Add context path to Spring Boot application
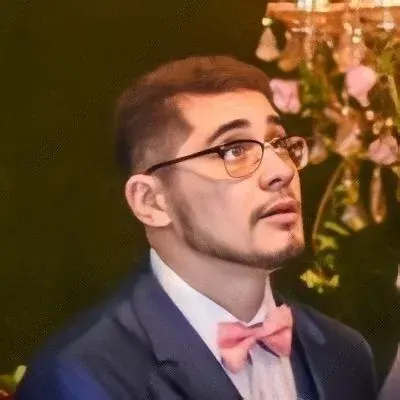
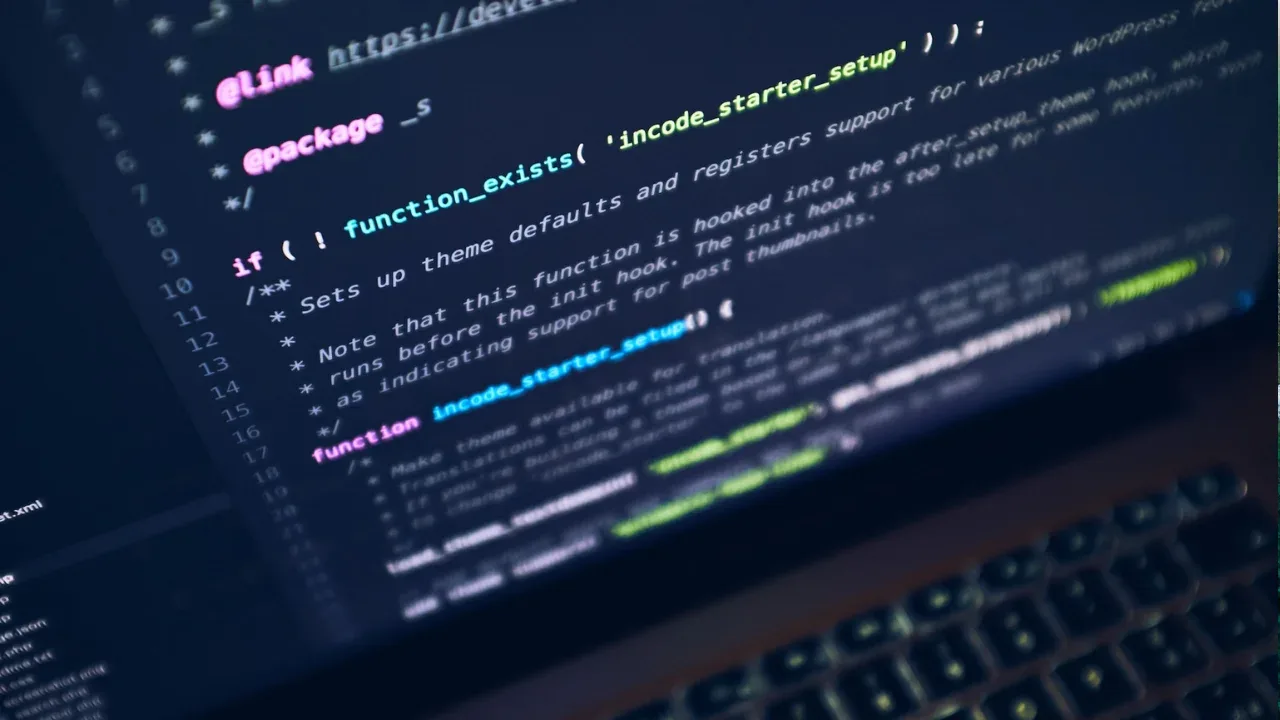
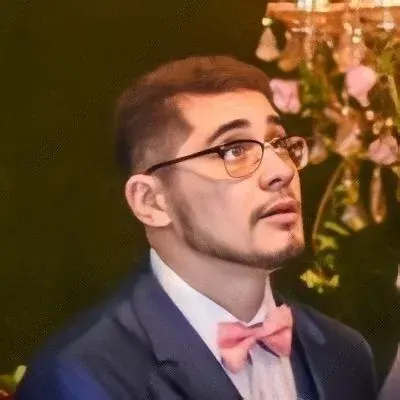
Adding a Context Path to a Spring Boot Application 🌱
Do you want to set a context root for your Spring Boot application? In other words, you want your app to be accessed from localhost:port/{app_name} while having all the controller paths append to it? We've got you covered! Here's a step-by-step guide to address this issue and get your application working as expected. 😎
Getting Started 🚀
Before we dive into the code, let's have a look at the application configuration file for the web-app. You'll find it under ApplicationConfiguration.java. It should look something like this:
@Configuration
public class ApplicationConfiguration {
Logger logger = LoggerFactory.getLogger(ApplicationConfiguration.class);
@Value("${mainstay.web.port:12378}")
private String port;
@Value("${mainstay.web.context:/mainstay}")
private String context;
// ... other code
@Bean
public EmbeddedServletContainerFactory servletContainer(){
TomcatEmbeddedServletContainerFactory factory = new TomcatEmbeddedServletContainerFactory();
logger.info("Setting custom configuration for Mainstay:");
logger.info("Setting port to {}",port);
logger.info("Setting context to {}",context);
factory.setPort(Integer.valueOf(port));
factory.setContextPath(context);
// ... other code
return factory;
}
// ... other code
}
As you can see, we're using a TomcatEmbeddedServletContainerFactory to configure the servlet container. You may have noticed the setContextPath method being called with the context variable. This is where the magic happens!
The Problem 🧐
When you run your application, you might notice that the new root is not being appended to the URL as expected. For example, even though you set the context path to /mainstay
, you might still find your application located at localhost:12378 instead of localhost:12378/mainstay.
The Solution 💡
To fix this issue, let's make sure we have everything set up correctly. First, check the index controller for the main page. It should be similar to the following code snippet:
@Controller
public class IndexController {
Logger logger = LoggerFactory.getLogger(IndexController.class);
@RequestMapping("/")
public String index(Model model) {
logger.info("Setting index page title to Mainstay - Web");
model.addAttribute("title","Mainstay - Web");
return "index";
}
}
To make use of the context root, we need to update the @RequestMapping annotation to include the context path. Here's the modified code snippet:
@Controller
@RequestMapping("${mainstay.web.context:/mainstay}")
public class IndexController {
Logger logger = LoggerFactory.getLogger(IndexController.class);
@RequestMapping("/")
public String index(Model model) {
logger.info("Setting index page title to Mainstay - Web");
model.addAttribute("title","Mainstay - Web");
return "index";
}
}
With the updated code, when you access the root URL (localhost:12378/mainstay), you should see the main page with the correct title.
Recap 📝
To recap, to add a context path to a Spring Boot application, you need to:
Update the @RequestMapping annotation in your controllers to include the context path.
Make sure the setContextPath method is called correctly in your ApplicationConfiguration.java file.
Conclusion 🎉
By following these steps, you should have successfully added a context path to your Spring Boot application. Now your app can be accessed from localhost:port/{app_name} with all the controller paths appended to it, providing a clean and organized URL structure. Stay tuned for more awesome tech tips and tricks!
Have any questions or tips to share? Let us know in the comments below. 👇