How to load a UIView using a nib file created with Interface Builder
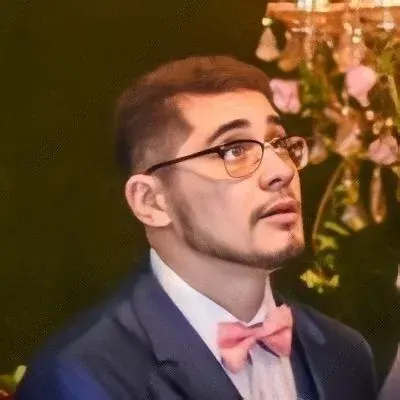
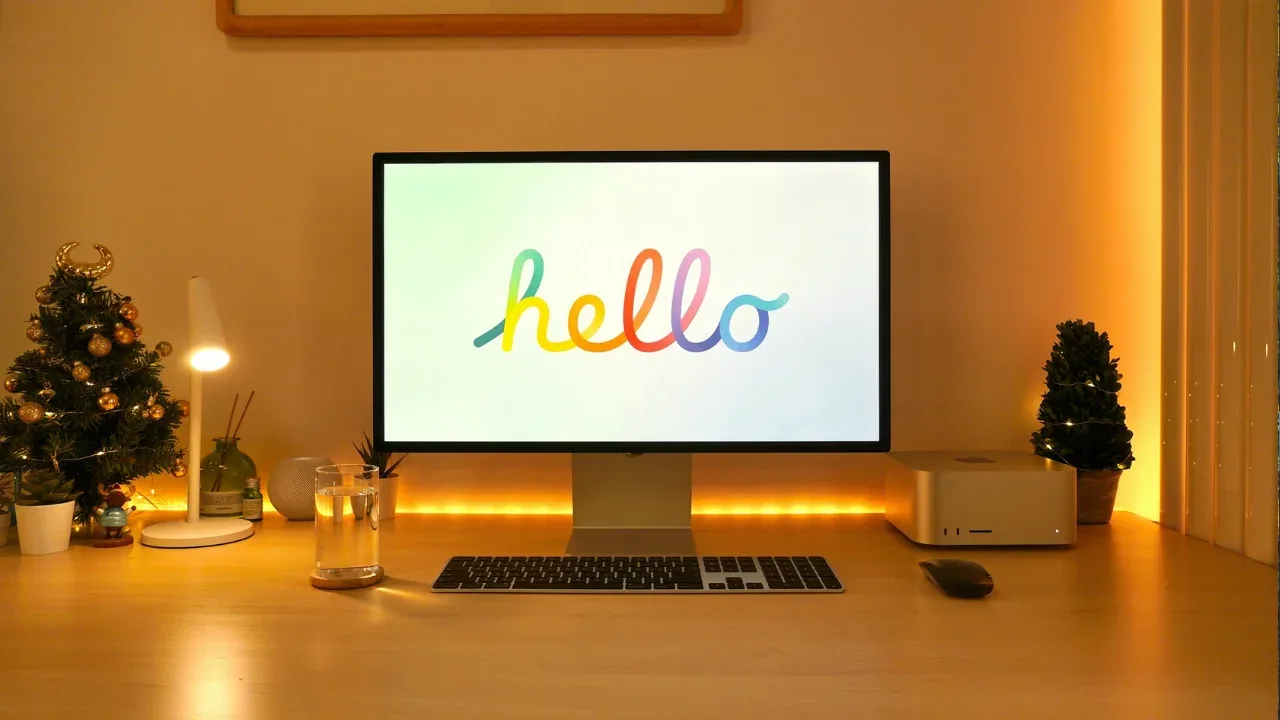
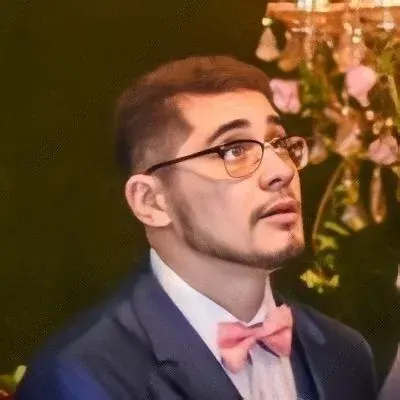
How to Load a UIView Using a Nib File Created with Interface Builder 😎💻
Are you trying to create a "Questionnaire" component for your QuestionManagerViewController
, but running into issues with loading different views? Don't worry, we've got you covered! In this guide, we'll show you how to load a UIView using a nib file created with Interface Builder, and even solve the common error when the view outlet is not set. Let's dive in! 👇
Step 1: Create your UIView subclass 🖌️
To begin, you'll need to create a UIView subclass. Let's call it Question1View
. In this subclass, define any IBOutlets
you need for your UI components, such as labels or buttons.
class Question1View: UIView {
@IBOutlet weak var questionLabel: UILabel!
@IBOutlet weak var answerButton: UIButton!
// Add more outlets as needed
}
Step 2: Create the nib file using Interface Builder 💡
Now, it's time to create the nib file in Interface Builder. Here's what you need to do:
Open Interface Builder and create a new file.
Choose "View" as the template for your file.
Set both the
File's Owner
and the top-level view to be of classQuestion1View
.Customize your UI by adding and arranging components on the view.
Step 3: Connect the outlets in Interface Builder 🔌
To make your UI components work properly, you'll need to connect them to the outlets defined in your Question1View
subclass. Here's how:
Open the nib file in Interface Builder.
Right-click on "File's Owner" in the "Placeholders" section.
Connect the outlets to their corresponding UI components by dragging from the circle next to the outlet name to the UI component.
Step 4: Load the view in your QuestionManagerViewController 🚀
Next, you'll need to load the view in your QuestionManagerViewController
using the nib file. Override the initWithNibName
method like this:
class QuestionManagerViewController: UIViewController {
override init(nibName nibNameOrNil: String?, bundle nibBundleOrNil: Bundle?) {
super.init(nibName: "Question1View", bundle: nil)
// Custom initialization
}
}
Step 5: Resolve the "view outlet not set" error 🛠️
If you encounter the following error: NSInternalInconsistencyException - [UIViewController _loadViewFromNibNamed:bundle:] loaded the "Question1View" nib but the view outlet was not set.
, don't panic! We know how to fix it:
Open the nib file in Interface Builder.
Right-click on the top-level view (your
Question1View
).Connect the
UIView
outlet to the top-level view by dragging from the circle next to the outlet name to the view.
And there you have it! You've successfully loaded a UIView using a nib file created with Interface Builder. 🎉
If you still need any assistance or have any further questions, feel free to reach out to us in the comments below. We're here to help! 😊💬
Your Challenge! 🏆✨
Now that you've learned how to load a UIView using a nib file, why not take it a step further? Try creating multiple views for different questions and dynamically load them in your QuestionManagerViewController
. Share your experience and any cool solutions you come up with in the comments below! We can't wait to see what you create! 👩💻👨💻
Happy coding! 💻🚀
Note: Don't forget to replace the "Question1View" references with the appropriate names for your views and nib files.