UIButton remove all target-actions
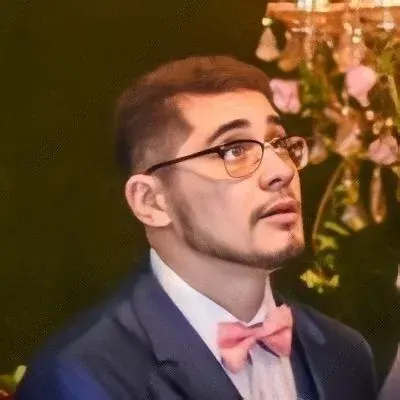
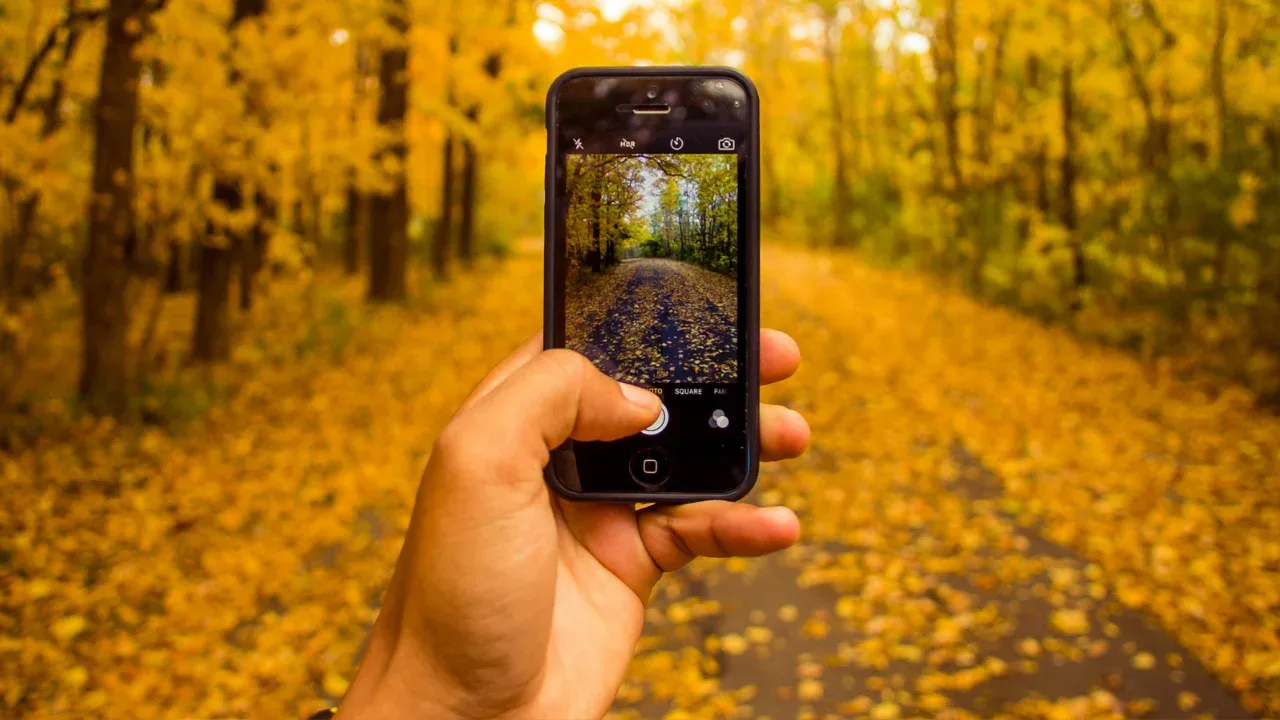
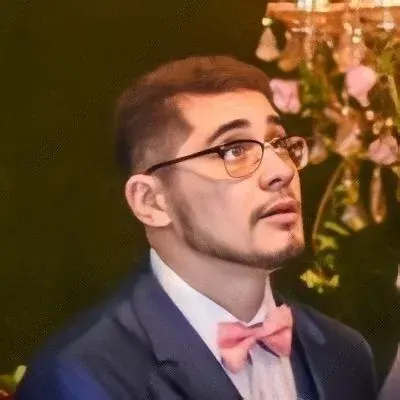
How to Remove All Target Actions from a UIButton
Are you feeling overwhelmed by the multiple target actions you added to your UIButton? Don't worry, we've got you covered! In this guide, we'll show you how to remove all target actions from a UIButton without deallocating anything, so you can set new targets with ease. ๐ช๐ฅ
The Problem ๐ค
Imagine you have a UIButton and have added multiple target-action pairs for different control events. However, you now want to clean the slate and start fresh by removing all these target actions. The question is, how can this be done efficiently and without causing any memory-related issues? ๐คทโโ๏ธ
The Solution ๐
Removing all target actions from a UIButton can be achieved by following these simple steps:
Iterate through the button's control events.
For each control event, remove all associated target actions.
Here's the code you need to achieve this:
button.removeTarget(nil, action: nil, for: .allEvents)
This single line of code will remove all target actions from the button, giving you a clean slate to work with. ๐งนโจ
Let's break down what this line of code does:
removeTarget
is a method that removes a target-action pair from the button.The
nil
parameter for the target means all targets associated with the button will be removed.The
nil
parameter for the action means all actions associated with the button will be removed.Finally,
.allEvents
specifies that we want to remove target actions for all control events.
By using these parameters, you can remove all target actions from your UIButton, no matter how many were added in the first place. ๐
Example ๐
Let's say you have a UIButton called myButton
and you added the following target-action pairs:
Target:
self
, Action:buttonTapped
, Control Event:.touchUpInside
Target:
myViewController
, Action:doSomething
, Control Event:.touchUpInside
Target:
anotherObject
, Action:performAction
, Control Event:.touchDown
To remove all these target actions, simply call the removeTarget
method as follows:
myButton.removeTarget(nil, action: nil, for: .allEvents)
Voila! The UIButton is now free of any target-action pairs. ๐
Your Turn to Take Action! โ๏ธ
Now that you know how to remove all target actions from a UIButton, it's time to take action! Put your newfound knowledge to good use and start fresh with your UIButton's target-actions. Share your experience with us in the comments section below. We'd love to hear how this guide helped you! ๐๐ฌ
And remember, sharing is caring! If you found this guide helpful, hit that share button and spread the knowledge to other developers who might also be struggling with the same issue. Together, we can make coding easier and more enjoyable for everyone! ๐๐ฃ
Happy coding! ๐ปโจ