Swift - encode URL
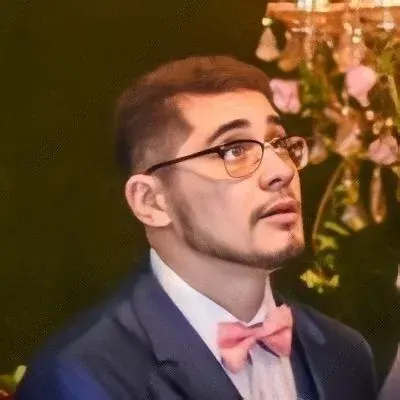
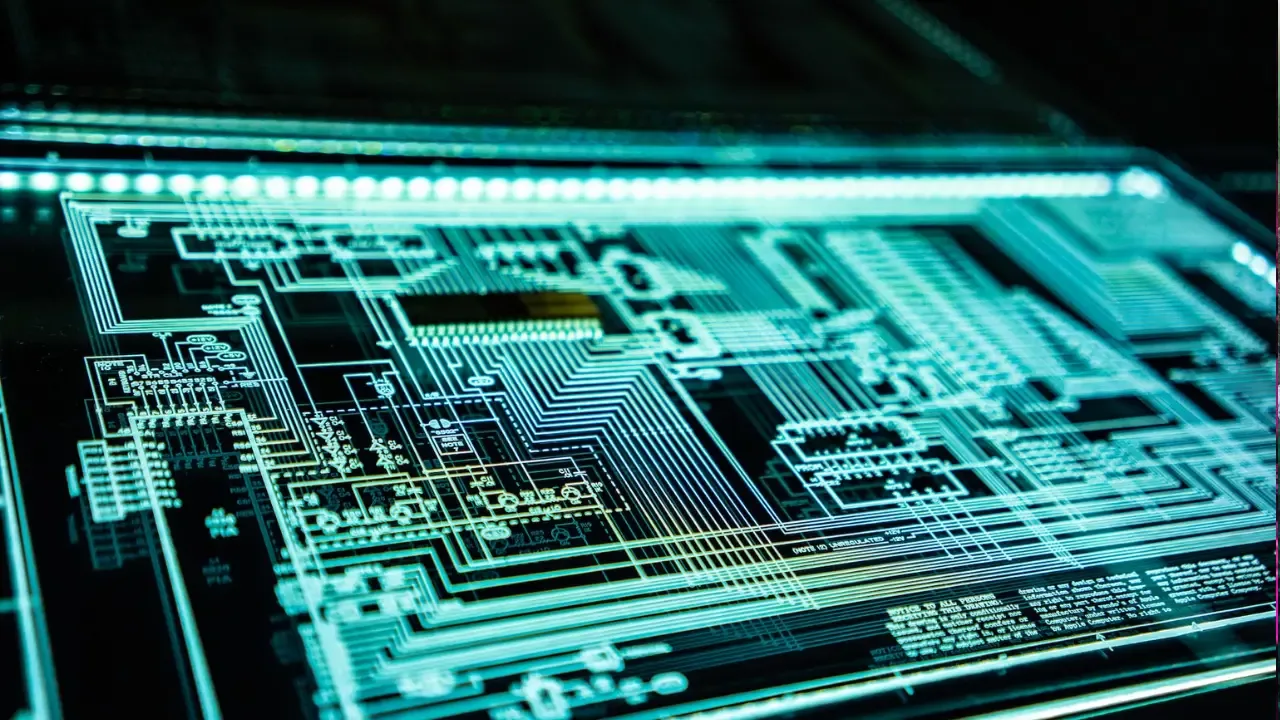
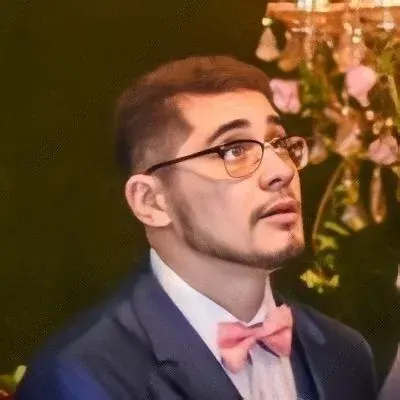
How to Encode URLs in Swift
So you want to encode a URL in Swift, huh? You've come to the right place! In this post, we'll guide you through common issues and provide easy solutions to get your URLs properly encoded.
The Problem: Slashes Not Being Escaped
Let's start with the specific problem mentioned. When using the stringByAddingPercentEscapesUsingEncoding
function to encode a string, you might have noticed that it doesn't escape slashes (/
). This can lead to unexpected behavior when working with URLs.
The Objective-C Solution
The original poster found an Objective-C solution that involved using CFURLCreateStringByAddingPercentEscapes
. While this is a valid solution, it might not be the most straightforward approach for someone working with Swift.
The Swift Solution
Fortunately, Swift provides a simple way to encode URLs using the URLComponents
class. Here's how you can do it:
if let url = URL(string: originalString), let urlComponents = URLComponents(url: url, resolvingAgainstBaseURL: true) {
if let percentEncodedString = urlComponents.string {
print("Encoded URL: \(percentEncodedString)")
}
}
✨ That's it! You've successfully encoded your URL using Swift.
By utilizing the URLComponents
class, you ensure that all components of your URL, including slashes, are properly encoded.
Further Customization
If you need to customize the characters that get escaped, you can manually specify the allowed characters in the URLComponents
initializer. For example:
var allowedCharacterSet = CharacterSet.urlQueryAllowed
allowedCharacterSet.insert("/")
// Add more characters as needed
if let url = URL(string: originalString), let urlComponents = URLComponents(url: url, resolvingAgainstBaseURL: true) {
urlComponents.percentEncodedQuery = urlComponents.percentEncodedQuery?.addingPercentEncoding(withAllowedCharacters: allowedCharacterSet)
if let percentEncodedString = urlComponents.string {
print("Encoded URL: \(percentEncodedString)")
}
}
In this example, we create a CharacterSet
with the default URL-allowed characters, and then we add the slash (/
) to it. Feel free to add any additional characters you wish to escape.
Call-to-Action: Join the Swift Encoding Revolution!
Encoding URLs in Swift doesn't have to be a headache. With the power of URLComponents
, you can quickly and easily encode your URLs to ensure proper functionality.
Give it a try and let us know how it went! Share your thoughts and experiences in the comments below. Happy URL encoding! 🚀💻
Note: Don't forget to check our previous blog posts on Swift tips and tricks.
Do you have any tricks for encoding URLs in Swift? Share them with the community!