Finding the direction of scrolling in a UIScrollView?
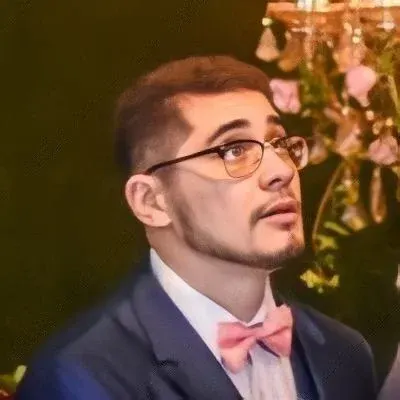
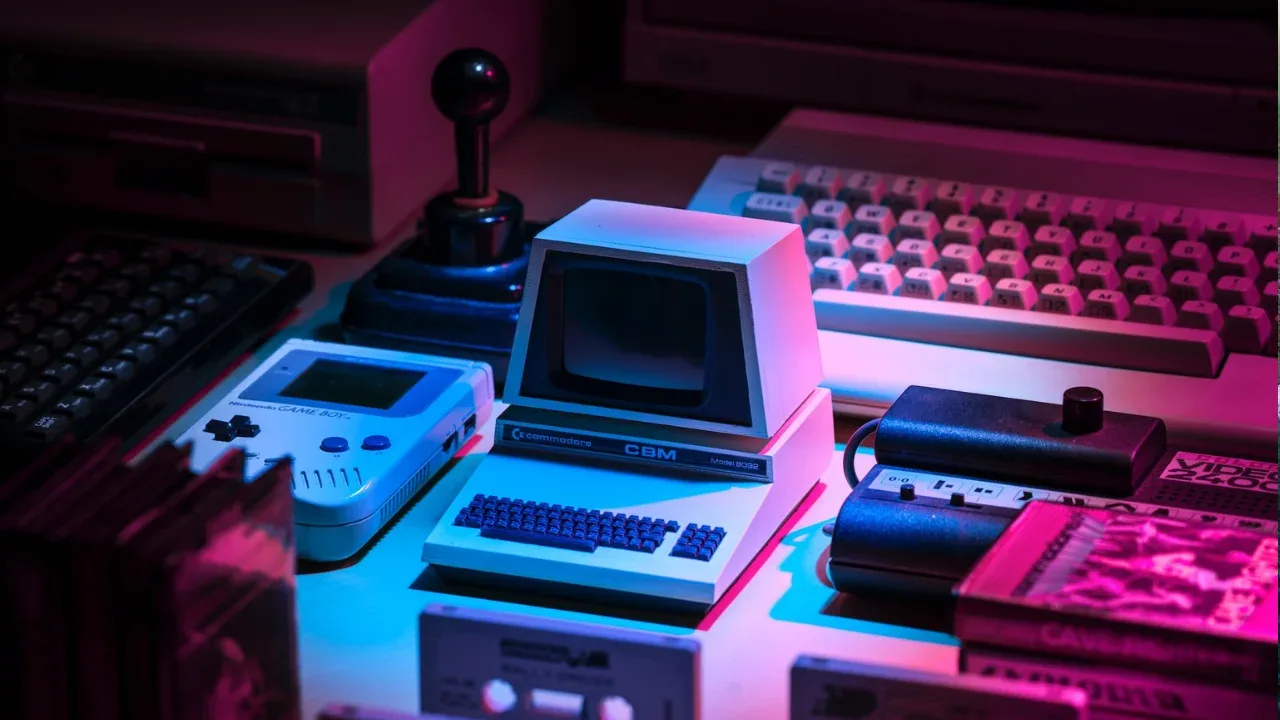
๐๐ฅ๐ Hey tech enthusiasts! Are you experiencing issues with finding the direction of scrolling in a UIScrollView
? ๐ Don't worry, we've got you covered! In this blog post, we'll address common problems and provide easy solutions to help you overcome this challenge. Let's dive in! ๐ช
๐ค The context for this question is having a UIScrollView
with only horizontal scrolling allowed, and the goal is to determine the direction (left or right) of the user's scroll. The original approach involved subclassing UIScrollView
and overriding the touchesMoved
method. However, there seems to be an issue where this method is not always being called when moving. So, what could be causing this problem? ๐ง
๐ One possible explanation could be that the touch events are being intercepted or overridden by other elements in the view hierarchy. To ensure that our overridden touchesMoved
method is called, we can try a different approach using gesture recognizers provided by UIKit. ๐
Here's how we can modify our code to use a UIPanGestureRecognizer
instead:
UIPanGestureRecognizer *panGesture = [[UIPanGestureRecognizer alloc] initWithTarget:self action:@selector(handlePan:)];
[self.scrollView addGestureRecognizer:panGesture];
Implement the handlePan:
method to determine the scroll direction based on the gesture's translation in the x-axis:
- (void)handlePan:(UIPanGestureRecognizer *)gestureRecognizer
{
CGPoint translation = [gestureRecognizer translationInView:self.scrollView];
if (translation.x > 0) {
NSLog(@"RIGHT");
} else if (translation.x < 0) {
NSLog(@"LEFT");
}
}
๐ก By using a gesture recognizer, we can ensure that our method will be called even if the UIScrollView
doesn't receive the touch events directly. This provides a more reliable solution for tracking the scroll direction and avoids potential conflicts with other components in the view hierarchy. ๐
But what if you want to handle the scrolling direction dynamically as the user scrolls, rather than just logging debug statements? ๐ค No worries! Here's an example of how you can achieve just that:
- (void)handlePan:(UIPanGestureRecognizer *)gestureRecognizer
{
CGPoint translation = [gestureRecognizer translationInView:self.scrollView];
if (gestureRecognizer.state == UIGestureRecognizerStateChanged) {
if (translation.x > 0) {
NSLog(@"RIGHT");
// Handle right scroll
} else if (translation.x < 0) {
NSLog(@"LEFT");
// Handle left scroll
}
}
}
Now that we've provided you with alternative solutions, it's time for you to try them out and see which one works best for your specific use case! ๐งช Remember to adapt the code snippets to your programming language of choice.
๐๐ So there you have it โ easy solutions to find the direction of scrolling in a UIScrollView
! But we don't want to stop here; we want to hear from you! ๐ Share your experiences, thoughts, or perhaps even a different solution to this problem in the comments section below. Let's help each other grow and overcome these challenges together! ๐ชโจ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
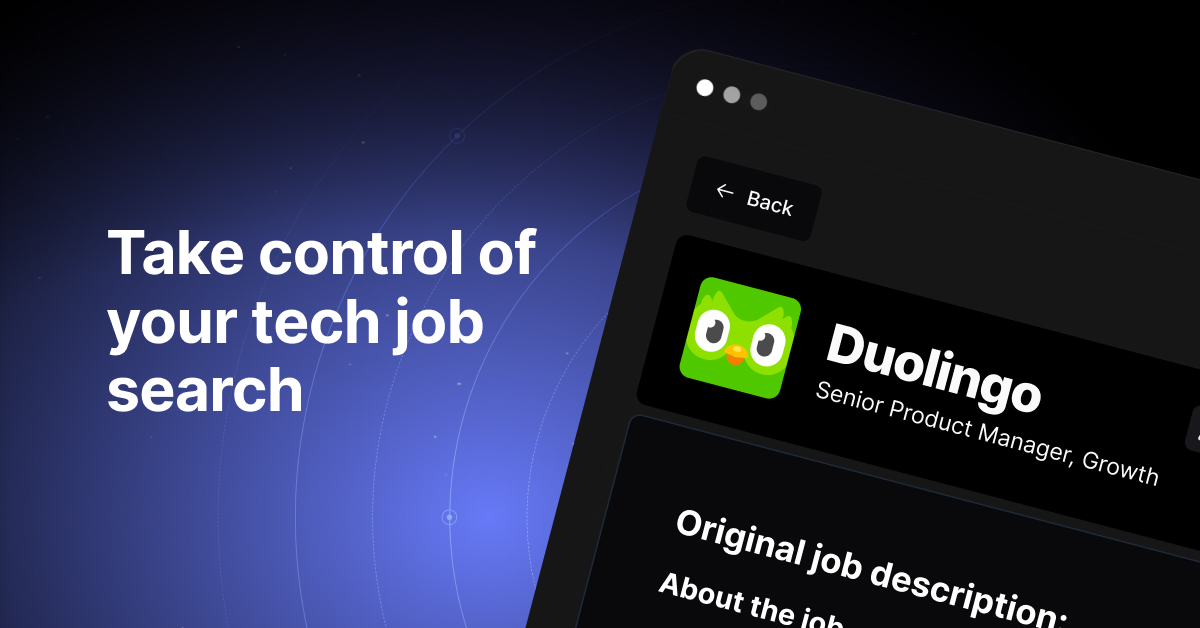