Is it possible to register a http+domain-based URL Scheme for iPhone apps, like YouTube and Maps?
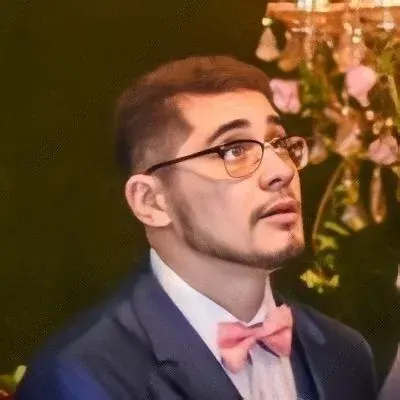
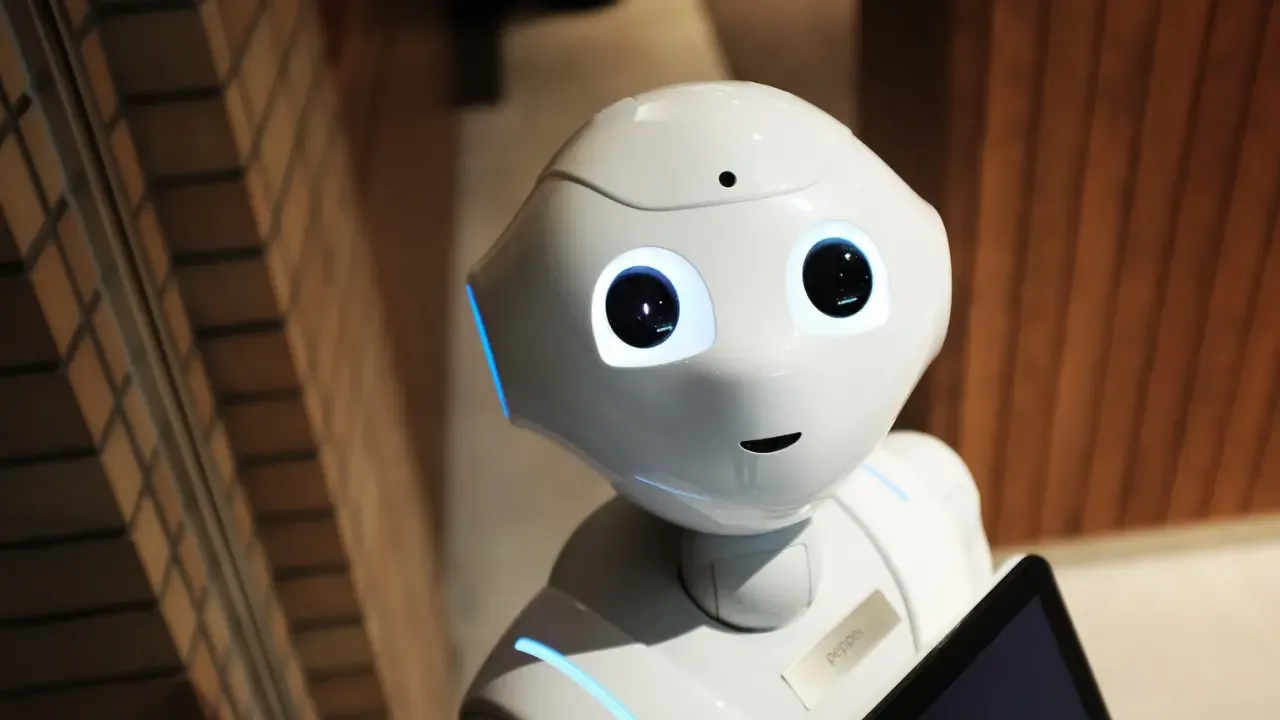
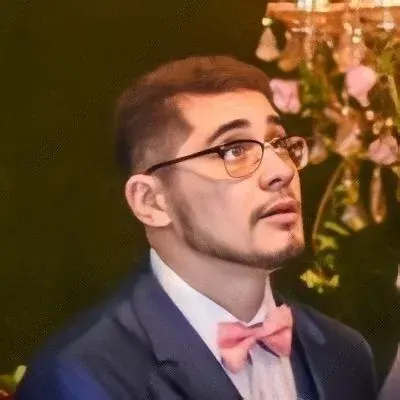
📱 How to Register HTTP+Domain-Based URL Scheme for iPhone Apps like YouTube and Maps
So, you want your iOS app to gracefully handle URLs from your domain like http://martijnthe.nl
, even when your app is not installed. You've heard about registering a unique protocol suffix in the Info.plist file, but you're concerned about the error that Mobile Safari displays when your app is not found. Don't worry! We've got you covered with a clever workaround.
The Problem
When you register a custom URL scheme for your app, Mobile Safari automatically launches your app when a user clicks on a link with that scheme. However, if the app is not installed, Mobile Safari displays an error message instead.
The Solution
Our workaround involves two steps to ensure a smooth user experience:
Use HTTP URLs that work in any desktop browser and render your service in the browser itself.
Check the User-Agent when a request is made from Mobile Safari. If it matches, attempt to open your app using a custom URL scheme. If that fails, redirect the user to the Mobile iTunes page for your app.
Step 1: Using HTTP URLs
By using regular HTTP URLs, you can make your service accessible through any desktop browser. This ensures that users can access your content even if they don't have your app installed. Moreover, it allows search engines to index your web content, making it discoverable and improving your overall visibility.
For example, if your app offers an online store, you can design a website that mimics your app's interface and functionality. This way, users without your app can still browse and purchase products.
Step 2: Handling Requests from Mobile Safari
Now, let's tackle the case where a user accesses your service from Mobile Safari. You can handle this situation by performing the following actions:
Check the
User-Agent
header in the HTTP request to detect if it's coming from Mobile Safari. You can find libraries or code snippets that help with this specific check.If the
User-Agent
matches the Mobile Safari pattern, attempt to open your app using a custom URL scheme. For example, you can try openingmyprotocol://
.If the app is not installed, the custom URL scheme will fail, and the user will be left hanging. To provide a seamless experience, redirect the user to the Mobile iTunes page for your app. You can construct the URL like this:
https://itunes.apple.com/app/[Your-App-Name]/[Your-App-ID]
.
Example Implementation
Here's an example implementation in JavaScript to help you visualize the workaround:
function openAppOrRedirectToStore() {
const userAgent = navigator.userAgent.toLowerCase();
if (/mobile safari/.test(userAgent)) {
// Attempt to open your app using a custom URL scheme
window.location.href = 'myprotocol://';
// If the app is not installed, redirect to the App Store
setTimeout(() => {
window.location.href = 'https://itunes.apple.com/app/[Your-App-Name]/[Your-App-ID]';
}, 2000); // Adjust the delay as needed
}
}
Make sure to adjust the myprotocol://
and iTunes URL placeholders with the appropriate values for your app.
Conclusion
You don't have to worry about Mobile Safari displaying an error when your app is not installed. By following our workaround, you can gracefully handle URLs from your domain, both in your app and on Mobile Safari. Users will be able to access your content seamlessly, whether they have your app installed or not.
So, go ahead and make your app more discoverable and user-friendly by implementing this workaround. Your users will thank you!
Got any more questions? Need further assistance? Let us know in the comments below! 👇