Is it possible to allow didSet to be called during initialization in Swift?
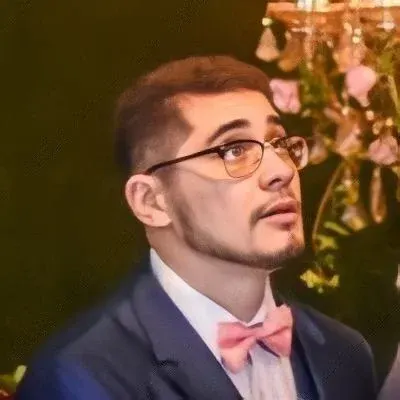
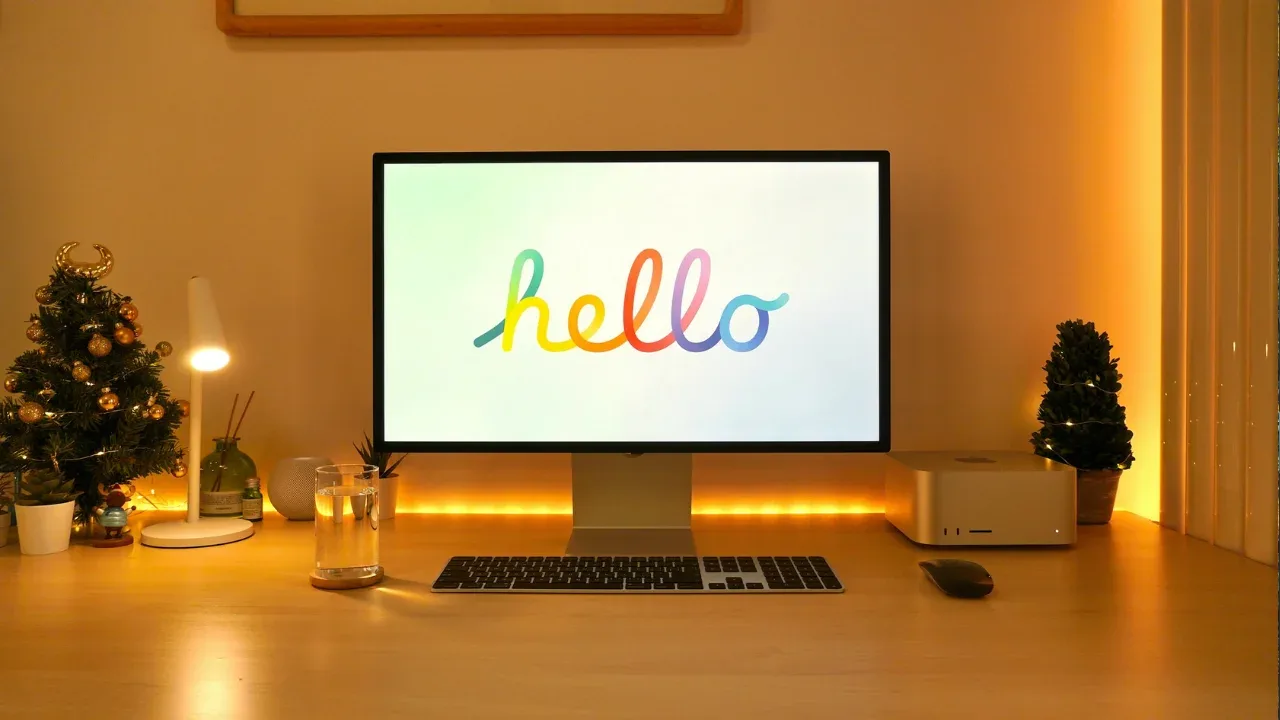
Is it possible to allow didSet to be called during initialization in Swift? 🤔
Have you ever wondered if it's possible to have the didSet
observer called during initialization in Swift? 🤔 Well, according to Apple's docs, didSet
and willSet
observers are not called when a property is first initialized. They are only called when the property's value is set outside of an initialization context. 😕
But what if you really need to perform some important actions as soon as the property is initialized? Let's look at a specific example to understand why this might be useful. 🤓
Imagine you have a class called SomeClass
with a property someProperty
that you want to observe using didSet
. You also have a method called doStuff()
that you want to call every time someProperty
is set. Here's an example of what this might look like:
class SomeClass {
var someProperty: AnyObject {
didSet {
doStuff()
}
}
init(someProperty: AnyObject) {
self.someProperty = someProperty
doStuff()
}
func doStuff() {
// perform some important actions
}
}
In this example, doStuff()
is called both in the init
method and inside the didSet
observer. However, if you read Apple's docs, you might be disappointed to learn that the didSet
observer is not called during initialization. 😞
But fear not, there's a workaround! One possible solution is to remove the convenience initializer and force the property to be set after initialization. This way, you can ensure that the didSet
observer will always be called. Here's an updated version of SomeClass
:
class SomeClass {
var someProperty: AnyObject {
didSet {
doStuff()
}
}
init() {
// do some other initialization if needed
}
func setSomeProperty(_ value: AnyObject) {
self.someProperty = value
doStuff()
}
func doStuff() {
// perform some important actions
}
}
Now, instead of setting someProperty
directly in the initializer, you can use the setSomeProperty()
method to set the property. This ensures that doStuff()
is called every time someProperty
is set.
While this workaround may not be as concise as having the didSet
observer called during initialization, it gives you the flexibility to control when the observer is triggered. 🙌
So, if you find yourself needing to perform actions immediately after property initialization in Swift, consider using this workaround to achieve the desired behavior. Give it a try and let me know how it works for you! 😊
Update:
After further consideration, I've decided to remove the convenience initializer from SomeClass
and require that the property be set after initialization. While this approach may not be suitable for every situation, it works well for my specific use case. 🤷♂️
Now that you know how to force didSet
to be called during initialization, go ahead and put this knowledge into practice! Share your experience or any other solutions you may have in the comments below. Let's learn and grow together! 💪💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
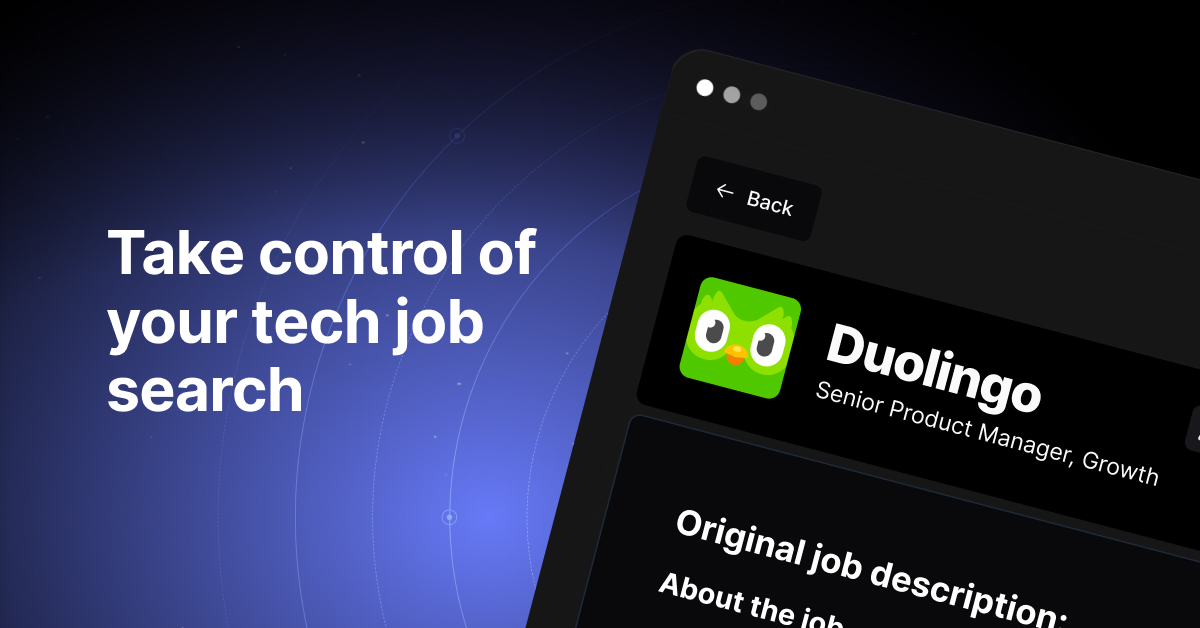