Move view with keyboard using Swift
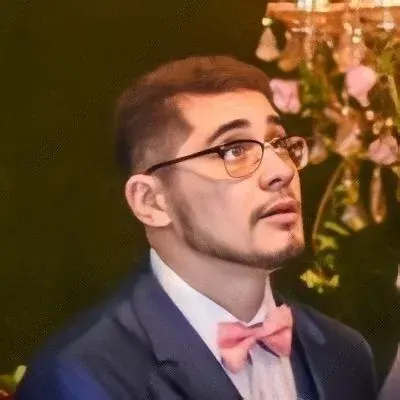
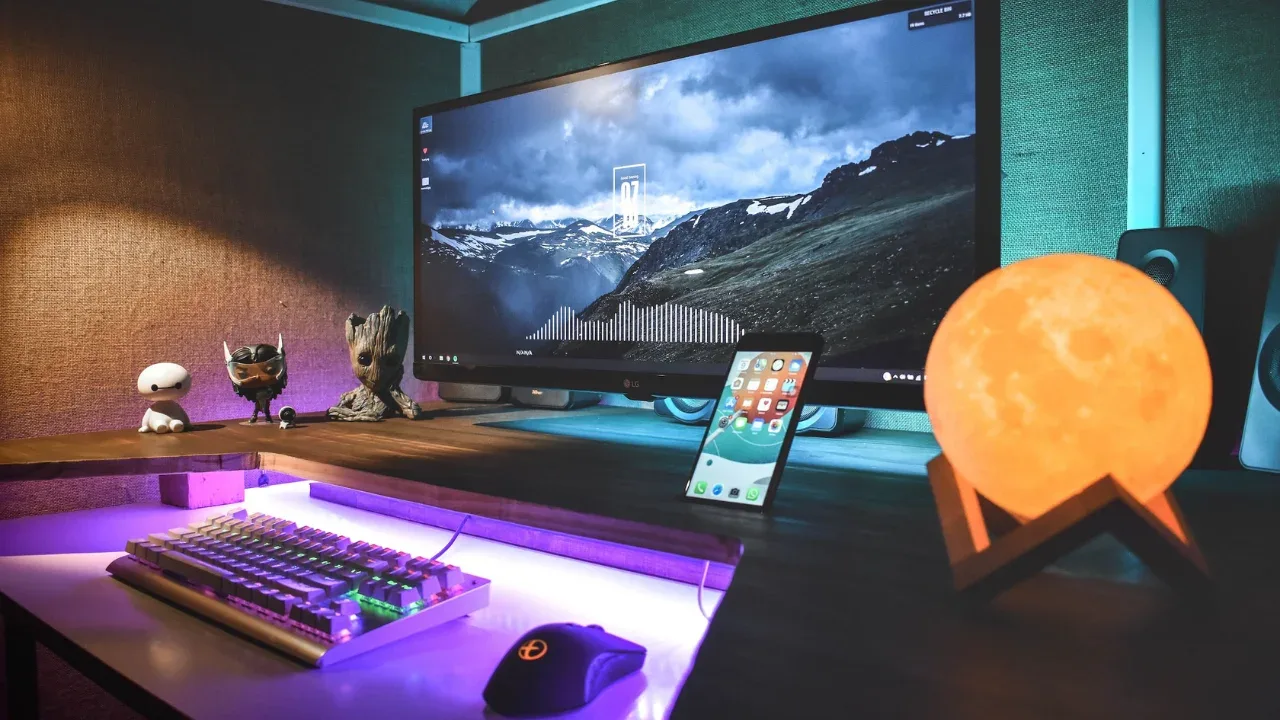
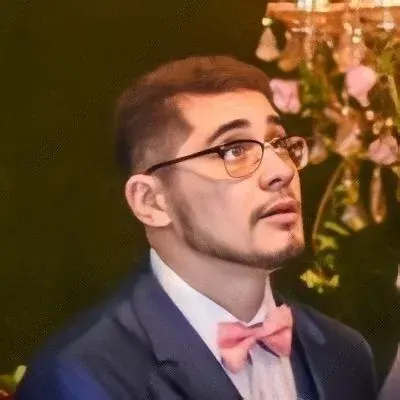
📱 How to Move View with Keyboard Using Swift
Are you frustrated because the keyboard is covering your text field in your Swift app? Don't worry, we've got you covered! In this guide, we'll address the common issue of the keyboard covering the text field and provide you with easy solutions to move the view upwards while typing and back down when the keyboard disappears. Let's dive in! 💪
The Problem: Keyboard Covering Text Field
The user is experiencing a common problem where the keyboard covers the text field in their app. This can be frustrating as it prevents the user from seeing what they're typing and providing a smooth user experience.
Solution: Moving the View Upward with Keyboard Appearance
To solve this problem, we need to make the view move upwards when the keyboard appears and back down when it disappears. Here's how you can achieve this in Swift:
Step 1: Add an observer for the keyboard notifications in your view controller's
viewDidLoad
method:NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillShow(_:)), name: UIResponder.keyboardWillShowNotification, object: nil) NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillHide(_:)), name: UIResponder.keyboardWillHideNotification, object: nil)
Step 2: Implement the
keyboardWillShow
andkeyboardWillHide
methods to handle the keyboard appearance:@objc func keyboardWillShow(_ notification: Notification) { if let keyboardSize = (notification.userInfo?[UIResponder.keyboardFrameEndUserInfoKey] as? NSValue)?.cgRectValue { self.view.frame.origin.y -= keyboardSize.height } } @objc func keyboardWillHide(_ notification: Notification) { if let keyboardSize = (notification.userInfo?[UIResponder.keyboardFrameEndUserInfoKey] as? NSValue)?.cgRectValue { self.view.frame.origin.y += keyboardSize.height } }
Step 3: Remove the keyboard notification observers in
deinit
to avoid memory leaks:deinit { NotificationCenter.default.removeObserver(self) }
And that's it! 🎉 By implementing these steps, your view will smoothly move upwards when the keyboard appears, allowing the user to see what they're typing, and move back down when the keyboard disappears.
Call-to-Action: Engage with the Community
We hope this guide has been helpful to you in solving the issue of the keyboard covering your text field. If you have any further questions or need additional help, don't hesitate to reach out to us or join our active community of Swift developers on our forum. We're always here to help you! 😊
📣 Share your thoughts in the comments below:
Have you encountered this issue before?
Did the solution provided work for you?
Do you have any additional tips or tricks to share?
💡 Remember, by sharing your experiences and insights, you contribute to a collaborative learning environment where everyone benefits!
Now go ahead and implement the solution in your app to enhance the user experience. Happy coding! 💻✨