NSNotificationCenter addObserver in Swift
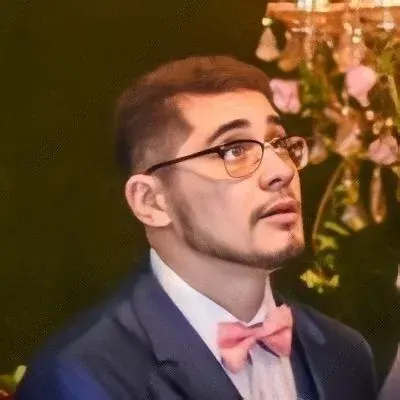
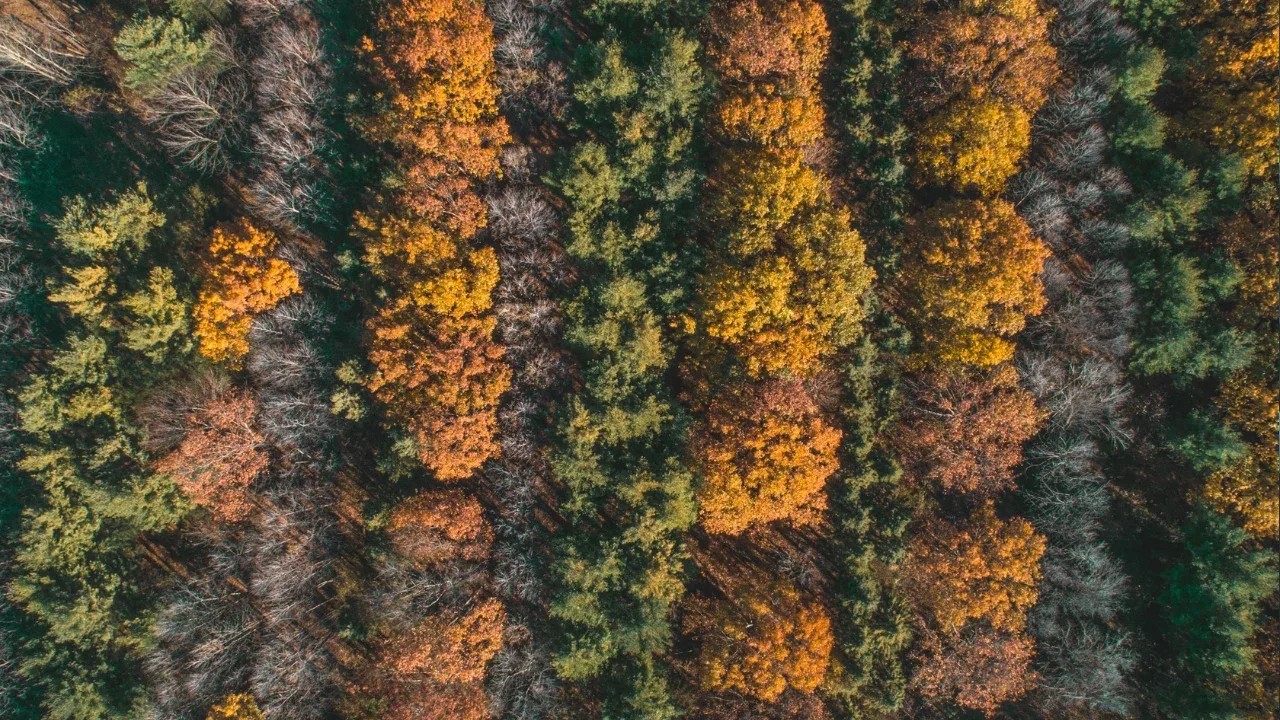
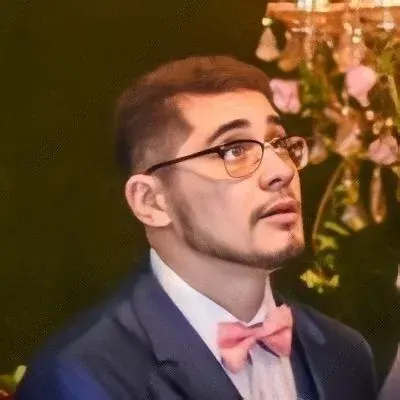
📢 The Ultimate Guide to NSNotificationCenter addObserver in Swift 📢
So you're trying to add an observer in Swift to the default notification center? Great! I'm here to help you navigate through this process and provide easy solutions to any problems you may encounter. 🕵️♀️
The Common Issues 🤔
Let's start by addressing some common issues you may face while using NSNotificationCenter addObserver
in Swift:
Selector not found: You may encounter this error if the
@objc
attribute is missing from your selector method. Remember, in Swift, you need to explicitly declare your selector method with the@objc
attribute to make it accessible to Objective-C. 🎯Notification name mismatch: Double-check that the notification name you're registering for matches the one being posted. Typos or inconsistent naming can prevent your observer from receiving notifications. 🔍
Retain cycles: Be cautious of retain cycles when using
addObserver
within a class. If the observer is not removed properly, your program may leak memory. It's essential to remove the observer when it's no longer needed. 🚨
🚀 Easy Solutions
Let's get to the good stuff! Here are some easy solutions to these common issues:
1. Declaring the selector method
In Swift, add the @objc
attribute before your selector method to bridge it with Objective-C. Here's an example:
@objc func batteryLevelChanged(_ notification: Notification) {
// Handle battery level change here
}
Make sure you replace the existing selector method batteryLevelChanged:
with this Swift equivalent.
2. Notification name consistency
Verify that the notification name you're observing is correct and matches the name used when posting the notification. For example:
NotificationCenter.default.addObserver(self, selector: #selector(batteryLevelChanged(_:)), name: UIDevice.batteryLevelDidChangeNotification, object: nil)
Ensure that the name
parameter is exactly UIDevice.batteryLevelDidChangeNotification
, as shown above.
3. Remember to remove the observer
To prevent memory leaks, it's crucial to remove the observer when it's no longer needed. You can achieve this by calling removeObserver
in the appropriate lifecycle method or when the observer becomes irrelevant.
deinit {
NotificationCenter.default.removeObserver(self)
}
📢 Your Call-to-Action
There you have it! You're now equipped to use NSNotificationCenter addObserver
in Swift like a pro. Start implementing these solutions and say goodbye to any issues you've been facing! 🎉
But wait! If you have any questions or other topics you'd like to dive into, let's connect! Leave a comment below and let's engage in some tech talk. 🔤
Now, go forth and conquer the world of NSNotificationCenter addObserver
in Swift! Remember, tech problems may appear complex, but with the right guidance, they can be as simple as emojis. 😉