Xcode 8 / Swift 3: "Expression of type UIViewController? is unused" warning
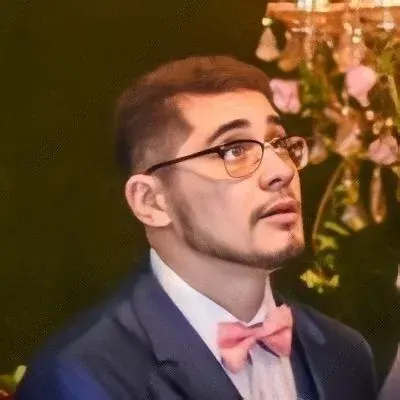
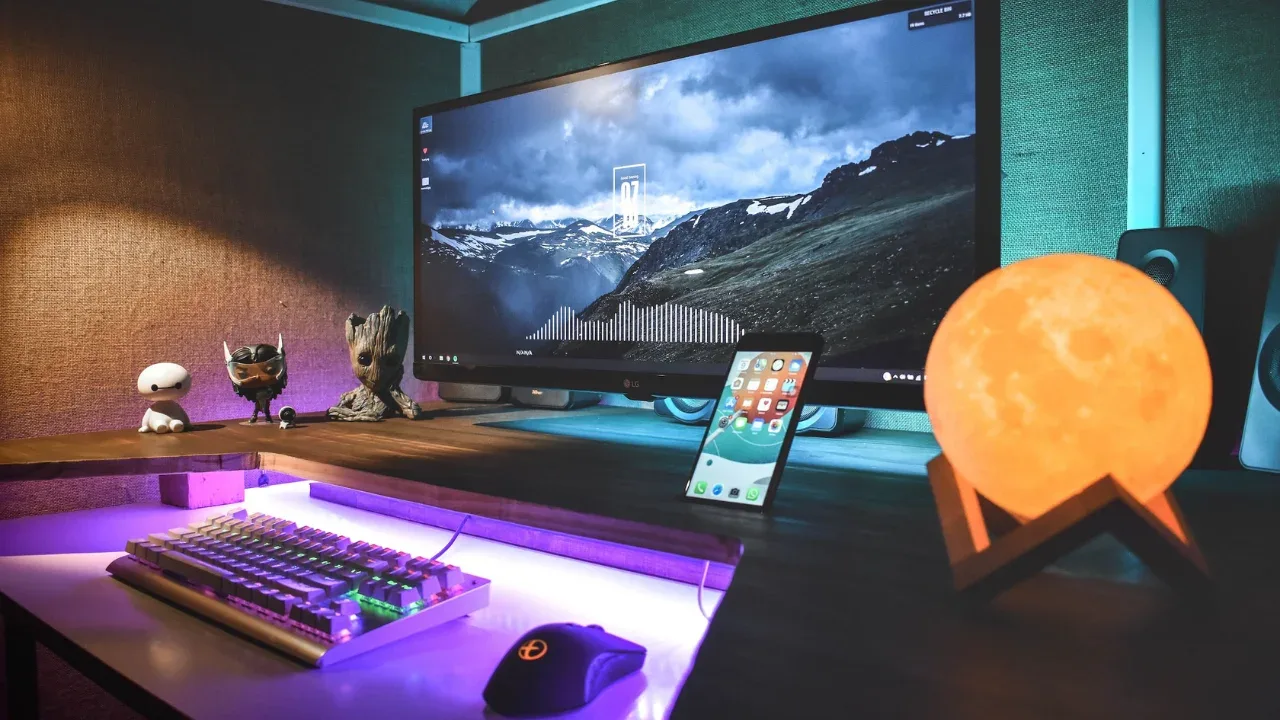
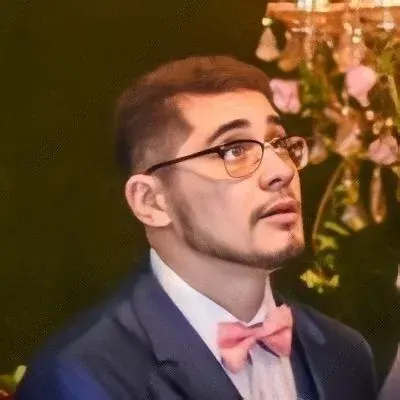
Fixing the "Expression of type UIViewController? is unused" warning in Xcode 8 / Swift 3. ๐ฑ๐ซ
So you've upgraded to Xcode 8 and suddenly you start seeing this warning: "Expression of type UIViewController?
is unused." ๐ณ But everything seems to be working fine, so what's the deal? Let's dive in and understand what's happening here. ๐ก
Understanding the warning โ ๏ธ
The warning is appearing because in Swift 3, optional values are not automatically unwrapped when used. In your code snippet, the navigationController
property is of type UINavigationController?
, meaning it's an optional value. Since you are not using the value directly, Xcode considers it as unused and warns you about it. ๐ข
Solving the issue ๐
There are a couple of ways to fix this warning, depending on whether you want to actually use the value or not. Let's explore both scenarios. ๐
Scenario 1: Using the value โ
If you actually want to use the navigationController
value, you can safely eliminate the warning by explicitly unwrapping it using optional chaining. Simply add the exclamation mark (!
) after the property, like this:
func exitViewController()
{
navigationController!.popViewController(animated: true)
}
By doing this, you are telling Xcode that you are aware the value might be nil
, but you want to force unwrap it and use it. This will remove the warning and make your code compile cleanly again. ๐
Scenario 2: Not using the value ๐ โโ๏ธ
If you don't actually need to use the navigationController
value and you want to get rid of the warning without forcing the unwrap, you can use an underscore (_
) to explicitly ignore the value. Here's how your code would look like:
func exitViewController()
{
_ = navigationController?.popViewController(animated: true)
}
By using the underscore, you are indicating to Xcode that you are intentionally ignoring the value, and therefore the warning should be disregarded. This allows you to keep the code clean and avoid any unnecessary force unwrapping. ๐งน
Conclusion and call-to-action ๐
Now that you understand the "Expression of type UIViewController? is unused" warning and how to fix it, go ahead and apply the appropriate solution to your code. Whether you choose to force unwrap the value or explicitly ignore it, make sure you're doing it consciously to avoid any potential crashes or bugs in your app. Happy coding! ๐ปโจ
Did you find this blog post helpful? Let me know in the comments below! And don't forget to share this post with your fellow iOS developers who might be facing the same warning. Together, we can make the coding world a better place! ๐๐ค