UIView Infinite 360 degree rotation animation?
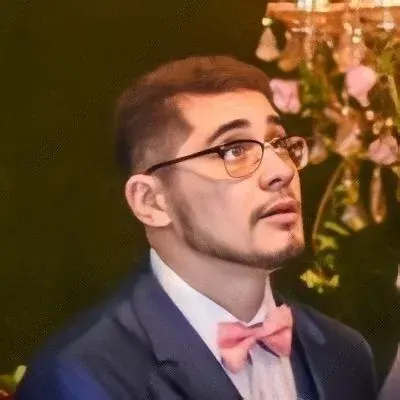
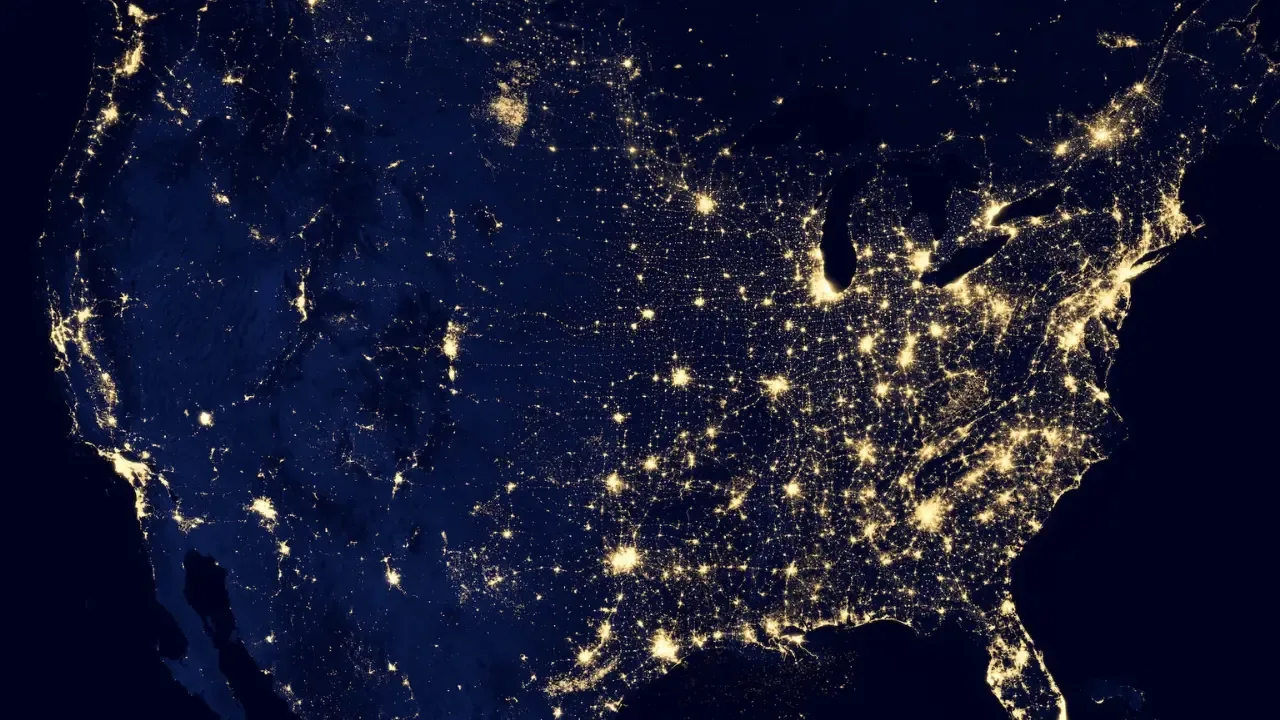
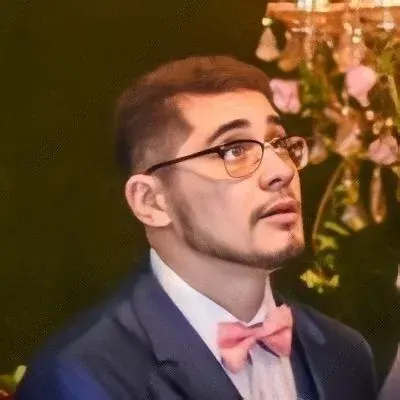
UIView Infinite 360 Degree Rotation Animation Made Easy! 😎
So you want to create an awesome 360-degree rotation animation for a UIImageView
, huh? You've tried several tutorials online, but none of them seem to work as expected. You're either facing issues where the rotation stops or jumps to a new position. Frustrating, right? But don't worry, I've got you covered! In this guide, I'll walk you through some common issues and provide easy solutions to achieve that seamless infinite rotation effect. Let's dive in! 🚀
The Annoying Behavior 🔄
Before we get into the solutions, let's understand the problem a bit better. You mentioned that you tried using the animateWithDuration:delay:options:animations:completion:
method, and it almost worked for you. However, there are a couple of hiccups you encountered:
When using
M_PI
(180 degrees), the rotation works fine. But if you call the method again, it rotates backward instead of continuing from the current position.If you use
2 * M_PI
(360 degrees), the view doesn't move at all since it's the same position.
Let's unravel these issues and give our animated rotation the seamless 360-degree spin it deserves! 💫
Solution #1: Using Keyframe Animations 🔄
One way to achieve the desired infinite rotation effect is to use keyframe animations. This approach allows us to create a loop by specifying multiple keyframes for the animation. Here's an example code snippet to get you started:
// Swift
UIView.animateKeyframes(withDuration: 1.0, delay: 0.0, options: [.repeat, .calculationModeCubic], animations: {
UIView.addKeyframe(withRelativeStartTime: 0.0, relativeDuration: 0.5, animations: {
imageToMove.transform = CGAffineTransform(rotationAngle: CGFloat.pi)
})
UIView.addKeyframe(withRelativeStartTime: 0.5, relativeDuration: 0.5, animations: {
imageToMove.transform = CGAffineTransform(rotationAngle: CGFloat.pi * 2)
})
}, completion: { _ in
// Animation completion block
})
// Objective-C
[UIView animateKeyframesWithDuration:1.0 delay:0.0 options:UIViewKeyframeAnimationOptionRepeat | UIViewKeyframeAnimationOptionCalculationModeCubic animations:^{
[UIView addKeyframeWithRelativeStartTime:0.0 relativeDuration:0.5 animations:^{
imageToMove.transform = CGAffineTransformMakeRotation(M_PI);
}];
[UIView addKeyframeWithRelativeStartTime:0.5 relativeDuration:0.5 animations:^{
imageToMove.transform = CGAffineTransformMakeRotation(2 * M_PI);
}];
} completion:^(BOOL finished) {
// Animation completion block
}];
In this example, we use keyframes with relative start times and durations. We rotate the view 180 degrees (CGFloat.pi
) in the first half of the animation and then rotate it another 180 degrees (CGFloat.pi * 2
) in the second half. By setting the animation options to .repeat
, the animation loops infinitely, giving us the desired 360-degree rotation effect. 🔄
Solution #2: Transform = Rotation + Previous Transform 🔄
Another approach to achieving an endless rotation is by keeping track of the previous rotation angle and adding it to the new angle. Here's how:
// Swift
UIView.animate(withDuration: 1.0, delay: 0.0, options: [.repeat, .curveLinear], animations: {
imageToMove.transform = imageToMove.transform.rotated(by: CGFloat.pi)
}, completion: { _ in
// Animation completion block
})
// Objective-C
[UIView animateWithDuration:1.0 delay:0.0 options:UIViewAnimationOptionRepeat | UIViewAnimationOptionCurveLinear animations:^{
imageToMove.transform = CGAffineTransformRotate(imageToMove.transform, M_PI);
} completion:^(BOOL finished) {
// Animation completion block
}];
In this solution, we use the CGAffineTransformRotate
function to add the previous rotation angle (CGFloat.pi
) to the existing transform of the image view. By setting the animation options to .repeat
, the rotation continues infinitely without any jump or pause. Voila! 🎉
Bonus Tip: Smooth Animation 🌈
If you want to make the rotation animation smoother, you can experiment with the animation curve options. In the above examples, we used .curveLinear
, which gives us a constant rotation speed. However, you can try out other animation curves like .curveEaseIn
or .curveEaseInOut
to add some flair to your rotation effect. Get creative! 🌟
Your Turn to Shine ✨
Congratulations! You now have two awesome solutions to create an infinite 360-degree rotation animation for a UIImageView
in your iOS app. Time to put your newfound knowledge into action and make your app shine! ✨
Share your thoughts, experiences, or any other creative ideas you have to enhance this rotation animation. Did you face any challenges while implementing it? Let's discuss in the comments below and keep the conversation going! 💬
Happy coding! 👩💻👨💻
Note: Make sure to import the necessary frameworks (UIKit for Swift, and UIKit and Foundation for Objective-C) and adjust the code as per your app's requirements.