Using isKindOfClass with Swift
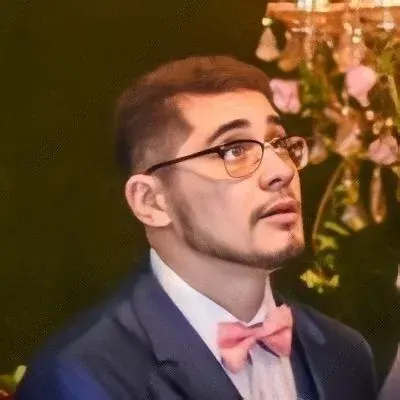
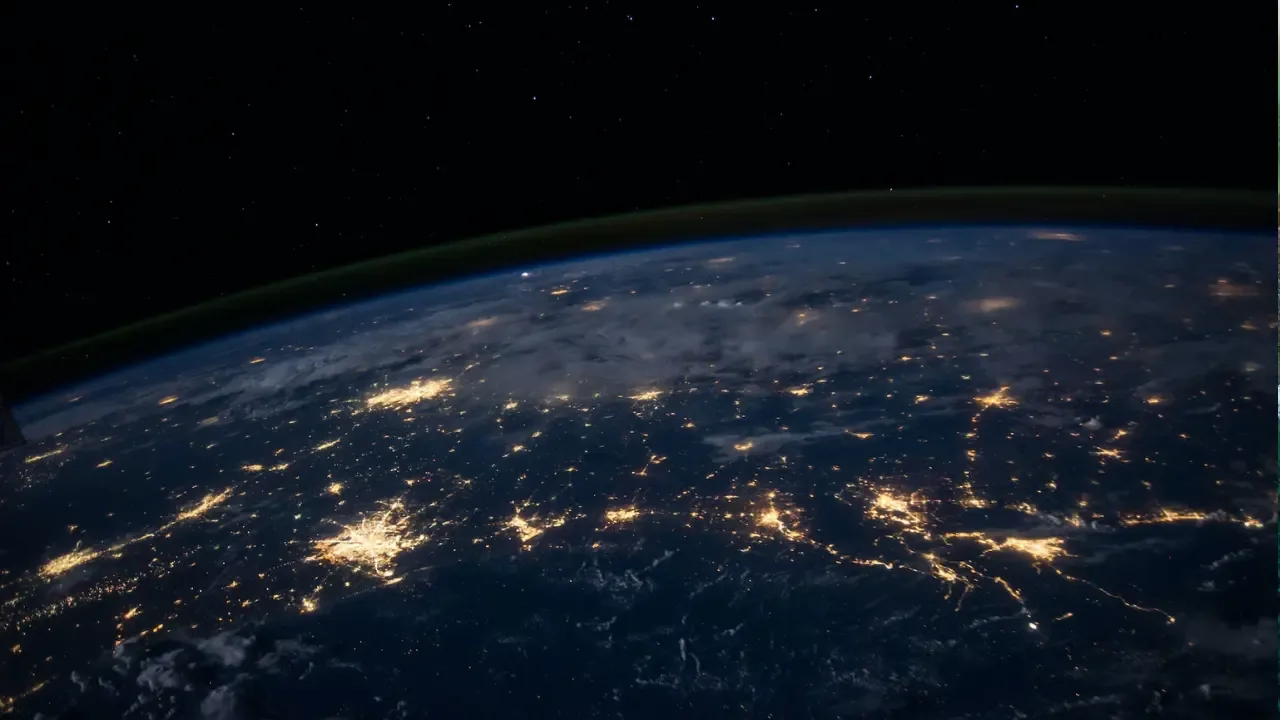
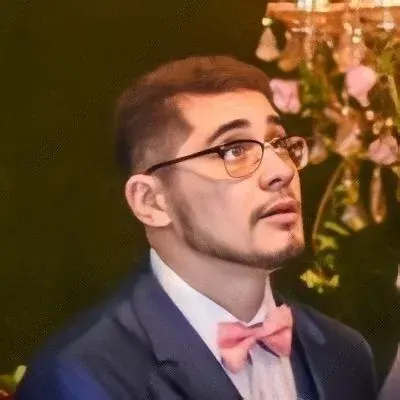
Using the isKindOfClass
Method with Swift 💻🤔
So, you're trying to convert some Objective-C code into Swift, but you're stuck on how to use the isKindOfClass
method in the new syntax. Don't worry, I've got you covered! In this blog post, I'll walk you through how to use isKindOfClass
with Swift, and provide easy solutions to any common issues you may encounter. Let's dive in! 🏊♂️
Understanding the Objective-C Code 📚
Before we jump into the Swift syntax, let's take a closer look at the Objective-C code snippet provided. This code is implementing the touchesBegan
method, which is triggered when a user touches the screen.
In the Objective-C code, the isKindOfClass
method is being used to check whether the touched view is of type UIPickerView
. If it is, some specific actions are performed.
Converting the Code to Swift 🔀
Now, let's convert this code snippet to Swift. The first step is to override the touchesBegan
method in your Swift class. Here's how you do it:
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
super.touchesBegan(touches, with: event)
// Your code goes here...
}
In Swift, the method signature has changed slightly. The NSSet
parameter is now of type Set<UITouch>
, and the UIEvent
parameter is optional (UIEvent?
).
Using isKind(of:)
with Swift ❤️🔬
To mimic the functionality of isKindOfClass
, we'll use the isKind(of:)
method in Swift. Here's how you can check if the touched view is of type UIPickerView
:
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
super.touchesBegan(touches, with: event)
let touch = touches.first
if touch?.view is UIPickerView {
// Your touch was in a UIPickerView... do whatever you have to do...
}
}
In Swift, we no longer need to use the class
keyword. Instead, we can simply use the is
keyword to check if the touched view is of the desired type. We'll use optional chaining (?
) to safely unwrap the touch
object and access its view
property.
Handling Optional Values 🧩❓
In the Swift code snippet above, you may have noticed the use of optional values. By using optional chaining and the ?
operator, we can handle cases where the touch
object or its view
property is nil
.
If the touched view is not of type UIPickerView
, the if
condition evaluates to false
, and the code inside the block will not be executed. You can add your specific actions within this block.
Common Issues and Troubleshooting 🛠️❗
Here are a few common issues you may encounter when using isKind(of:)
(or is
) in Swift, along with their solutions:
No access to the touched view: Make sure you have a reference to the touched view. In the code snippet above, we retrieve the first touch from the
touches
set using thefirst
property.View hierarchy issues: Double-check that the view you're testing with
isKind(of:)
is actually a subview within the view hierarchy. If it's not, the condition will always evaluate tofalse
.Subclassing issues: If you're working with custom subclasses, make sure that you're checking against the correct class. The
isKind(of:)
method checks for the specified class or any of its subclasses.
Conclusion and Call-to-Action 🎉📣
Congratulations! You now know how to use the isKind(of:)
method in Swift to check the type of a view. I hope this guide has helped you understand the conversion process and overcome any common issues you may have encountered.
If you found this blog post helpful, why not share it with your friends or colleagues who are also learning Swift? And if you have any further questions or need assistance, feel free to leave a comment below. Happy coding! 🚀💻