How to dismiss keyboard for UITextView with return key?
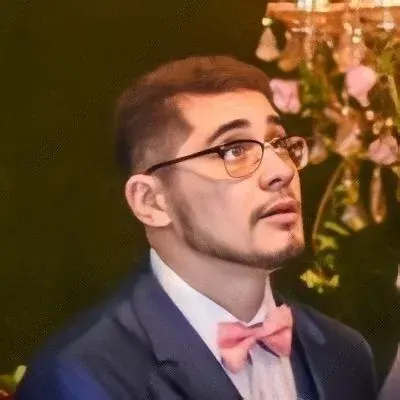
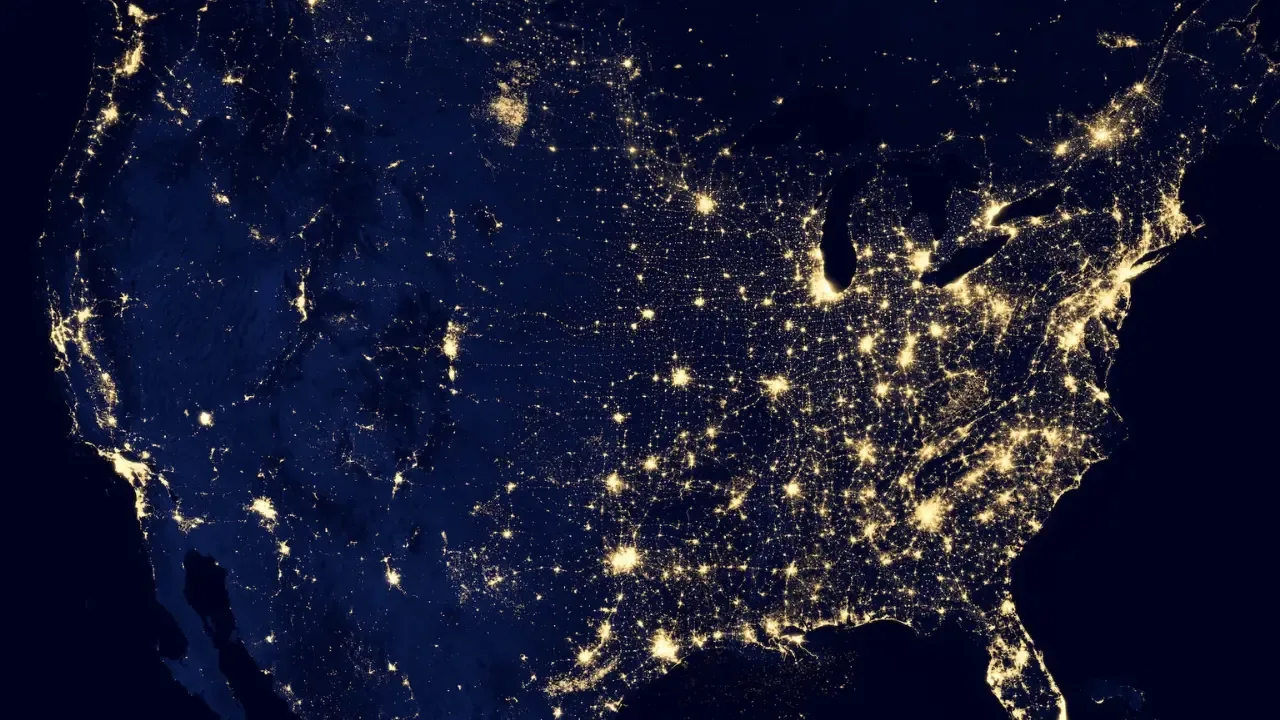
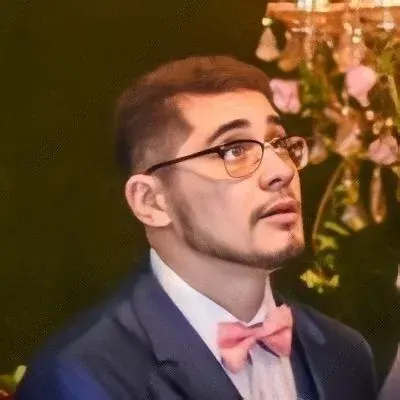
š Title: Easy Ways to Dismiss Keyboard for UITextView using the Return Key
š Hey there! Are you wondering how to dismiss the keyboard for UITextView by simply tapping the return key? š¤ You're not alone! Many developers face this common issue and struggle to find an easy solution. But worry not, because I'm here to guide you through the process and provide you with some handy tips and tricks. Let's get started! š»
š” Understanding the problem
In Interface Builder's library, it is mentioned that pressing the return key on the keyboard should make it disappear for UITextView. But in reality, the return key actually acts as a newline character ('\n') and doesn't dismiss the keyboard as expected. š
š Finding solutions
While resigning first responder using a button and the resignFirstResponder
method is one valid approach, you might be wondering if there's a way to dismiss the keyboard without the need for an additional UIButton. Well, great news! There are a couple of simple alternatives you can try out. Let's explore them:
Using the UITextFieldDelegate method
By conforming to the UITextFieldDelegate
protocol and implementing the method textFieldShouldReturn
, we can listen for the return key press and dismiss the keyboard. However, UITextView doesn't inherit from UITextField, so this approach won't directly work. But don't worry, we have a workaround! š
Subclassing UITextView
One solution is to subclass UITextView and override the canBecomeFirstResponder
property to make it behave like a UITextField. This way, all the UITextFieldDelegate methods will be accessible. Here's how you can do it:
class CustomTextView: UITextView {
override var canBecomeFirstResponder: Bool {
return true
}
}
Now, you can implement textFieldShouldReturn
in your view controller, and when the return key is pressed, the keyboard will automatically dismiss! š
Using NotificationCenter
If subclassing is not a viable option for you, another approach is to listen for the return key press using NotificationCenter and resign the first responder status of the UITextView. Here's how you can do it:
// Add this code in your view controller
override func viewDidLoad() {
super.viewDidLoad()
NotificationCenter.default.addObserver(self, selector: #selector(dismissKeyboard), name: UITextView.textDidEndEditingNotification, object: nil)
}
@objc func dismissKeyboard() {
view.endEditing(true)
}
With this setup, when the return key is pressed on the UITextView, the dismissKeyboard
method will be called, and the keyboard will be dismissed. š
š£ Time to take action!
Now that you know a few handy ways to dismiss the keyboard for UITextView using the return key, it's time to put this knowledge into action and simplify your user interactions. Give these methods a try and see which one works best for your specific needs. And don't forget to share your experience with us in the comments below! Let's engage in a tech-savvy conversation and help each other out! šš
Remember, mastering these small details can make a huge difference in creating polished and user-friendly apps. So keep exploring and learning, my fellow developers! Good luck! ššŖ
āØļøāØ