Swift - Convert to absolute value
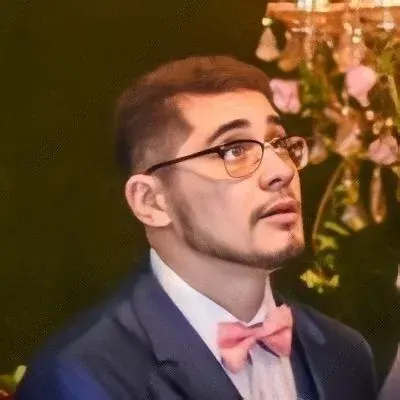
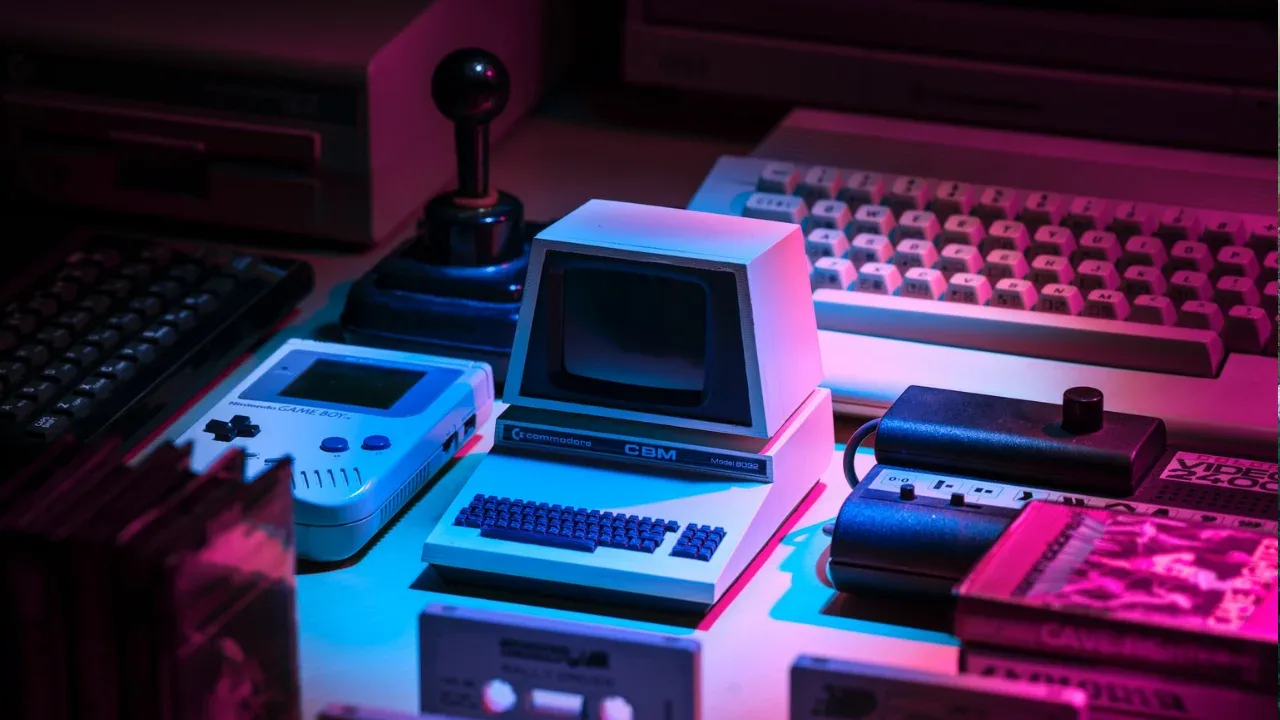
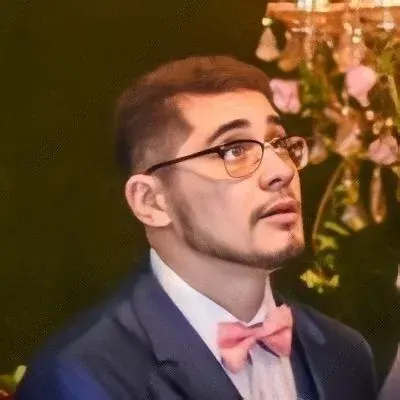
Converting to Absolute Value in Swift: A Quick and Easy Guide ✨📝💻
Are you struggling to find a way to convert an integer to its absolute value in Swift? Look no further because we've got you covered! 🙌
The Problem 😓
Let's say you have an integer value of -8, and you want to convert it to its absolute value, 8. Unfortunately, the straightforward approach of using the UInt()
method doesn't seem to work in this case.
The Solution 🌟
To convert an integer to its absolute value in Swift, you can leverage the abs()
function. This function returns the absolute value of the given number, regardless of its signedness. Here's how you can use it:
let number = -8
let absoluteValue = abs(number)
And just like that, absoluteValue
will be equal to 8! 😎
Explaining the Magic ✨💡
The abs()
function takes a numeric argument and returns its absolute value. It works seamlessly with various numeric types, including integers, floating-point numbers, and even complex numbers. In our case, it efficiently converts the negative integer to its positive counterpart.
Extra Tips and Tricks 🎯
Applying the Absoluteness 😄
The abs()
function is not limited to integers – you can also use it with other numeric types. Whether you're working with a Float
, a Double
, or any other numeric value, the abs()
function will provide you with the absolute value you desire.
Combining abs()
With Math Operations 🧮
You can incorporate the abs()
function into more complex calculations with ease. For example, let's say you have a variable x
that represents the difference between two points:
let x = point1 - point2
let distance = abs(x)
In this case, distance
will hold the absolute value of the difference between the two points.
Spread the Word! 📣
We hope this quick and easy guide has helped you find the solution to converting integers to their absolute values in Swift. No more headaches over this seemingly simple task! 💪
Now, it's your turn to put this knowledge into practice! Try it out in your own projects and let us know how it goes. If you have any further questions or insights to share, feel free to leave a comment below. Happy coding! 😊🚀
Share this article with your fellow Swift developers to help them conquer the absolute value conundrum! 🤝
P.S.: Don't forget to follow us for more exciting Swift tips and tricks! 😉✨
Disclaimer: The information provided in this blog post is for educational purposes only. We do not take responsibility for any misuse or misinterpretation of the techniques discussed here.