Swift apply .uppercaseString to only the first letter of a string
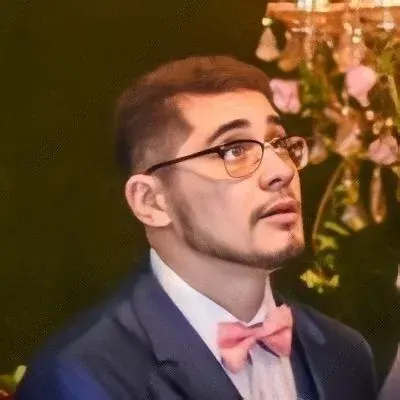
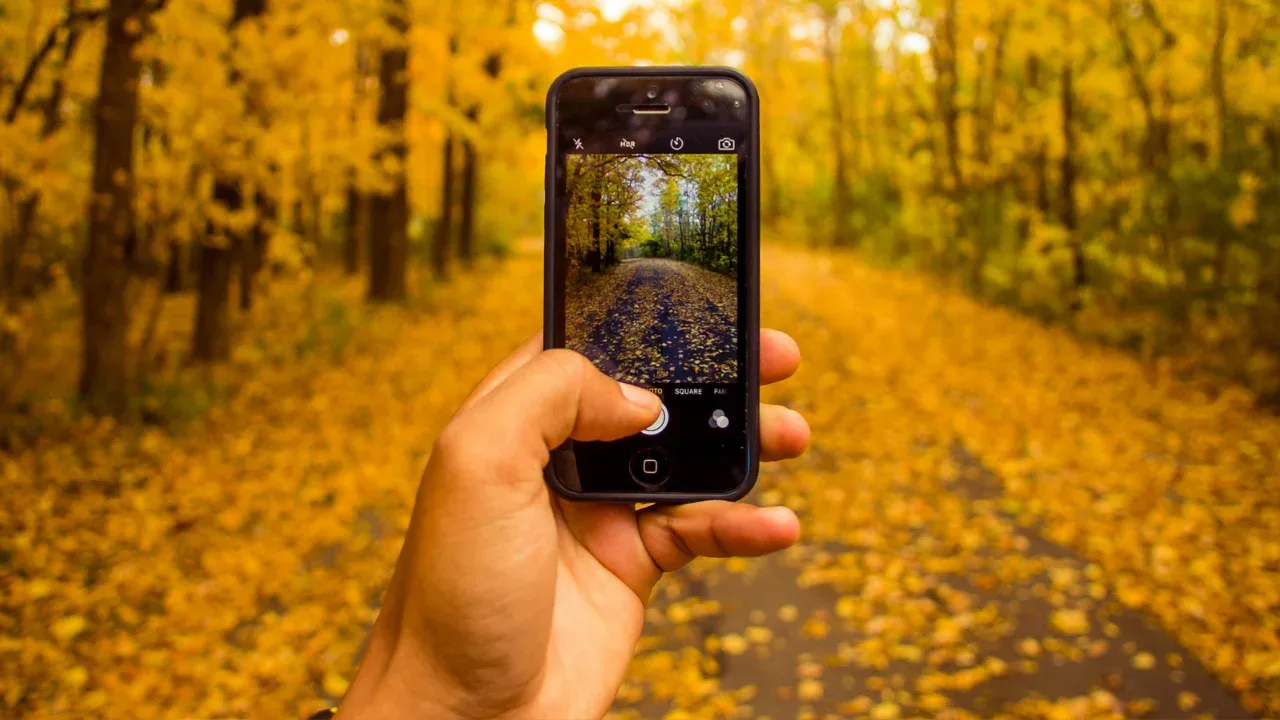
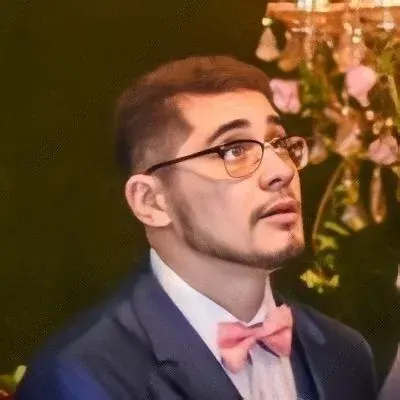
How to Capitalize only the First Letter of a String in Swift
š Hey there, Swift developer! Are you working on an autocorrect system or any other feature where you need to capitalize only the first letter of a string? You've come to the right place! I'll show you how to achieve this in a few simple steps. š
The Problem: Capitalizing Only the First Letter
The user in question wanted to capitalize only the first letter of a string while keeping the rest of the string in lowercase. They were trying to build an autocorrect system but ran into an issue where the replaced word became all lowercase. Let's dive into the solution!
Solution 1: Using .prefix and .capitalized
One way to achieve this is by using the .prefix
method to extract the first letter of the string, and then applying the .capitalized
property to capitalize it. Here's an example:
let input = "apple"
let firstLetter = input.prefix(1).capitalized
let remainingLetters = input.dropFirst()
let output = firstLetter + remainingLetters
print(output) // Output: Apple
In this example, we first extract the first letter of the string input
using .prefix(1)
. We then apply the .capitalized
property to capitalize the first letter. Finally, we concatenate the capitalized first letter with the remaining letters using the +
operator.
Solution 2: Using the Foundation Framework
If you prefer a more concise solution, you can use the capitalized(with:)
method from the Foundation framework. Here's how it works:
import Foundation
let input = "apple"
let output = input.capitalized(with: NSLocale.current)
print(output) // Output: Apple
By importing the Foundation framework, we gain access to the capitalized(with:)
method. It takes an NSLocale
parameter, which we can set to NSLocale.current
to capitalize the string according to the user's locale.
Get the Full String with the First Letter Capitalized
To print the full string with only the first letter capitalized, you can use either of the above solutions and store the result in a variable. Here's an updated example:
// Solution 1: Using .prefix and .capitalized
let input = "apple"
let firstLetter = input.prefix(1).capitalized
let remainingLetters = input.dropFirst()
let output = firstLetter + remainingLetters
print(output) // Output: Apple
// Solution 2: Using the Foundation Framework
import Foundation
let input2 = "apple"
let output2 = input2.capitalized(with: NSLocale.current)
print(output2) // Output: Apple
Feel free to apply these solutions to your autocorrect system or any other scenario where you need to capitalize only the first letter of a string.
Wrapping Up
Now that you know how to capitalize only the first letter of a string in Swift, go ahead and enhance your autocorrect system or any other feature that requires this functionality. š
If you found this guide helpful, consider sharing it with your fellow Swift developers. Let's keep the Swift community growing! š¤ Got questions, suggestions, or other interesting solutions? Leave a comment below and let's discuss! š¬āØ