Gradients on UIView and UILabels On iPhone
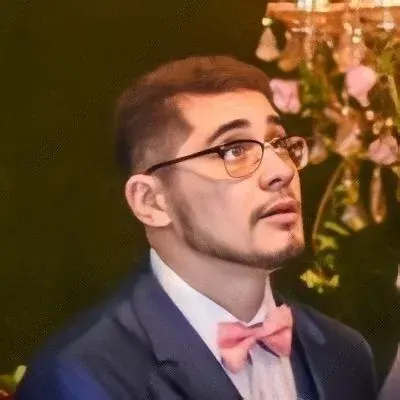
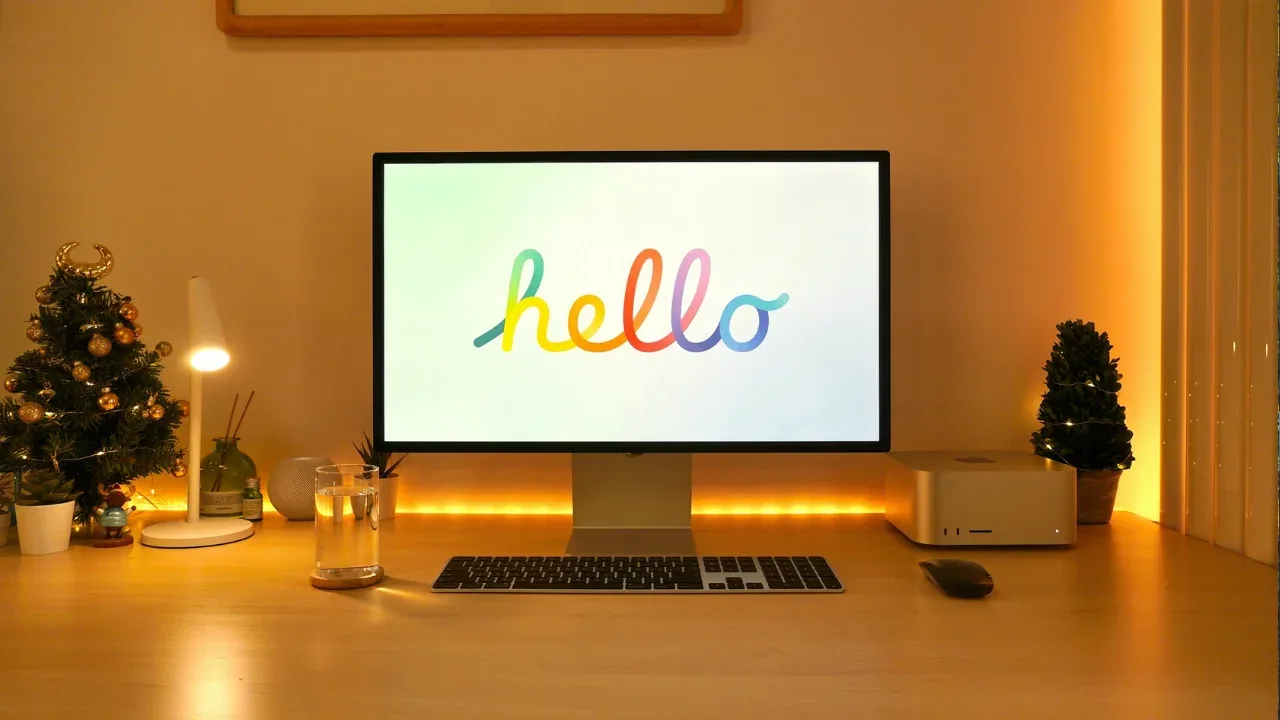
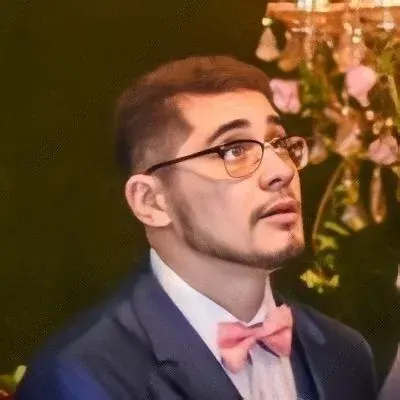
🌈📱 How to Create Gradients on UIView and UILabels on iPhone 🌈📱
Have you ever wanted to spice up your iPhone app design by using gradients as the background for your UIView or UILabel? 😍🔤 But wait, you're wondering how you can achieve this without hardcoding the colors or relying on static images? 🤔 Does this sound like a problem you've been struggling with? Fear not, because I'm here to show you the quickest and easiest way to tackle this issue! 🙌💪
Before we dive into the solutions, let's first address the common problem mentioned in the question. 👥 The issue at hand is that the background for the UIView or UILabel needs to be a gradient, which cannot be achieved using a regular UIColor. Additionally, using a graphics program to create the desired gradient look is not feasible, as the text may vary depending on data returned from a server. 🖥️💯
So, what can you do to create gradients on UIView and UILabels programmatically? 🤔 Here are two easy solutions:
1️⃣ Using CAGradientLayer:
The first solution involves using CAGradientLayer, which is a Core Animation class provided by iOS. This class allows us to create gradients with ease. Here's an example of how to apply a gradient to a UIView:
import UIKit
let gradientLayer = CAGradientLayer()
gradientLayer.frame = yourView.bounds
gradientLayer.colors = [UIColor.red.cgColor, UIColor.blue.cgColor]
yourView.layer.insertSublayer(gradientLayer, at: 0)
You can customize the colors array to include as many colors as you want, and even specify the locations of each color within the gradient. For UILabels, you can wrap the label inside a UIView and apply the gradient to that UIView instead. 🎨🌈
2️⃣ Using NSAttributedString:
If you want to apply a gradient to a UILabel directly, without using an extra UIView, you can leverage NSAttributedString. Here's an example of how to achieve this:
import UIKit
let gradientText = "Your Text"
let gradient = CAGradientLayer()
gradient.frame = yourLabel.bounds
gradient.colors = [UIColor.red.cgColor, UIColor.blue.cgColor]
gradient.startPoint = CGPoint(x: 0, y: 0)
gradient.endPoint = CGPoint(x: 1, y: 0)
let labelText = NSMutableAttributedString(string: gradientText)
labelText.addAttribute(.foregroundColor, value: gradient, range: NSRange(location: 0, length: gradientText.count))
yourLabel.attributedText = labelText
In this solution, we create a CAGradientLayer and set it as the foreground color for the UILabel using an NSAttributedString. Adjust the start and end points of the gradient to achieve the desired effect. 🎨✨
Now that you have the solutions, it's time for you to implement gradients into your app! 🚀💻 Let your creativity flow and experiment with different colors, locations, and directions to make your app truly unique.
If you have any other questions or want to share your results, feel free to leave a comment below. We love hearing from our readers! ❤️🗨️
So, what are you waiting for? Get ready to take your app's design to the next level with stunning gradients! 🎉🌈