Programmatically set the initial view controller using Storyboards
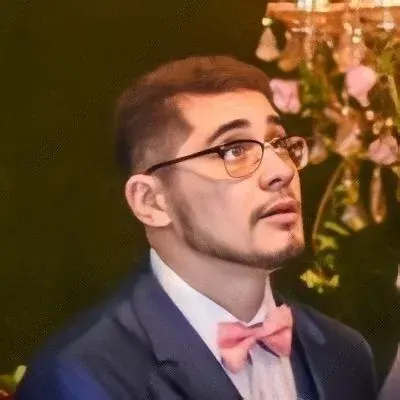
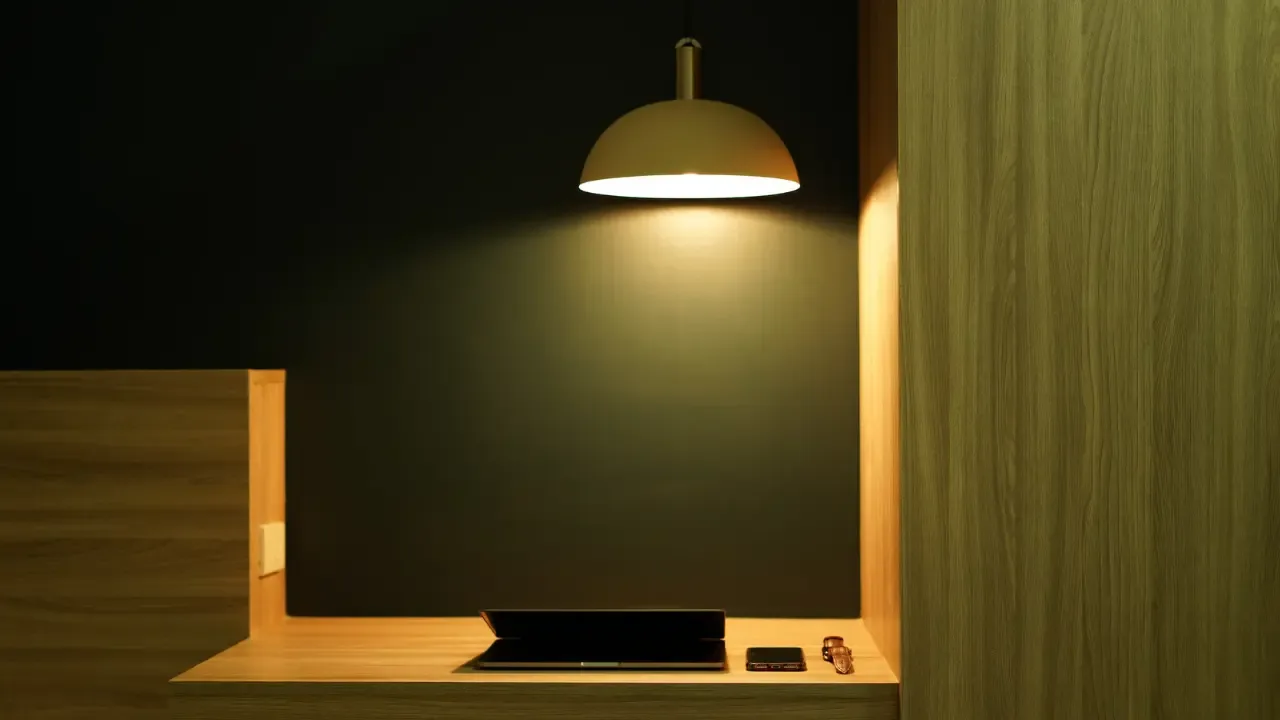
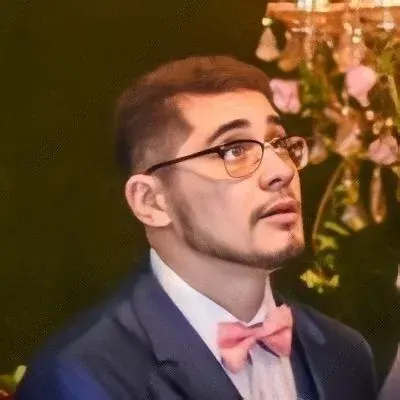
📱💡 Setting the Initial View Controller Programmatically in Storyboards
So you've got a Storyboard and you want to dynamically control which view controller is displayed when your app launches. 🚀 Don't worry, it's easier than you might think! In this guide, we'll walk through the common issues developers face and provide easy solutions to programmatically set the initial view controller using Storyboards. Let's dive in! 💪
The Problem
You have a storyboard with multiple view controllers and want to change the initial view controller based on some condition, such as user preferences or app settings. You don't want your users to always see the same screen when they launch the app - you want to show them the most relevant view right away!
The Solution
To programmatically set the initial view controller in a storyboard, follow these steps:
Open your storyboard file in Xcode.
Select the view controller that you want to set as the initial view controller.
In the Attributes Inspector pane on the right side of Xcode, check the "Is Initial View Controller" box.
Repeat steps 2 and 3 for each potential initial view controller, as needed.
By following these steps, you've now set the initial view controller in your storyboard. But how do you dynamically control which view controller is displayed when the app launches? That's where a little bit of code comes in! 💻
Programmatically Determining the Initial View Controller
To dynamically set the initial view controller based on some condition, we'll use a few lines of code in your AppDelegate. Open the AppDelegate.swift
file and add the following code to the didFinishLaunchingWithOptions
method:
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
let storyboard = UIStoryboard(name: "Main", bundle: nil) // Replace "Main" with your storyboard name
var initialViewController: UIViewController
// Your condition to determine the initial view controller
if someCondition {
initialViewController = storyboard.instantiateViewController(withIdentifier: "ViewController1") // Replace "ViewController1" with the identifier of the desired view controller
} else {
initialViewController = storyboard.instantiateViewController(withIdentifier: "ViewController2") // Replace "ViewController2" with the identifier of another desired view controller
}
window?.rootViewController = initialViewController
window?.makeKeyAndVisible()
return true
}
Let's break down what's happening here. We're creating an instance of our storyboard (replace "Main"
with your actual storyboard name) and assigning it to the storyboard
constant. Then, we declare a variable initialViewController
without assigning it just yet.
Next, we use an if-else
statement (or any condition you prefer) to determine which view controller should be set as the initial view controller. We retrieve the desired view controller using storyboard.instantiateViewController(withIdentifier:)
and assign it to initialViewController
.
Finally, we set initialViewController
as the root view controller of the app's window, make it key and visible, and we're good to go! 🎉
Conclusion
Setting the initial view controller programmatically in Storyboards is a powerful way to dynamically control the flow of your app. By following the steps outlined in this guide and adding a few lines of code to your AppDelegate, you can provide a personalized and relevant user experience right from the start.
Now that you know how to handle this tricky issue, go ahead and make your app shine with a customized initial view controller! If you have any questions or need further assistance, feel free to leave a comment below. Happy coding! 💻🚀