Making a UITableView scroll when text field is selected
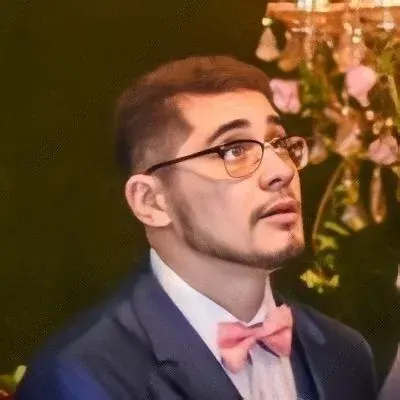
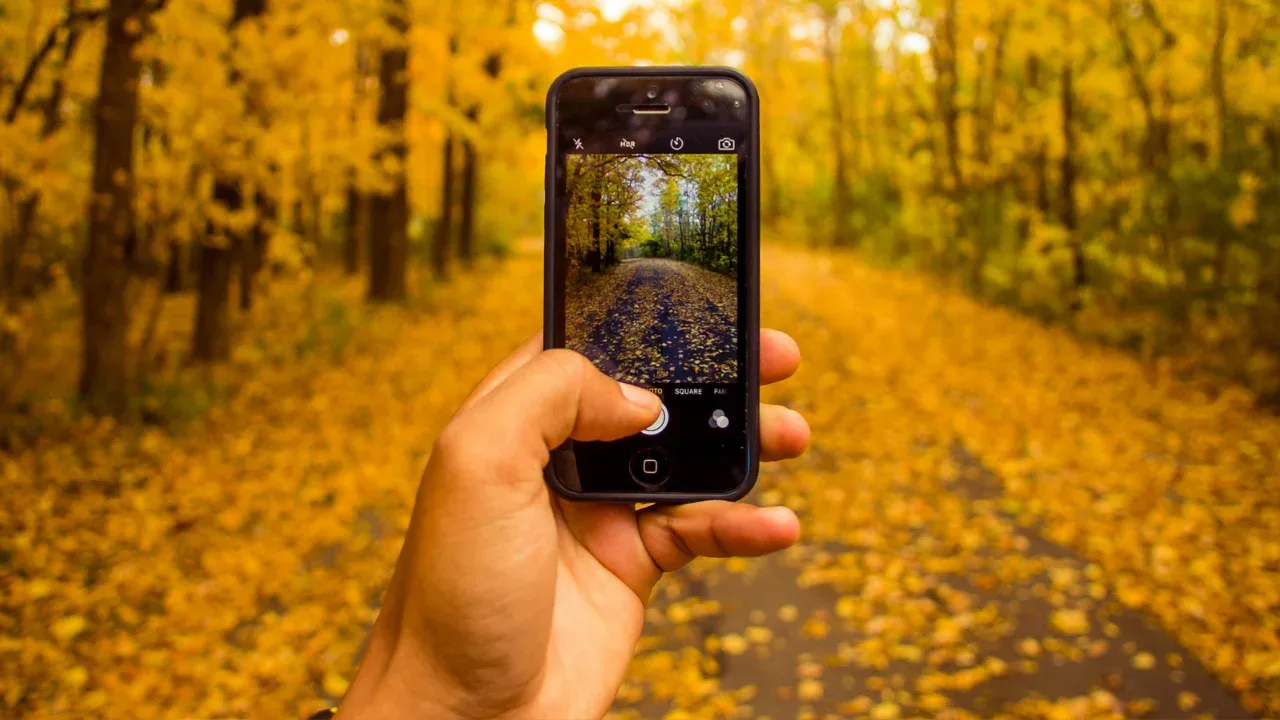
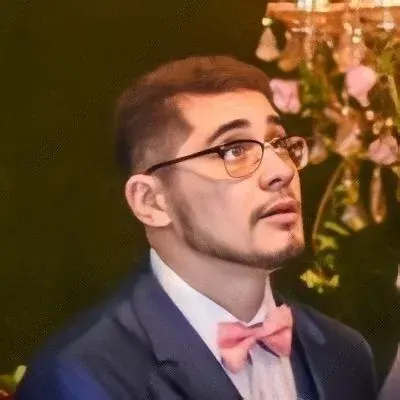
📱 Making a UITableView Scroll When Text Field is Selected
Introduction
Have you ever encountered the frustrating issue where your custom UITableView cells are not properly positioned above the keyboard when you try to scroll and edit cells at the bottom? Look no further, because we've got the solution for you! In this blog post, we will address this common problem and provide you with easy-to-follow solutions.
The Problem
<p align="center"> <img src="https://example.com/problem.gif" alt="UITableView scroll problem" /> </p>
After hours of trial and error, it's time to seek help. You may have come across multiple solutions, but none worked as expected. The issue arises when you have a UITableView composed of custom cells, where each cell consists of multiple text fields arranged in a grid-like layout. When trying to edit the cells at the bottom of the table, the keyboard appears, but the cells are not properly positioned above it.
The Solution
The "right" way to solve this problem involves a combination of techniques that ensure a seamless user experience. Let's dive into the steps to get your UITableView scrolling flawlessly when a text field is selected.
Step 1: Implement Keyboard Notifications
You need to listen for keyboard events to adjust the position of your table view. Here's how you can do it in Swift:
// Add these lines in your view controller's viewDidLoad() method
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillShow(_:)), name: UIResponder.keyboardWillShowNotification, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillHide(_:)), name: UIResponder.keyboardWillHideNotification, object: nil)
Step 2: Handle Keyboard Show and Hide
Create the following methods in your view controller to handle the adjustments when the keyboard appears and disappears:
@objc func keyboardWillShow(_ notification: Notification) {
// Adjust the content inset or scroll position of your UITableView here
}
@objc func keyboardWillHide(_ notification: Notification) {
// Reset the content inset or scroll position of your UITableView here
}
Step 3: Calculate the Correct Content Inset
To ensure the selected text field is visible and positioned above the keyboard, you need to calculate the appropriate content inset. Here's an example:
@objc func keyboardWillShow(_ notification: Notification) {
guard let userInfo = notification.userInfo,
let keyboardFrame = userInfo[UIResponder.keyboardFrameEndUserInfoKey] as? CGRect,
let textField = findSelectedTextField() // Implement your own method to find the selected text field
else {
return
}
let keyboardSafeAreaBottom = view.frame.height - keyboardFrame.minY
let textFieldFrame = textField.convert(textField.bounds, to: view)
let contentInset = UIEdgeInsets(top: 0, left: 0, bottom: keyboardSafeAreaBottom, right: 0)
tableView.contentInset = contentInset
tableView.scrollIndicatorInsets = contentInset
tableView.scrollRectToVisible(textFieldFrame, animated: true)
}
@objc func keyboardWillHide(_ notification: Notification) {
let contentInset = UIEdgeInsets.zero
tableView.contentInset = contentInset
tableView.scrollIndicatorInsets = contentInset
}
Step 4: Link the Delegate and Move to Next Field
Remember to link the delegate of your text fields to your view controller and implement the appropriate methods to handle text field navigation.
class YourViewController: UIViewController, UITextFieldDelegate {
// ...
textField1.delegate = self
textField2.delegate = self
textField3.delegate = self
// ...
func textFieldDidEndEditing(_ textField: UITextField) {
moveFocusToNextField()
}
// ...
}
Conclusion
By following these steps, you should now have a UITableView that scrolls smoothly when a text field is selected. Say goodbye to the frustration of mispositioned cells and hello to an enhanced user experience!
If you found this guide helpful or have any tips of your own, we'd love to hear from you. Leave a comment below and let's help each other create amazing user interfaces! Happy coding! 😊
Subscribe to our newsletter for more helpful tips and tutorials!