Loading/Downloading image from URL on Swift
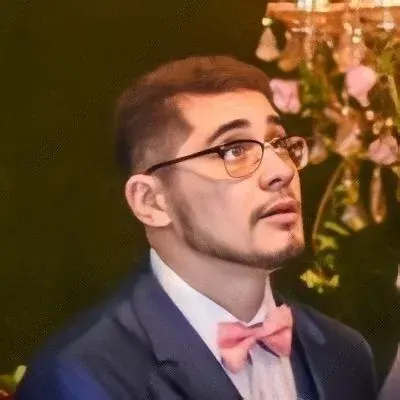
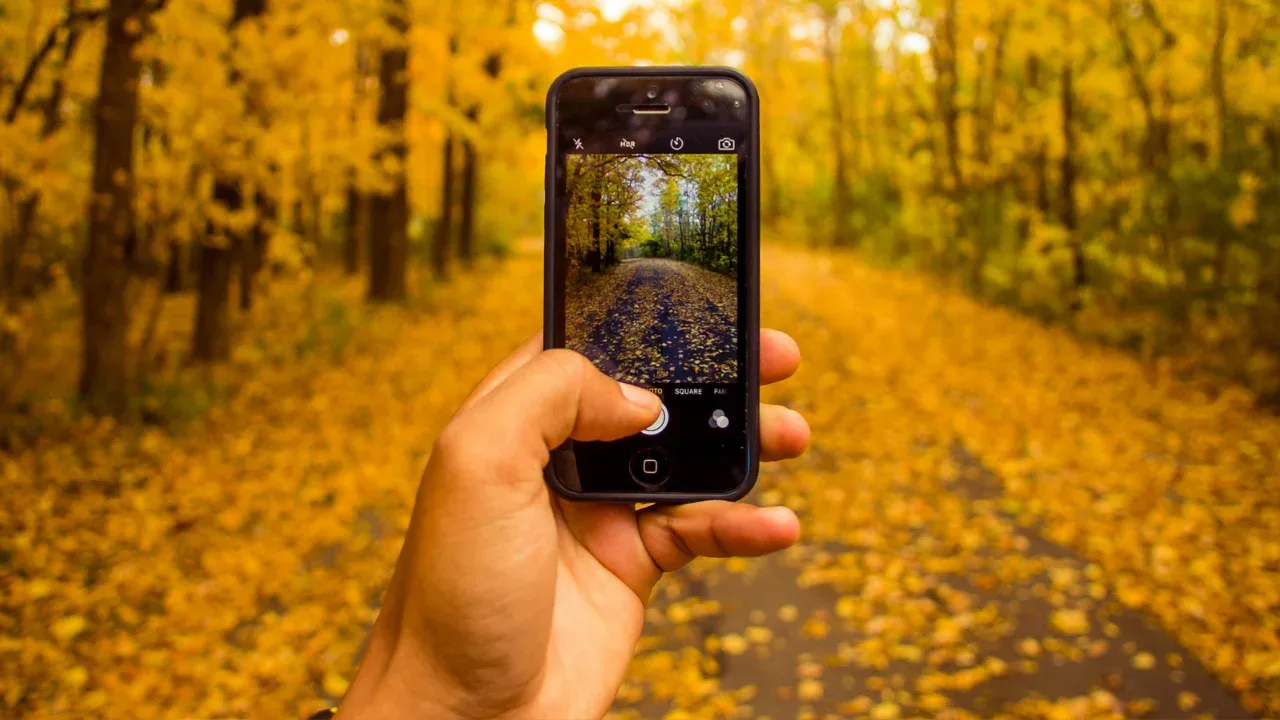
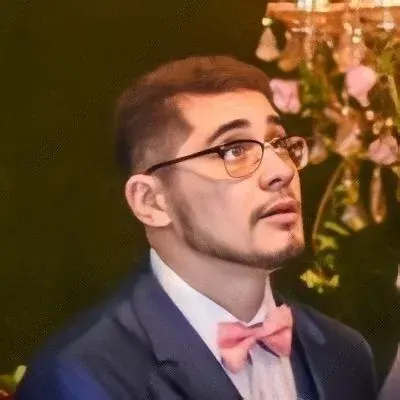
Loading/Downloading Image from URL on Swift: Easy Fix for Compilation Error 👨💻
We all have been there - trying to load an image from a URL in our Swift application, only to hit a compilation error. 😫 Fear not, my fellow Swift enthusiasts! In this blog post, I'll explain why you're facing this issue and provide you with an easy solution to solve it. 🚀
The Compilation Error: 'imageWithData' is Unavailable 🚫
So you want to load an image from a URL using Swift, just like you did successfully with Objective-C. However, when you try to use the imageWithData
method, you encounter the following compilation error:
'imageWithData' is unavailable: use object construction 'UIImage(data:)'
Don't worry, this error is easily fixable! 🛠️
Understanding the Issue: Swift Object Construction 🏗️
In Swift, the syntax for constructing an object is slightly different from Objective-C. Instead of using traditional class methods like imageWithData
, Swift prefers object construction through initializers. 🏗️
To fix the compilation error, you need to use the correct initializer for creating a UIImage from data. In Swift, this is done through the UIImage(data:)
constructor. 🖼️
Solving the Problem: Swift's UIImage Initialization 🎉
Let's modify your code snippet to use the appropriate initializer and eliminate the compilation error. Here's the updated Swift code:
@IBOutlet var imageView: UIImageView
override func viewDidLoad() {
super.viewDidLoad()
let url: URL = URL(string: "http://myURL/ios8.png")!
let data: Data = try! Data(contentsOf: url)
imageView.image = UIImage(data: data) // No more error here 🎉
}
By using URL(string:)
to create a URL object and Data(contentsOf:)
to fetch the image data, we ensure a successful image loading process without any pesky compilation errors. 🙌
Wrapping Up and Bonus Objective-C Comparison 🎁
We've successfully solved the compilation error and embraced Swift's object construction style for loading images from URLs. 🎉
To solidify your understanding, here's your original Objective-C code snippet for loading an image from a URL:
- (void)viewDidLoad {
[super viewDidLoad];
NSURL *url = [NSURL URLWithString:@"http://myURL/ios8.png"];
NSData *data = [NSData dataWithContentsOfURL:url];
_imageView.image = [UIImage imageWithData:data];
}
As you can see, the Objective-C approach using imageWithData
is still valid, but in Swift, we prefer the initializer UIImage(data:)
for a more seamless integration. 🌟
Your Turn: Let's Engage! 💬
I hope this guide helped you understand and solve the compilation error you encountered when loading an image from a URL in Swift. Now it's your turn to share your thoughts and experiences!
Have you ever faced similar issues while working with Swift? What other Swift topics would you like me to cover in future blog posts? Let me know in the comments below! 💬
Happy coding! 🚀💻