UIButton inside a view that has a UITapGestureRecognizer
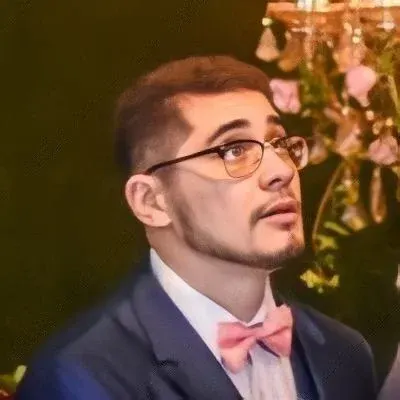
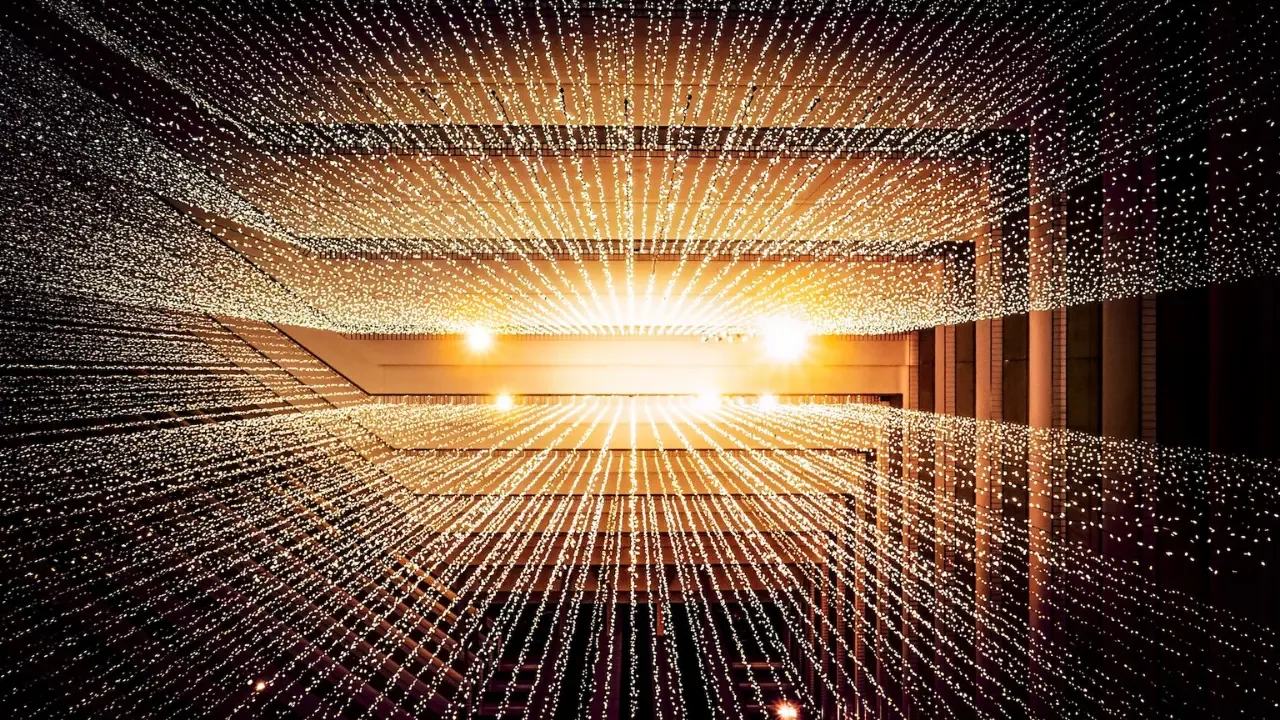
🎯 Are Your Buttons Not Responding Inside a View with UITapGestureRecognizer?
Have you ever encountered a situation where you have a view with a UITapGestureRecognizer, and when you tap on the view, another view appears above it with buttons? But when you try to interact with these buttons, only the tap gesture action gets triggered, and the buttons remain unresponsive? 🤔
Well, fret not! You are not alone in this struggle. This issue often arises due to the conflict between the UITapGestureRecognizer and the button's touch events. But fear not, dear reader, for I have unearthed the solutions to this conundrum! 🕵️♀️
💡 Understanding the Problem
When a UITapGestureRecognizer is added to a view, it intercepts touch events and performs the specified action when the view is tapped. However, this can cause issues with buttons placed within the view, as the tap gesture takes precedence over the button's touch events. As a result, the button actions are ignored, leaving you frustrated and buttonless. 😫
🚀 Easy Solutions to Regain Button Functionality
Fortunately, there are multiple ways to tackle this problem, depending on your specific requirements. Let's explore some easy solutions:
Solution 1: Disable the Tap Gesture Recognizer
If you don't need the tap gesture functionality after the buttons appear, you can disable the tap gesture recognizer, ensuring that the buttons can receive touch events. Here's an example of how to achieve this in code:
yourTapGestureRecognizer.isEnabled = false
This simple line of code will disable the tap gesture recognizer, allowing your buttons to regain their responsiveness. 🙌
Solution 2: Add the Buttons Above the View
Alternatively, you can add the buttons above the initial view, rather than within it. By doing so, the buttons will not be affected by the UITapGestureRecognizer, ensuring their actions are registered correctly. This approach eliminates the conflict entirely. 🎉
Solution 3: Implement Gesture Delegate Methods
If you still want the tap gesture functionality while retaining button interactivity, you can delve into the world of gesture delegate methods. By implementing these methods, you can control the interaction between the gesture and the buttons. Here's an example:
yourTapGestureRecognizer.delegate = self
Then, conform to the UIGestureRecognizerDelegate
protocol and implement the following delegate method:
func gestureRecognizer(_ gestureRecognizer: UIGestureRecognizer, shouldReceive touch: UITouch) -> Bool {
return !touch.view!.isDescendant(of: yourButton)
}
This code ensures that the gesture recognizer will ignore touches on the button, allowing the button actions to be triggered as expected. 🤝
📢 Your Turn to Take Action!
Now that you have the knowledge to resolve the issue of unresponsive buttons within views with UITapGestureRecognizer, it's time to put it into practice! Choose the most suitable solution for your scenario and start enjoying fully functioning buttons once again. Don't let those buttons be a mere showpiece! 💪
If you found this guide helpful in untangling the mysteries surrounding this problem, share it with your fellow developers. Together, we can rid the world of unresponsive buttons! 🌍💥
Let us know in the comments below which solution worked best for you or if you have any other exciting button-related tales to share. Let's keep the conversation going! 💬
Happy coding! 😊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
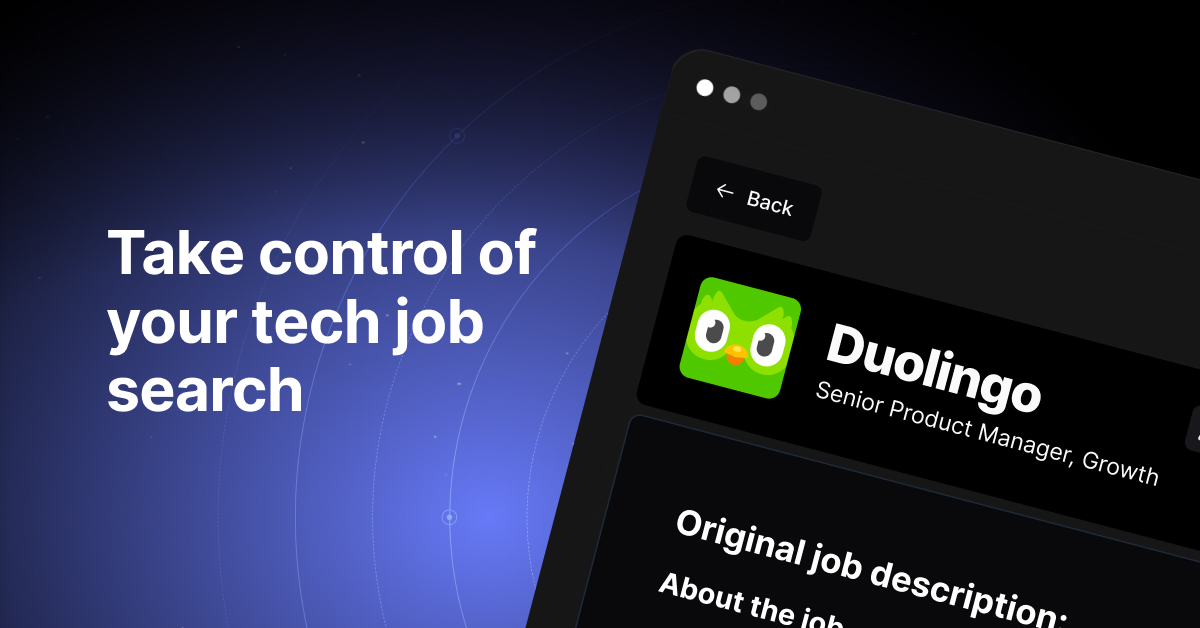