UIActivityViewController crashing on iOS 8 iPads
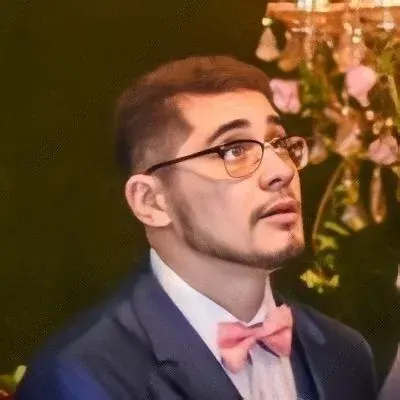
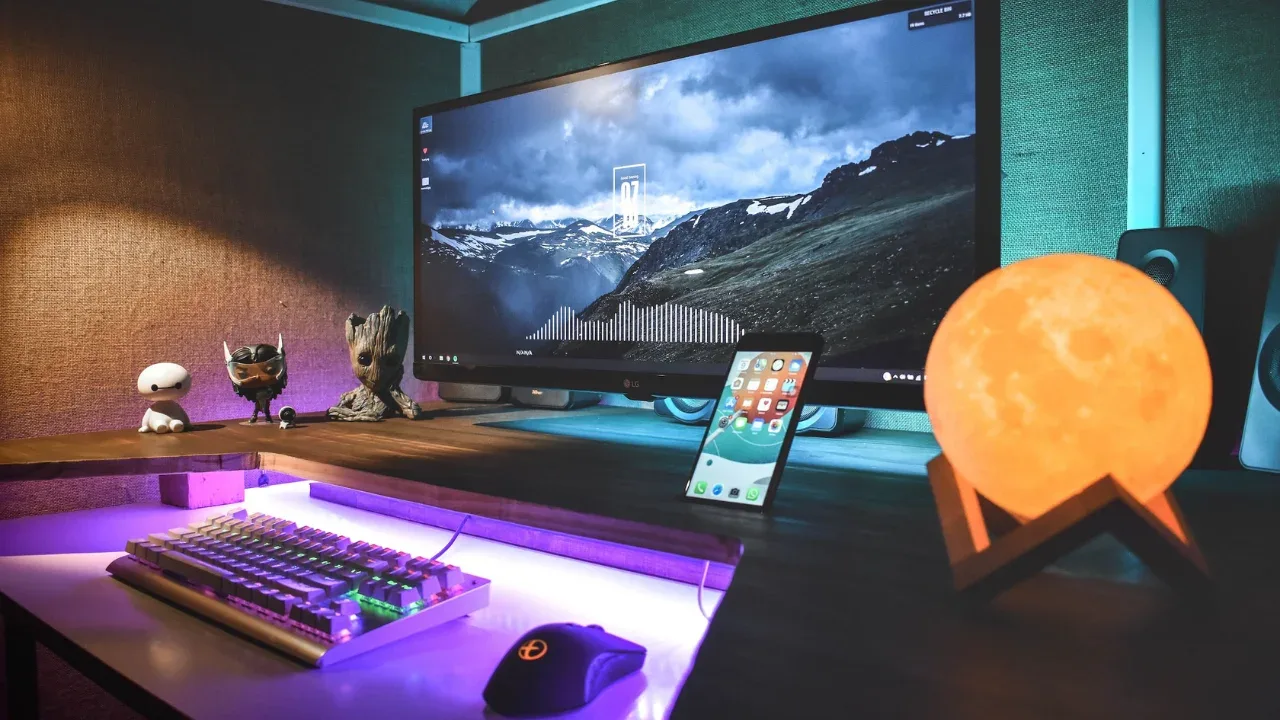
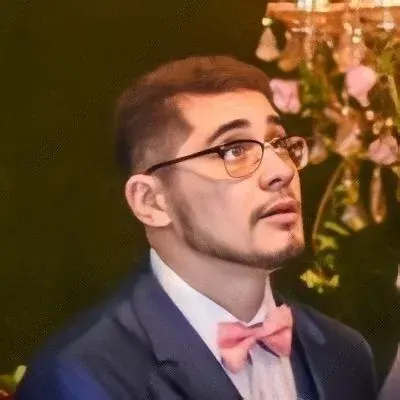
Crashing Issues with UIActivityViewController on iOS 8 iPads: A Comprehensive Guide
Hey there, fellow app developers! 👋 Are you facing a frustrating issue with your UIActivityViewController crashing on iOS 8 iPads? 😫 Don't worry, you're not alone! Many developers have encountered similar problems, but fear not, for I am here to guide you through this sticky situation and help you find a solution. 🚀
Understanding the Issue
The error message you're seeing is a NSGenericException caused by a missing or improperly set sourceView
or barButtonItem
in the UIPopoverPresentationController. An UIPopoverPresentationController
is responsible for managing the presentation of content in a popover style view.
The Possible Cause
In iOS 8, Apple introduced adaptive presentation, which allows applications to take advantage of the available space and presentation style on different devices. This adaptive behavior can cause issues when presenting a UIActivityViewController
on an iPad, specifically on iOS 8.
The crash occurs because the UIPopoverPresentationController
associated with the UIActivityViewController
requires a valid sourceView
or barButtonItem
to determine where the popover should be presented from. Without this information, the app crashes with the provided error message.
The Solution
To resolve this crashing issue, you need to ensure that you properly set the sourceView
or barButtonItem
for the UIActivityViewController
before presenting it on iOS 8 iPads.
UIActivityViewController *activityViewController = [[UIActivityViewController alloc] initWithActivityItems:nil applicationActivities:nil];
activityViewController.excludedActivityTypes = @[UIActivityTypeCopyToPasteboard];
// Set the source view or bar button item for iPad (iOS 8 and above)
if ([activityViewController respondsToSelector:@selector(popoverPresentationController)]) {
activityViewController.popoverPresentationController.sourceView = self.view;
// Alternatively, you can set a specific bar button item
// activityViewController.popoverPresentationController.barButtonItem = self.navigationItem.rightBarButtonItem;
}
[self presentViewController:activityViewController animated:YES completion:nil];
By setting the sourceView
or barButtonItem
for the UIPopoverPresentationController
, you provide the necessary information for the popover to be presented correctly on iPads running iOS 8 or later.
A Word of Caution
It's important to note that the popoverPresentationController
property is only available on devices running iOS 8 or later. Therefore, you should always check for its availability using respondsToSelector:
before setting the source view or bar button item.
Conclusion
Now you're on your way to resolving the crashing issue with UIActivityViewController on iOS 8 iPads! 💪 Remember to set the sourceView
or barButtonItem
property of the UIPopoverPresentationController
to ensure smooth and error-free presentation.
If you found this guide helpful, don't hesitate to share it with other developers who might be facing the same issue. Together, we can make the iOS development community even stronger! 🤝
Got any questions or other iOS development challenges you'd like me to tackle? Let me know in the comments section below. Happy coding! 🚀