A for-loop to iterate over an enum in Java
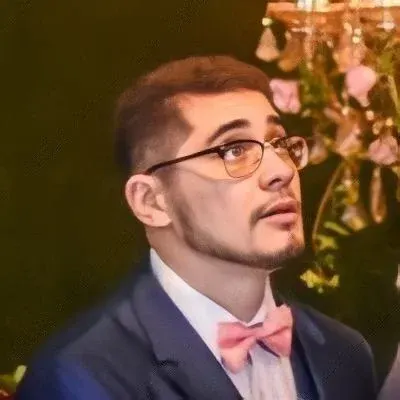
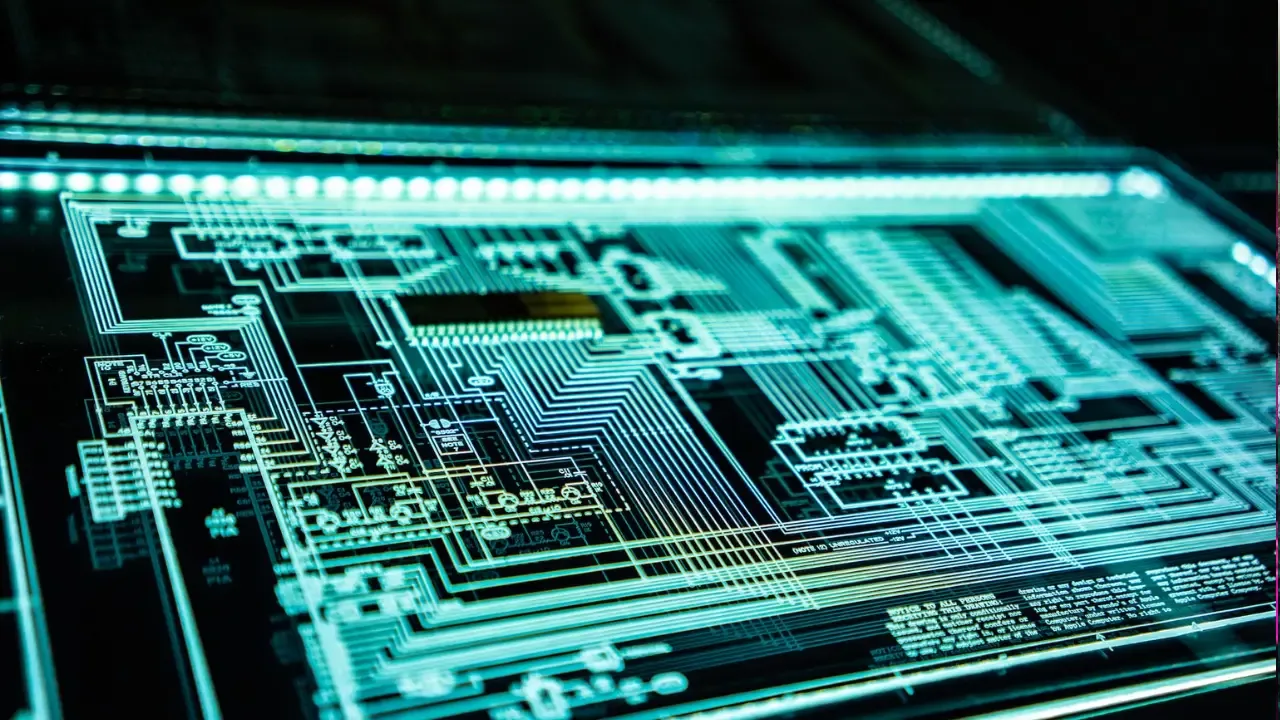
🌟 Mastering Enum Iteration in Java 🌟
Welcome to another exciting blog post where we unravel the mysteries of programming! Today, we'll tackle the question that has been bugging many Java developers: How can I write a for
loop that iterates through each value of an enum?
Enums are a powerful feature in Java that allow you to define a set of constant values. They can represent a fixed number of predefined options, like the cardinal and intermediate directions in our case. Let's take a closer look at how we can iterate over an enum using a for
loop:
public enum Direction {
NORTH, NORTHEAST, EAST, SOUTHEAST, SOUTH, SOUTHWEST, WEST, NORTHWEST
}
🤔 The Challenge
So, you have an enum called Direction
with all the different directions you can think of. Now, you want to traverse this enum and do something with each direction.
💡 The Solution
To iterate over the enum values, we can use the values()
method provided by Java. This method returns an array containing the enum constants in the order they were declared.
To accomplish this, follow these steps:
Retrieve the enum values using the
values()
method:Direction[] directions = Direction.values();
Iterate over the enum values using a
for
loop:for (Direction direction : directions) { // Perform your desired actions on each direction // You can access the direction using the 'direction' variable // For example, System.out.println(direction) to print the direction }
That's it! 🎉 You now have a for
loop that iterates over each value of your enum!
🚀 Take it to the Next Level
With the basic knowledge of enum iteration, you can explore further possibilities and enhance your code. Here are some ideas to spark your imagination:
Calculate the opposite direction for each enum value.
Use a switch statement inside the loop to perform different actions based on the direction.
Implement a
toString()
method in theDirection
enum to customize the direction's string representation.
Remember, the sky's the limit! 😄
✨ Share Your Experiences
Now that you have a powerful tool in your coding arsenal, it's time to put it to use! Share your experiences and let us know how this enum iteration technique helped you in your projects. We would love to hear about any creative ideas or challenges you encountered.
Feel free to leave a comment below and engage in a lively discussion with fellow developers. Together, we can make the programming world a better place! 🌍💻
Happy coding! 👨💻🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
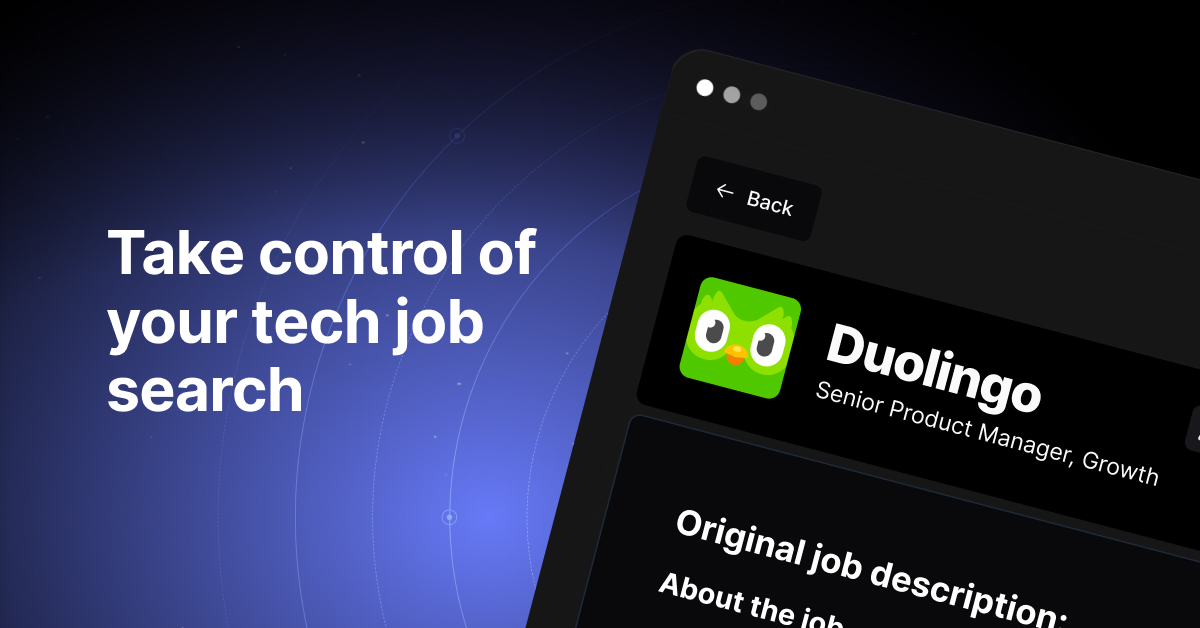