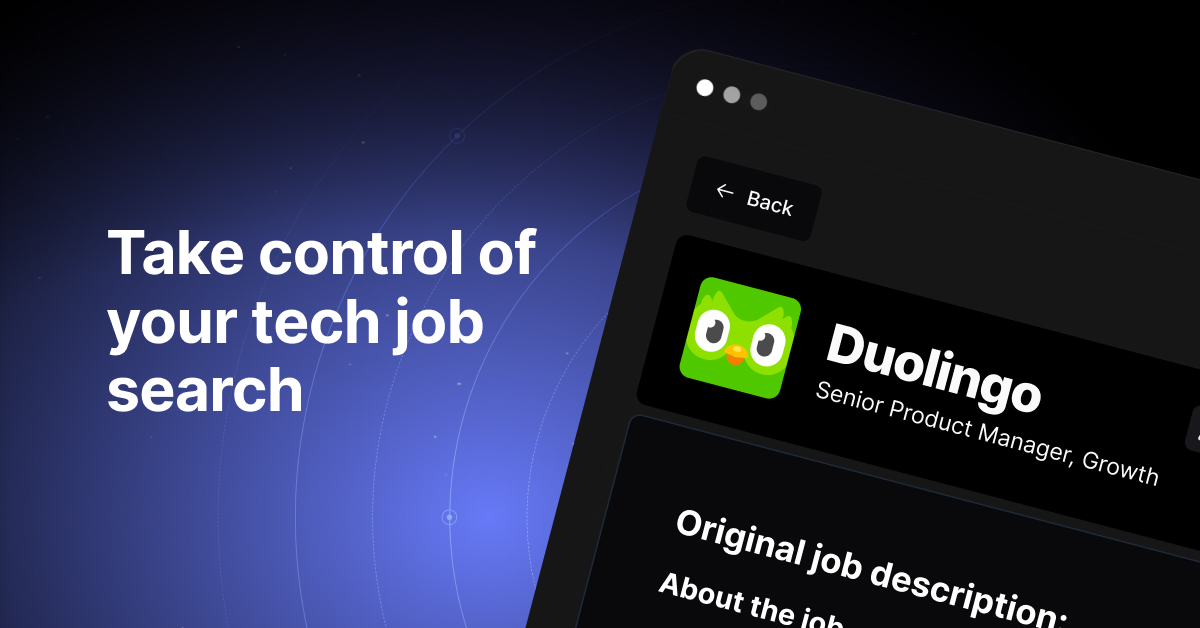