UILongPressGestureRecognizer gets called twice when pressing down
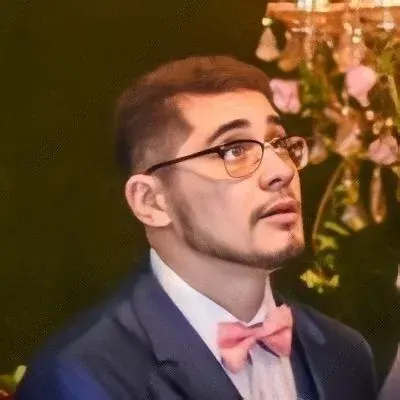
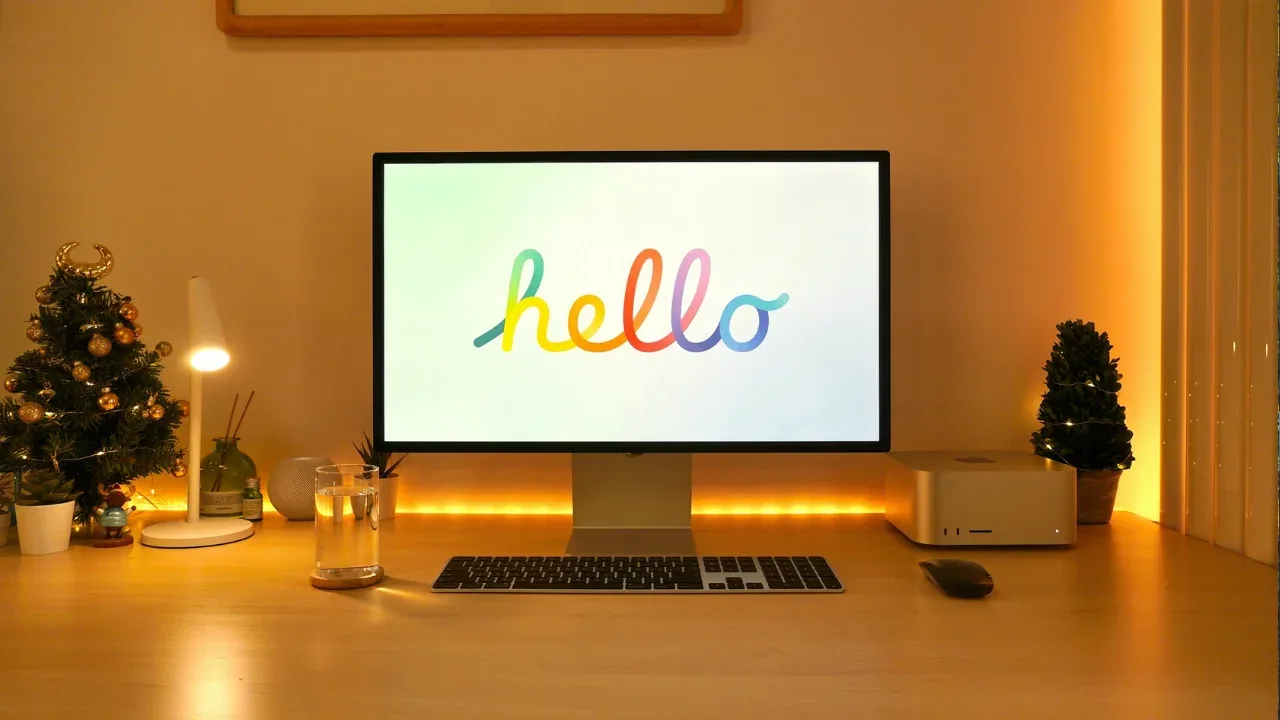
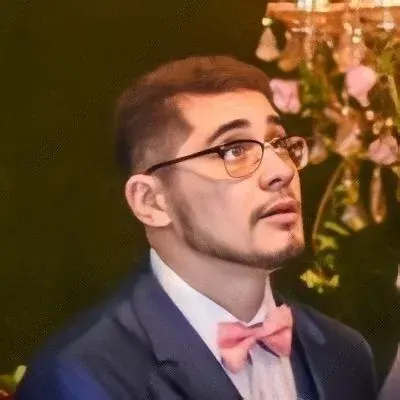
Fixing the Issue of UILongPressGestureRecognizer Getting Called Twice
š Hey there, tech enthusiasts! In today's blog post, we're going to tackle a common issue that some iOS developers might encounter when using the UILongPressGestureRecognizer
. š±š„ Specifically, we'll address why this recognizer's handler method gets called twice instead of just once when you press down for longer than the specified duration. š®
Before diving into the solutions, let's quickly go over the code snippet provided for some context:
UILongPressGestureRecognizer *longPress = [[UILongPressGestureRecognizer alloc]
initWithTarget:self
action:@selector(handleLongPress:)];
longPress.minimumPressDuration = 2.0;
[self addGestureRecognizer:longPress];
[longPress release];
The code above sets up a UILongPressGestureRecognizer
instance, longPress
, and assigns it a target and an action using the initWithTarget:action:
method. The minimumPressDuration
property is also set to 2.0 seconds, indicating that the gesture should be recognized after holding down for at least that duration. Finally, the gesture recognizer is added to a view and released.
The handler method for the long press is defined as follows:
-(void)handleLongPress:(UILongPressGestureRecognizer*)recognizer{
NSLog(@"double oo");
}
Now, let's address the question at hand: Why is the handleLongPress:
method getting called twice when you press down for longer than 2 seconds? And, more importantly, how can you fix this behavior? š¤
Understanding the Issue
The reason for this behavior is not a bug or a mistake in your code but rather an intended feature of UILongPressGestureRecognizer
. When you press down and hold your finger for longer than the specified minimumPressDuration
, the recognizer initially fires one gesture event for the "began" state and then continuously repeats the "changed" state events at a predefined interval. š
This "began" event is the first time the gesture is recognized, while the "changed" events are triggered continuously until you release your finger. Therefore, when you hold down for a longer duration, both the "began" event and multiple "changed" events occur, resulting in the handler method being called multiple times. ā°
Solving the Issue
Now that we understand why the handleLongPress:
method is triggered twice, let's look at two possible solutions to address this issue and ensure that the handler only runs once.
Solution 1: Use Gesture Recognizer State
One way to handle this is by checking the state
property of UILongPressGestureRecognizer
within your method implementation. By doing so, you can determine when the gesture has transitioned from the "began" state to the "changed" state, and run your code only once at that point. Here's an updated version of the handleLongPress:
method to demonstrate this:
-(void)handleLongPress:(UILongPressGestureRecognizer*)recognizer{
if (recognizer.state == UIGestureRecognizerStateBegan) {
NSLog(@"double oo");
// Perform your logic here for the "began" state event
}
}
With this modification, the code within the if
statement will execute only when the gesture recognizer transitions to the "began" state, preventing duplicate execution.
Solution 2: Use Gesture Recognizer Delegate
Another approach is to make use of the UIGestureRecognizerDelegate
protocol to control the execution of the handler method. By implementing the shouldRecognizeSimultaneouslyWithGestureRecognizer:
method and returning NO
for the UILongPressGestureRecognizer
, you can prevent duplicate recognition. Here's an example of how to implement this solution:
@interface YourViewController () <UIGestureRecognizerDelegate>
@end
@implementation YourViewController
- (void)viewDidLoad {
[super viewDidLoad];
UILongPressGestureRecognizer *longPress = [[UILongPressGestureRecognizer alloc]
initWithTarget:self
action:@selector(handleLongPress:)];
longPress.minimumPressDuration = 2.0;
longPress.delegate = self; // Set the delegate to self
[self addGestureRecognizer:longPress];
[longPress release];
}
// Implement UIGestureRecognizerDelegate method
- (BOOL)gestureRecognizer:(UIGestureRecognizer *)gestureRecognizer
shouldRecognizeSimultaneouslyWithGestureRecognizer:(UIGestureRecognizer *)otherGestureRecognizer {
return NO;
}
-(void)handleLongPress:(UILongPressGestureRecognizer*)recognizer{
NSLog(@"double oo");
// Perform your logic here
}
@end
By setting the delegate
property of UILongPressGestureRecognizer
to self
and implementing the shouldRecognizeSimultaneouslyWithGestureRecognizer:
method, we make sure that the handler method is called only once.
It's Time to Get It Right!
And there you have it, folks! Two easy-to-implement solutions to fix the issue of UILongPressGestureRecognizer
getting called twice instead of once: using the gesture recognizer state or leveraging the gesture recognizer delegate. š
Now it's up to you to choose your preferred solution and get your app behaving the way you want it to. Remember, understanding the problem is key to finding the right solution, and hopefully, this blog post has shed some light on the issue and offered you tools to fix it. š”š§
If you have any questions, further issues, or suggestions, please feel free to reach out and engage with us in the comments section below. We'd love to hear from you! šš£ļø
Happy coding, and until next time! šāØ