How to compare two NSDates: Which is more recent?
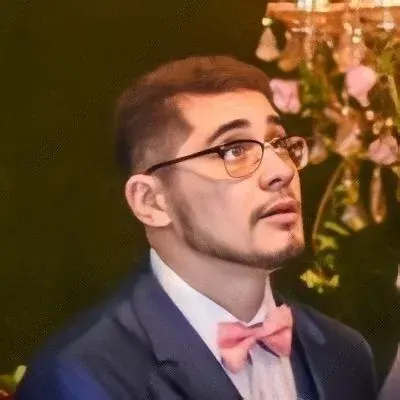
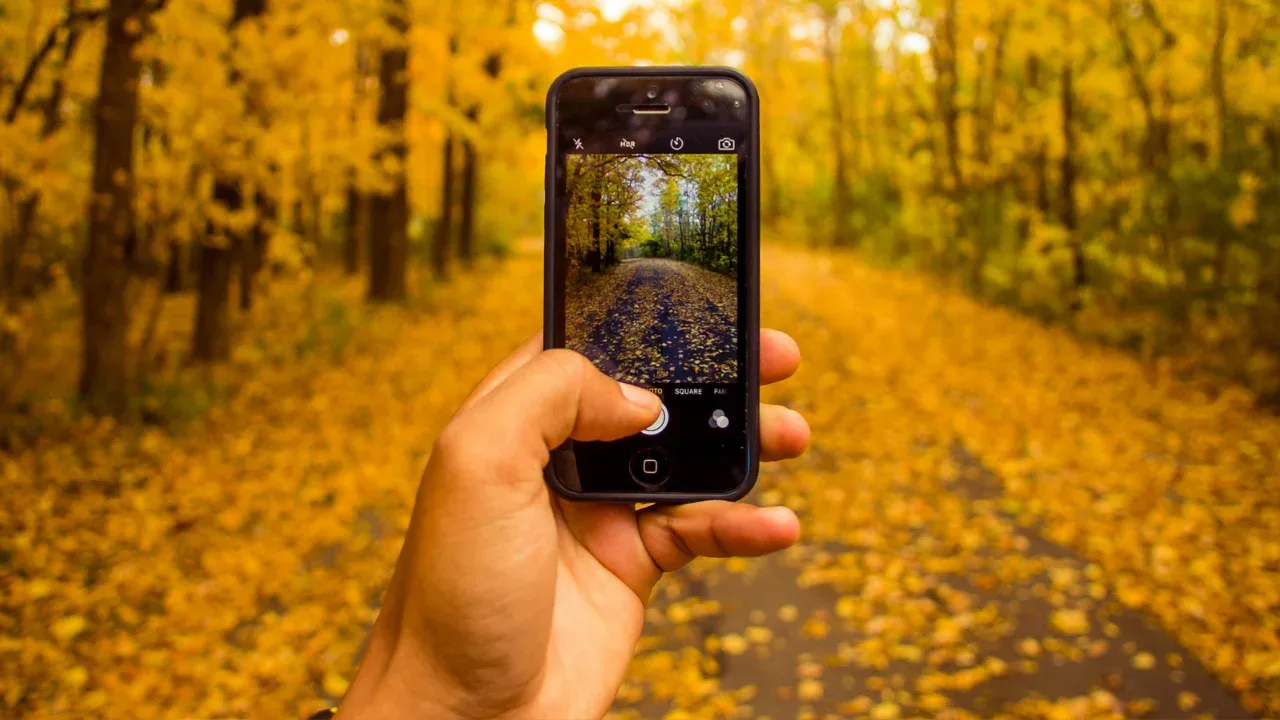
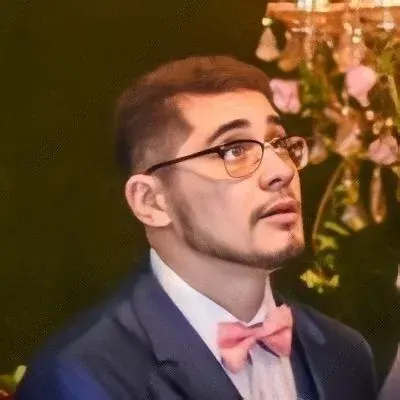
How to compare two NSDates: Which is more recent?
š»š” Welcome back to our tech blog! Today, we're going to address a common issue many developers face when trying to compare two NSDate
objects to determine which one is more recent. This problem came up recently when a reader was attempting to achieve a Dropbox sync between their account and their iPhone.
Here's the code snippet our reader shared with us:
NSLog(@"dB...lastModified: %@", dbObject.lastModifiedDate);
NSLog(@"iP...lastModified: %@", [self getDateOfLocalFile:@"NoteBook.txt"]);
if ([dbObject lastModifiedDate] < [self getDateOfLocalFile:@"NoteBook.txt"]) {
NSLog(@"...db is more up-to-date. Download in progress...");
[self DBdownload:@"NoteBook.txt"];
NSLog(@"Download complete.");
} else {
NSLog(@"...iP is more up-to-date. Upload in progress...");
[self DBupload:@"NoteBook.txt"];
NSLog(@"Upload complete.");
}
Understanding the Issue
Our reader mentioned that they were getting unexpected results when comparing the two dates. Upon inspecting the code, we noticed that they were comparing two NSDate
strings using the <
and >
operators. This is incorrect because NSDate
objects are not comparable using these operators.
The Solution
To correctly compare two NSDate
objects and determine which one is more recent, we need to use the compare:
method. Let's update the code:
NSLog(@"dB...lastModified: %@", dbObject.lastModifiedDate);
NSLog(@"iP...lastModified: %@", [self getDateOfLocalFile:@"NoteBook.txt"]);
NSComparisonResult result = [[dbObject lastModifiedDate] compare:[self getDateOfLocalFile:@"NoteBook.txt"]];
if (result == NSOrderedAscending) {
NSLog(@"...db is more up-to-date. Download in progress...");
[self DBdownload:@"NoteBook.txt"];
NSLog(@"Download complete.");
} else if (result == NSOrderedDescending) {
NSLog(@"...iP is more up-to-date. Upload in progress...");
[self DBupload:@"NoteBook.txt"];
NSLog(@"Upload complete.");
} else {
NSLog(@"...both dates are the same.");
}
In this updated code, we use the compare:
method to compare the two NSDate
objects, and we store the result in result
. Then, we use an if-else
statement to handle the different comparison scenarios.
Understanding the Output
Let's take a look at the random output our reader shared:
2011-05-11 14:20:54.413 NotePage[6918:207] dB...lastModified: 2011-05-11 13:18:25 +0000
2011-05-11 14:20:54.414 NotePage[6918:207] iP...lastModified: 2011-05-11 13:20:48 +0000
2011-05-11 14:20:54.415 NotePage[6918:207] ...db is more up-to-date.
In this case, the output is incorrect because 2011-05-11 13:18:25 +0000
is actually less recent than 2011-05-11 13:20:48 +0000
. This is because the comparison was being performed incorrectly earlier in the code.
On the other hand, the correct output would be:
2011-05-11 14:20:25.097 NotePage[6903:207] dB...lastModified: 2011-05-11 13:18:25 +0000
2011-05-11 14:20:25.098 NotePage[6903:207] iP...lastModified: 2011-05-11 13:19:45 +0000
2011-05-11 14:20:25.099 NotePage[6903:207] ...iP is more up-to-date.
Here, the code correctly identifies that the iPhone's date is more recent than the Dropbox date.
Conclusion
Comparing two NSDate
objects can be tricky, but by following the correct approach, we can easily determine which date is more recent. Remember to use the compare:
method and handle the different comparison scenarios.
š” Now that you understand how to compare two NSDate
objects, why not try implementing it in your own project? Share your experience with us in the comments below! Happy coding! š©āš»š
šš For more helpful tech guides and tutorials, be sure to subscribe to our blog and follow us on social media. Visit our website for more exciting content!