Programmatically create a UIView with color gradient
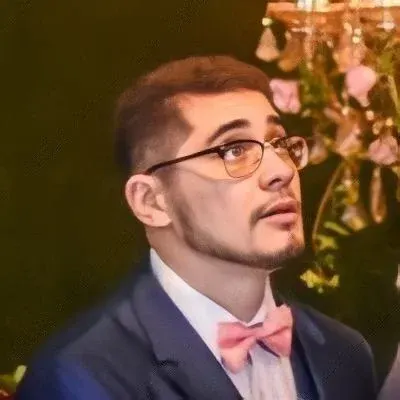

How to Programmatically Create a 🌈 UIView with Color Gradient in Swift
So, you want to create a UIView with a stunning color gradient? Look no further! In this guide, we'll walk you through the steps to achieve this programmatically in Swift. 🚀
The Problem:
Creating a view with a color gradient can be a bit tricky, especially when you want to generate it dynamically at runtime.
The Solution:
Luckily, with a few lines of code, you can achieve this effect seamlessly. Here's how you can do it:
Create a new UIView subclass, let's call it
GradientView
. This subclass will be responsible for generating the gradient background.class GradientView: UIView { override class var layerClass: AnyClass { return CAGradientLayer.self } override func awakeFromNib() { super.awakeFromNib() if let gradientLayer = layer as? CAGradientLayer { // Configure your gradient colors here // For example, let's create a gradient from red to transparent gradientLayer.colors = [UIColor.red.cgColor, UIColor.clear.cgColor] } } }
Now, in your ViewController, you can use this
GradientView
class to generate the color gradient for your UIView. Make sure to set the appropriate frame for your view.class MyViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() let gradientView = GradientView(frame: CGRect(x: 50, y: 50, width: 200, height: 200)) view.addSubview(gradientView) } }
That's it! 🎉 You have successfully created a UIView with a color gradient programmatically.
📌 Bonus Tips:
Customize the gradient by adjusting the
colors
property of theCAGradientLayer
.To create a horizontal gradient instead of a vertical one, set the
startPoint
andendPoint
properties of theCAGradientLayer
.
Now It's Your Turn!
Go ahead and give it a try! Create unique and eye-catching color gradients for your views. Play around with different colors and gradients to make your app truly stand out. 💪
Remember, sharing is caring! If you found this guide helpful, don't hesitate to share it with your fellow developers. Comment below to let us know how it worked for you or if you have any questions. Happy coding! 😊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
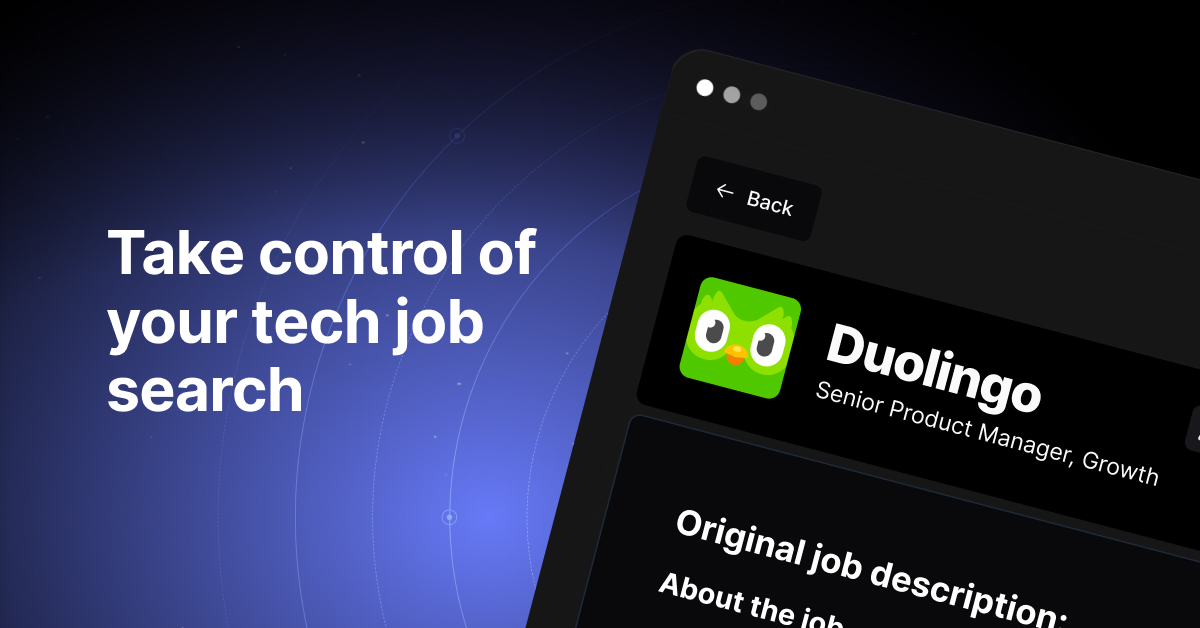