Set padding for UITextField with UITextBorderStyleNone
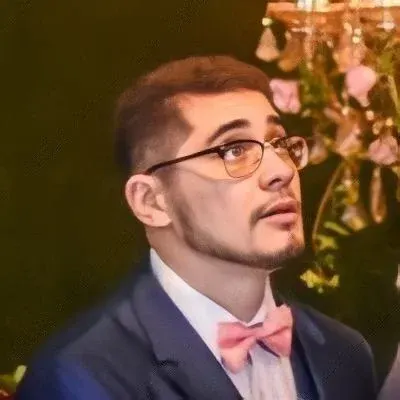

📝🤔📱💭🖌️💬 Hey there tech enthusiasts! 👋 Are you trying to create a customized background for your UITextField but running into issues with the padding when using UITextBorderStyleNone? 😫 Fret not, because I've got you covered! 🔍🔌✨
So, imagine this situation: You've successfully set a custom background image for your UITextField, but the UITextBorderStyleNone option is not giving you the desired padding. Instead, you want it to look similar to UITextBorderStyleRoundedRect. 🤷♂️
First things first, let's understand why this happens. When you set the UITextBorderStyleNone option, it removes the default padding that comes with other border styles. This is why your text sticks to the left without any padding. 😩💔
But hold on a minute! You don't need to feel defeated just yet. There's a simple and easy solution to achieve the desired padding for your UITextField. 🎉✨
To manually set the padding and make it look similar to UITextBorderStyleRoundedRect, you can use the UITextField's leftView
property along with the textRect(forBounds:)
method. 🎨📏
Here's an example of how you can accomplish this in Swift:
// Create a UITextField instance
let textField = UITextField(frame: CGRect(x: 0, y: 0, width: 200, height: 40))
// Set your custom background image
textField.background = UIImage(named: "your_custom_background")
// Create a UIView instance to act as the padding
let paddingView = UIView(frame: CGRect(x: 0, y: 0, width: 10, height: 40))
// Assign the padding view to the UITextField's leftView property
textField.leftView = paddingView
textField.leftViewMode = .always
// Implement the textRect(forBounds:) method to customize the padding
override func textRect(forBounds bounds: CGRect) -> CGRect {
return bounds.inset(by: UIEdgeInsets(top: 0, left: 10, bottom: 0, right: 0))
}
In this example, we've created a UIView instance called paddingView
with a width of 10, which will act as the padding for the left side of the UITextField. By assigning it to the UITextField's leftView
property and setting the leftViewMode
to .always
, we ensure that the padding is always visible.
Additionally, by implementing the textRect(forBounds:)
method and using bounds.inset(by:)
, we can achieve the desired padding by adjusting the left edge of the UITextField's bounds.
And voila! 🎩🌟 With this elegant solution, you can now enjoy the combined benefits of your custom background image and the padding similar to UITextBorderStyleRoundedRect.
But wait, there's more! 📣💬 I want to hear from you! Have you encountered any other UITextField-related challenges? Let me know in the comments below. 👇🗨️
So, next time you face any padding issues with UITextField, just remember these simple steps and triumph over the UITextBorderStyleNone challenge! 🚀💪
Until the next tech adventure, happy coding! 😄👨💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
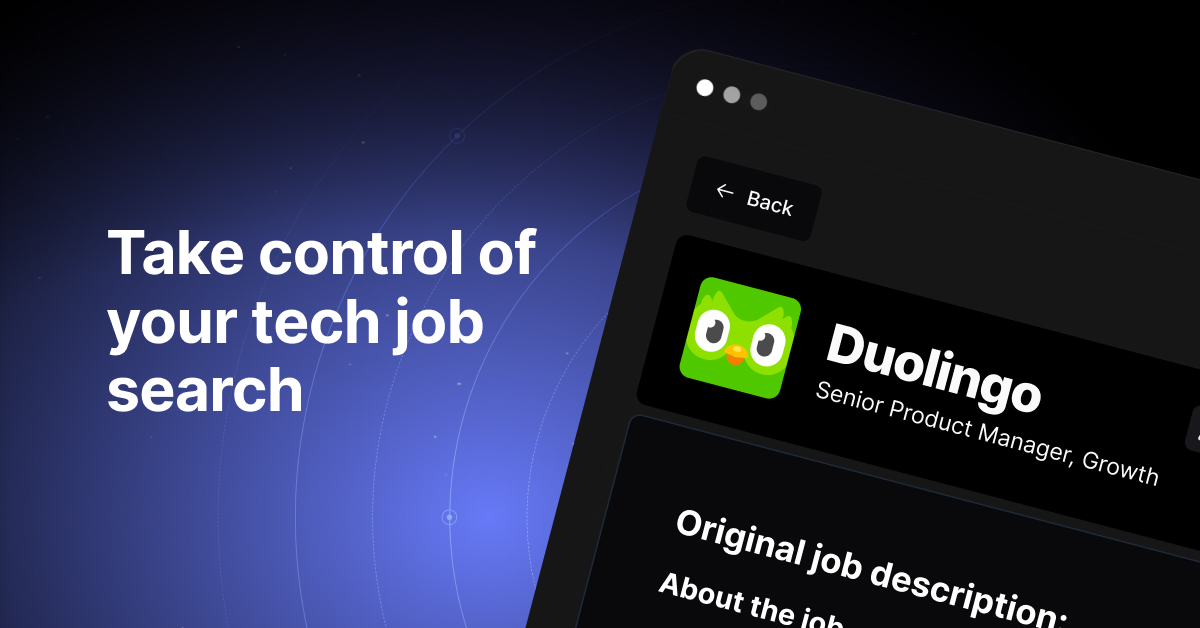