Programmatically add custom event in the iPhone Calendar
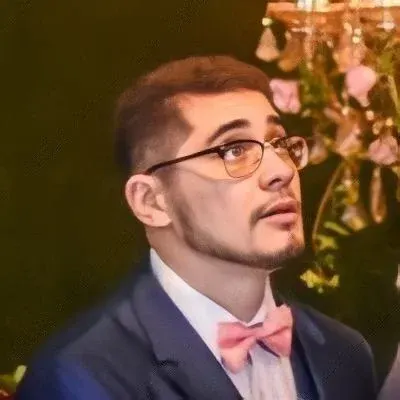
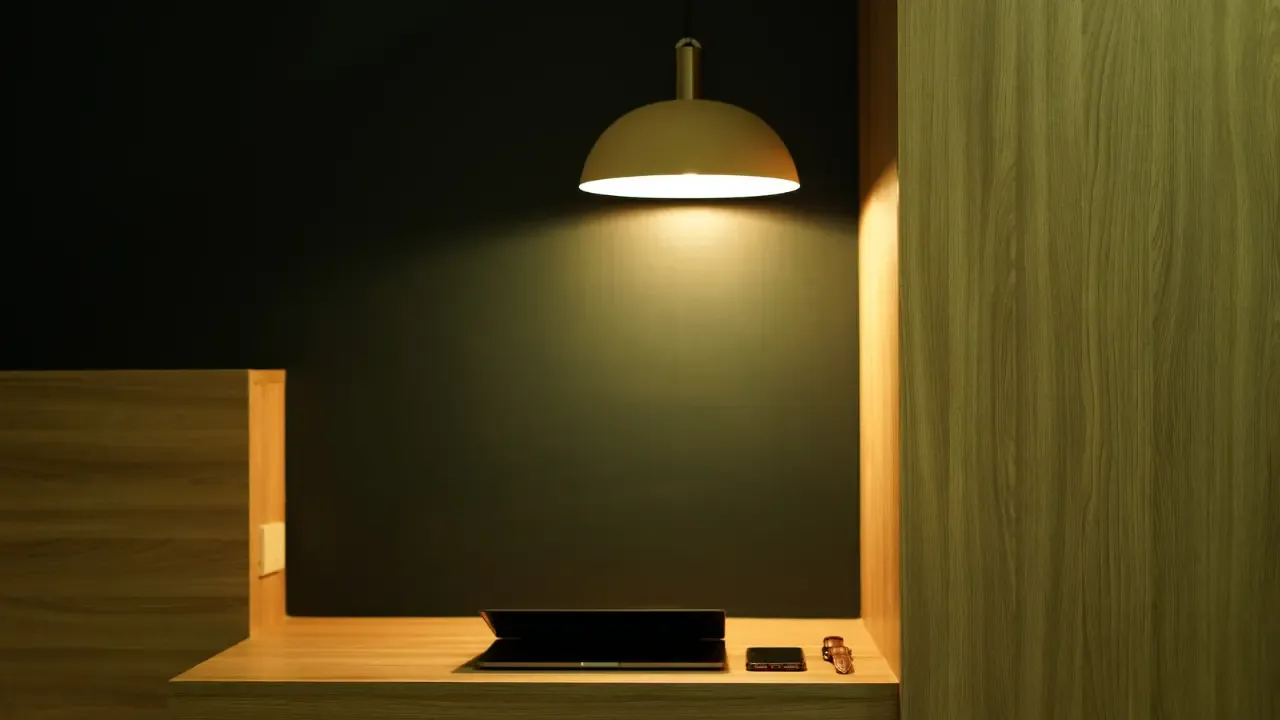
📅 How to Programmatically Add Custom Events in the iPhone Calendar 📱
So, you want to add iCal events to the iPhone Calendar from your custom app? You've come to the right place! 😎 In this guide, we'll address common issues and provide easy solutions to help you achieve this goal. Let's dive in! 🚀
The Challenge 🤔
Adding custom events to the iPhone Calendar programmatically can be a bit tricky. Apple has some restrictions in place to ensure user privacy and prevent abuse. But fear not! We'll show you how to overcome these obstacles. 💪
The Solution 💡
To programmatically add custom events to the iPhone Calendar, you can leverage the EventKit framework provided by Apple. This framework allows you to interact with the user's calendar and create events seamlessly. Here are the steps to get you started:
Import the EventKit framework into your project.
import EventKit
Request access to the user's calendar. Add the following code to your app's permission request flow:
let eventStore = EKEventStore() eventStore.requestAccess(to: .event) { (granted, error) in if granted { // Access granted, proceed with event creation } else { // Access denied, handle accordingly } }
Create and configure your custom event using the EKEvent class.
let event = EKEvent(eventStore: eventStore) event.title = "Your event title" event.startDate = Date() // Set your desired start date event.endDate = Date() // Set your desired end date event.notes = "Additional event details"
Add the event to the user's calendar using the EKEventStore.
do { try eventStore.save(event, span: .thisEvent) // Event saved successfully } catch { // Error occurred while saving event }
That's it! 🎉 You've now successfully added a custom event to the iPhone Calendar programmatically. Your app users will be able to view and manage these events directly from their device's calendar app.
Common Issues and Troubleshooting 🛠️
Issue 1: "Access to the calendar was denied."
If you encounter this issue, make sure you have properly requested access to the user's calendar and handle the access denial case gracefully. You can prompt the user to grant access again through the app's settings.
Issue 2: "Error occurred while saving event."
This error can occur due to various reasons, such as invalid event data or a problem with the user's calendar settings. Double-check the event details and ensure your app handles potential errors robustly.
Engage and Spread the Word! 📣
We hope this guide has helped you overcome the challenges of programmatically adding custom events to the iPhone Calendar. Now it's your turn to take action! ✨
👍 Try implementing this feature in your app and let us know how it goes.
🔄 Share this blog post with your fellow developers and spread the knowledge.
💬 Leave a comment below if you have questions or want to share your experience.
Remember, adding custom events to the iPhone Calendar can greatly enhance the user experience of your app. So, don't miss out on this opportunity to create something amazing! 🌟
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
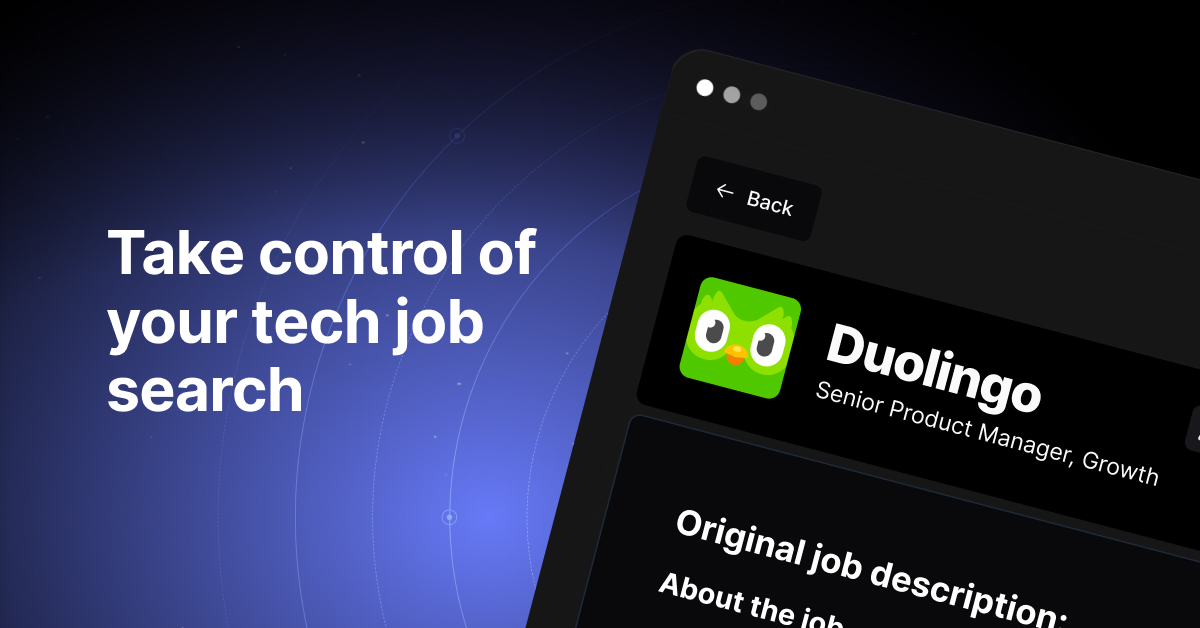