NSUserDefaults - How to tell if a key exists
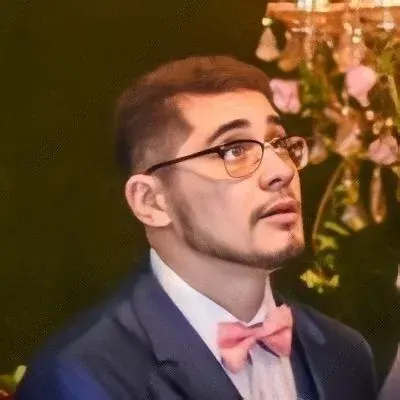
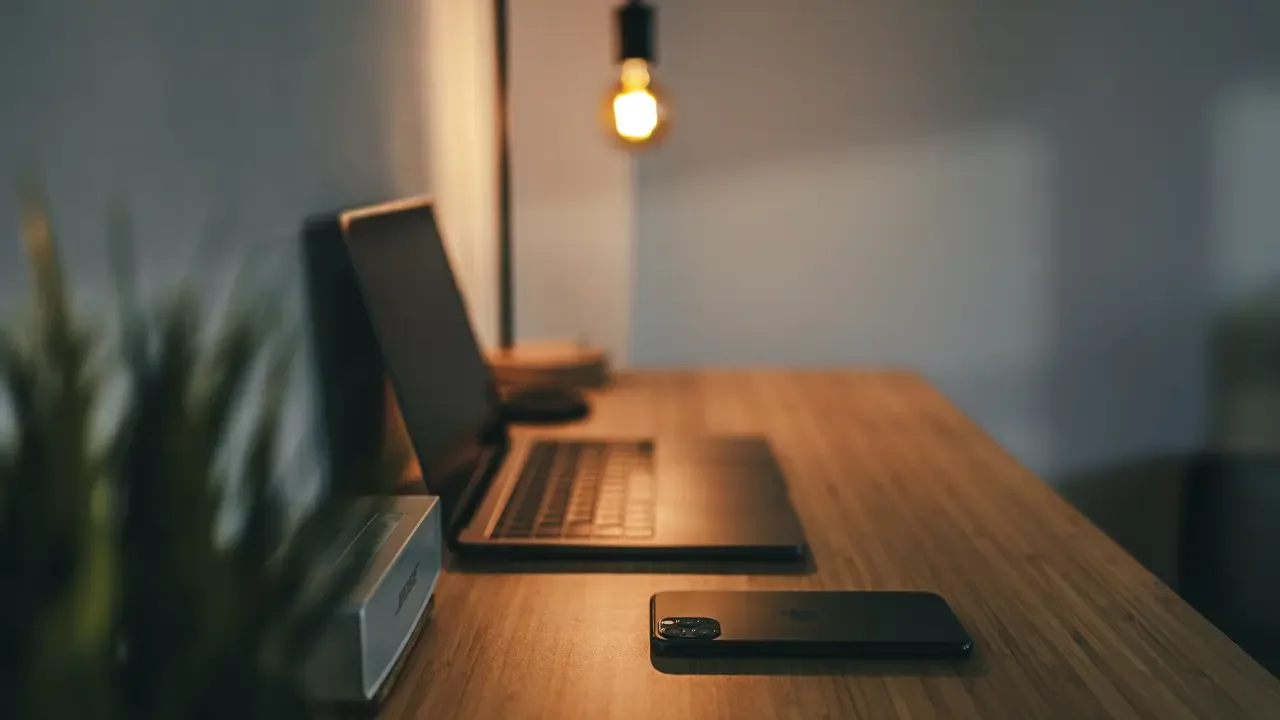
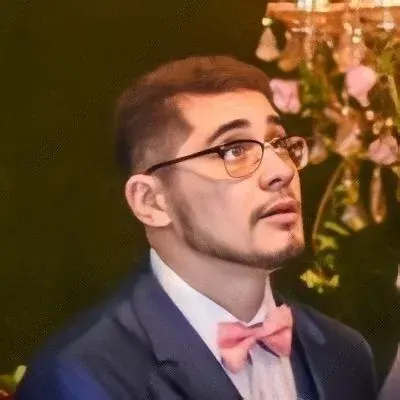
📱🔒 Checking if a key exists in NSUserDefaults 📝
Are you developing an iPhone app and using NSUserDefaults for data persistence? 📲 If so, you might have encountered a common problem: How do you check if a value or a key already exists in NSUserDefaults? 🤔
Well, fear not! I'm here to provide you with some easy-to-implement solutions. Let's dive in! 💪🏼
Option 1: Using objectForKey
One way to check if a key exists in NSUserDefaults is by using the objectForKey
method. Here's an example:
if let value = UserDefaults.standard.object(forKey: "yourKey") {
// Key exists, do something
} else {
// Key does not exist, do something else
}
In this code snippet, we're using the object(forKey: "yourKey")
method to retrieve the value associated with the key "yourKey" from NSUserDefaults. If the key exists and has a value, the if block will be executed. Otherwise, the else block will be executed.
Option 2: Using optional binding with value(forKey:)
Another approach is to use optional binding with the value(forKey:)
method. Here's an example:
if let _ = UserDefaults.standard.value(forKey: "yourKey") {
// Key exists, do something
} else {
// Key does not exist, do something else
}
In this code snippet, we're using optional binding to check if the value for the key "yourKey" exists in NSUserDefaults. If it does, the if block will be executed. Otherwise, the else block will be executed.
Option 3: Using the bool(forKey:)
If you only need to check if a key exists and you don't need the associated value, you can use the bool(forKey:)
method. Here's an example:
if UserDefaults.standard.bool(forKey: "yourKey") {
// Key exists, do something
} else {
// Key does not exist, do something else
}
In this code snippet, we're using the bool(forKey:)
method to directly check if the key "yourKey" exists in NSUserDefaults. If it does, and its value is true
, the if block will be executed. Otherwise, the else block will be executed.
Wrap Up and Take Action!
Now that you have these easy solutions at your disposal, you can confidently check if a key exists in NSUserDefaults and take appropriate actions in your iPhone app. 🙌🏼
But wait... there's more! If you found this guide helpful, why not share it with your fellow iOS developers? They might be struggling with the same issue, and your share could save their day! 🌟
So go ahead, click that share button, and help spread the knowledge! And if you have any more questions or need further assistance, feel free to leave a comment below. Happy coding! 💻💡