A quick and easy way to join array elements with a separator (the opposite of split) in Java
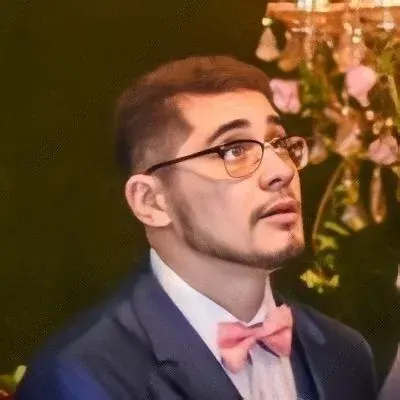
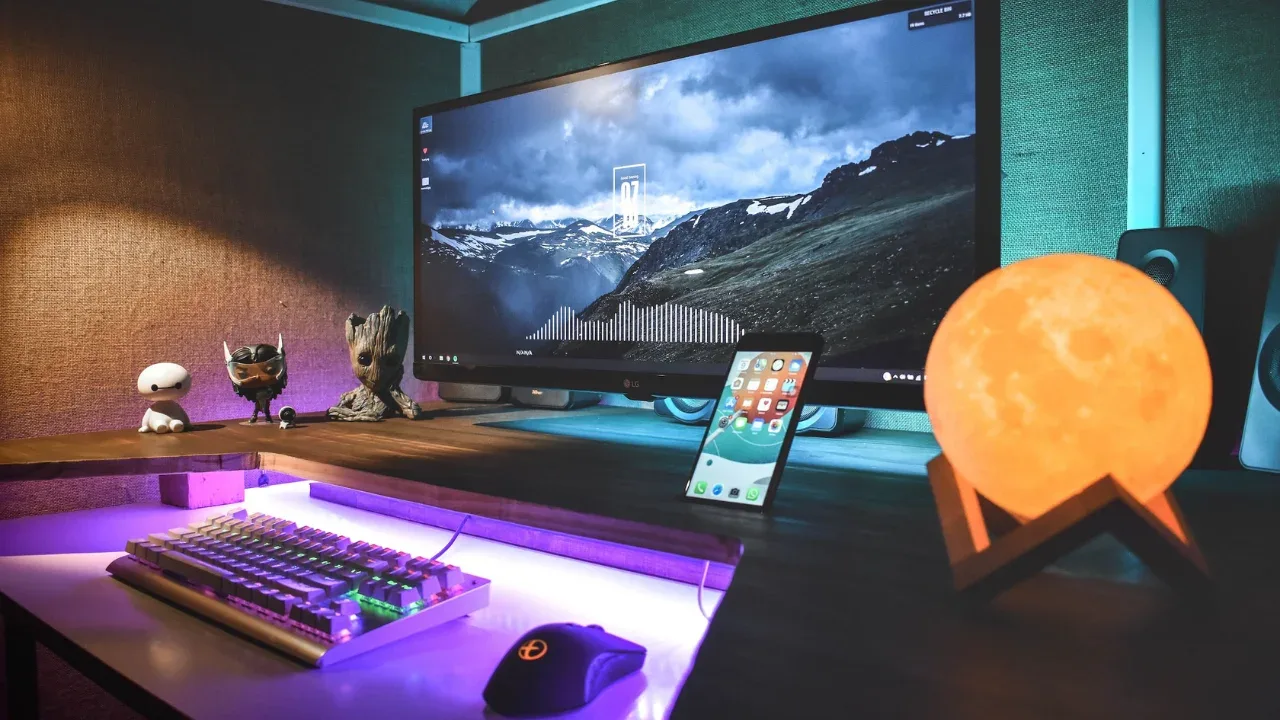
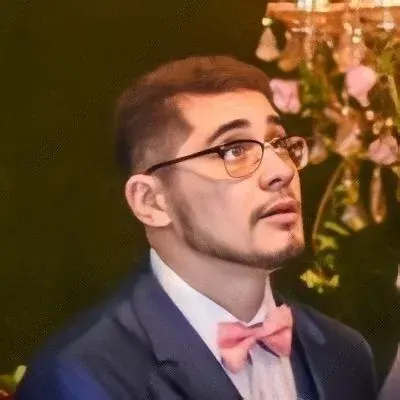
💥 Joining Array Elements with a Separator in Java: The Easy Way! 💥
Are you tired of struggling with the opposite of the split
function in Java? Don't worry, we've got you covered! In this blog post, we'll discuss a quick and easy way to join array elements with a separator in Java, so you can transform ["a","b","c"]
into the elegant and concise "a,b,c"
.
But before we dive into the solution, let's take a quick look at the problem itself. The original poster on Stack Overflow pointed out that iterating through an array requires extra conditions or substring manipulation to achieve the desired result. This can be cumbersome and error-prone, which makes it imperative to find a more efficient and certified solution.
The good news is, there's no need to reinvent the wheel here! Java provides a simple and effective solution to this problem. Allow us to introduce you to the String.join
method, which is part of the Java standard library since version 8.
String result = String.join(",", array);
In just one line of code, you can concatenate all the elements of array
, using ","
as the separator, into a single String
named result
. How cool is that?
But what if you're working with a collection of objects, instead of an array of strings? No problem! Just make sure that the elements in your collection have a suitable toString
implementation, and you're good to go.
List<Person> people = new ArrayList<>();
// Add your objects to the list...
String result = people.stream().map(Person::toString).collect(Collectors.joining(","));
By using the join
method from the Collectors
class along with the map
method from the Stream API, you can easily convert your collection of objects into a comma-separated string.
🚀 Take It to the Next Level
Now that you know this neat little trick, imagine the possibilities! You can use it for logging purposes, generating CSV files, or even creating custom representations of your data. Get creative and explore the full potential of joining array elements with a separator in Java.
📢 Share Your Wisdom
We would love to hear how you prefer to join array elements in your projects. Do you have any ingenious hacks or alternative solutions to share? Let us know in the comments below! Your insights could be invaluable to fellow developers facing a similar challenge.
So go ahead, try out the String.join
method in your code and let us know how it works for you. Happy coding! 😄👩💻👨💻
References: