How can we programmatically detect which iOS version is device running on?
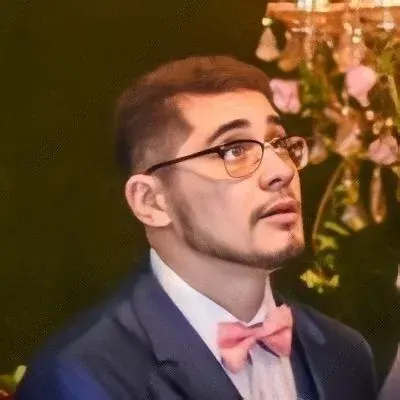
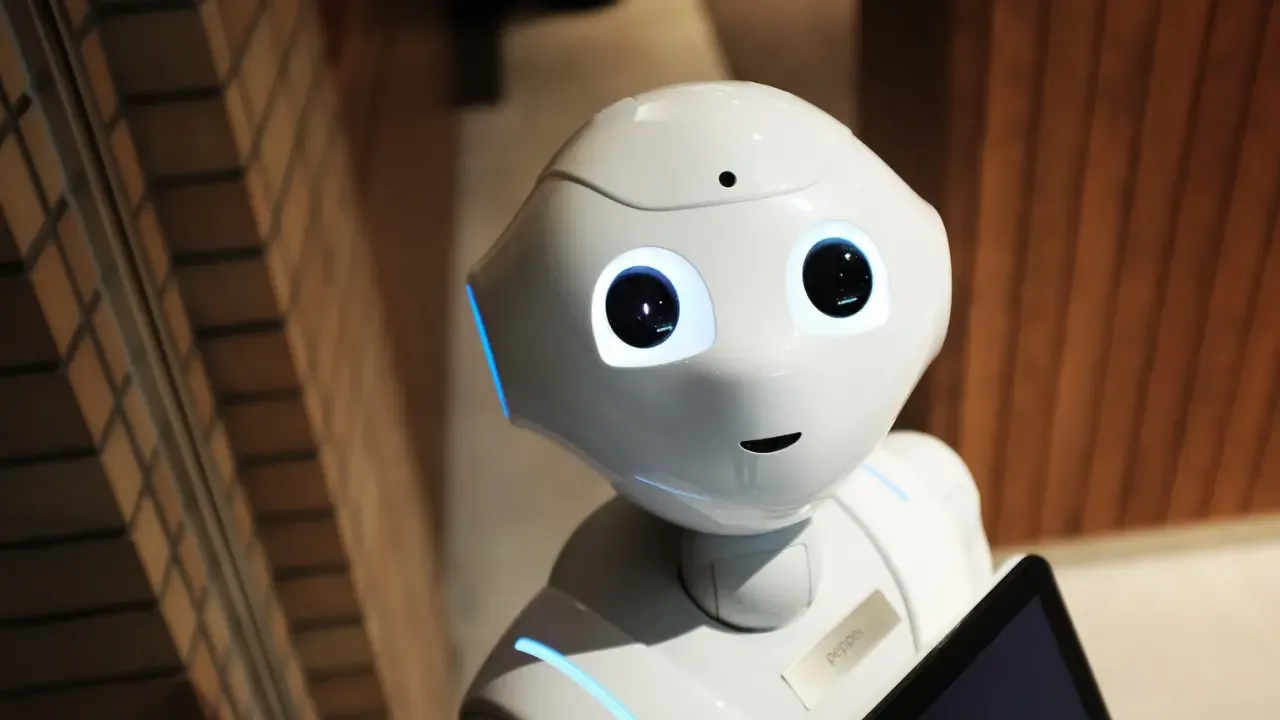
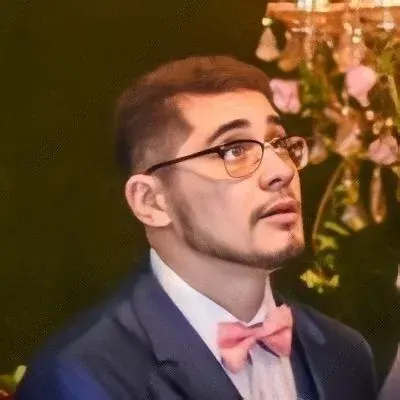
📱 How to Programmatically Detect the iOS Version on a Device?
Are you a developer struggling to detect which iOS version a user's device is running? 🤔 Don't worry, we've got you covered! In this blog post, we will explore the common issue of programmatically detecting the iOS version and provide you with easy solutions to tackle this problem. 💪
The Problem: Detecting iOS Versions
Imagine you want to display a label in your app, but only for users with iOS versions older than 5.0. How can you accurately determine the iOS version running on a user's device? 📲 It's time to find out!
Easy Solution: Using the UIDevice Class
To obtain the iOS version programmatically, we can leverage the power of the UIDevice
class provided by Apple. 🙌 This class gives us access to a variety of device-related information, including the iOS version.
Here's an example snippet of code that demonstrates how to get the iOS version:
let systemVersion = UIDevice.current.systemVersion
By calling UIDevice.current.systemVersion
, you will obtain a String
representing the iOS version running on the device. 🎉
Putting It into Action: Comparing iOS Versions
Now that you have the iOS version in your hands, you can compare it and execute different actions based on the result. Let's assume you want to display a label for users with iOS versions older than 5.0.
if let systemVersion = Double(UIDevice.current.systemVersion), systemVersion < 5.0 {
yourLabel.isHidden = false
}
In this example, we first convert the obtained system version (String
) to a Double
value to enable straightforward comparison. After that, you can use a simple if
statement to determine whether the label should be displayed or not. 😎
Take It Further: Handling Edge Cases
It's essential to consider potential edge cases when working with iOS versions. For example, what if UIDevice.current.systemVersion
is nil? To handle such cases gracefully, you can incorporate optional unwrapping or provide a fallback value:
guard let systemVersion = UIDevice.current.systemVersion else {
// Handle the case when system version is nil
return
}
if let version = Double(systemVersion), version < 5.0 {
yourLabel.isHidden = false
} else {
yourLabel.isHidden = true
}
With these additional considerations, you ensure that your code remains robust and resilient, even in unexpected scenarios. 💪
🚀 Time for Action!
You are now armed with the knowledge to programmatically detect the iOS version on any user's device. Go ahead and implement this solution in your app, and make sure to test it thoroughly to ensure everything works as expected. 🏋️♀️
If you have any questions or face any issues, don't hesitate to leave a comment or reach out to our friendly developer community. We're always here to help you out! 😊
Have you ever struggled with programmatically detecting iOS versions on a device? How did you solve it? Share your experience with us in the comments below! 💬👇
Remember, technology is ever-evolving, and we must stay updated. Hit that share button and pass on this knowledge to fellow developers who might find it handy! 📤📢 Together, we can build a stronger, more efficient developer community.
Happy coding! 💻🎉