Instantiate and Present a viewController in Swift
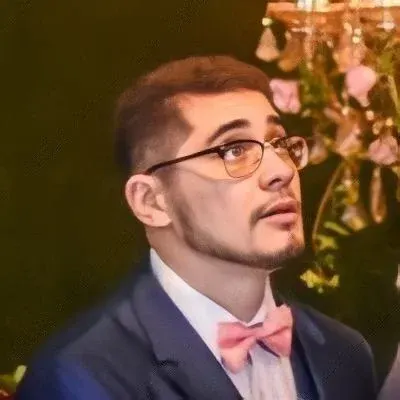
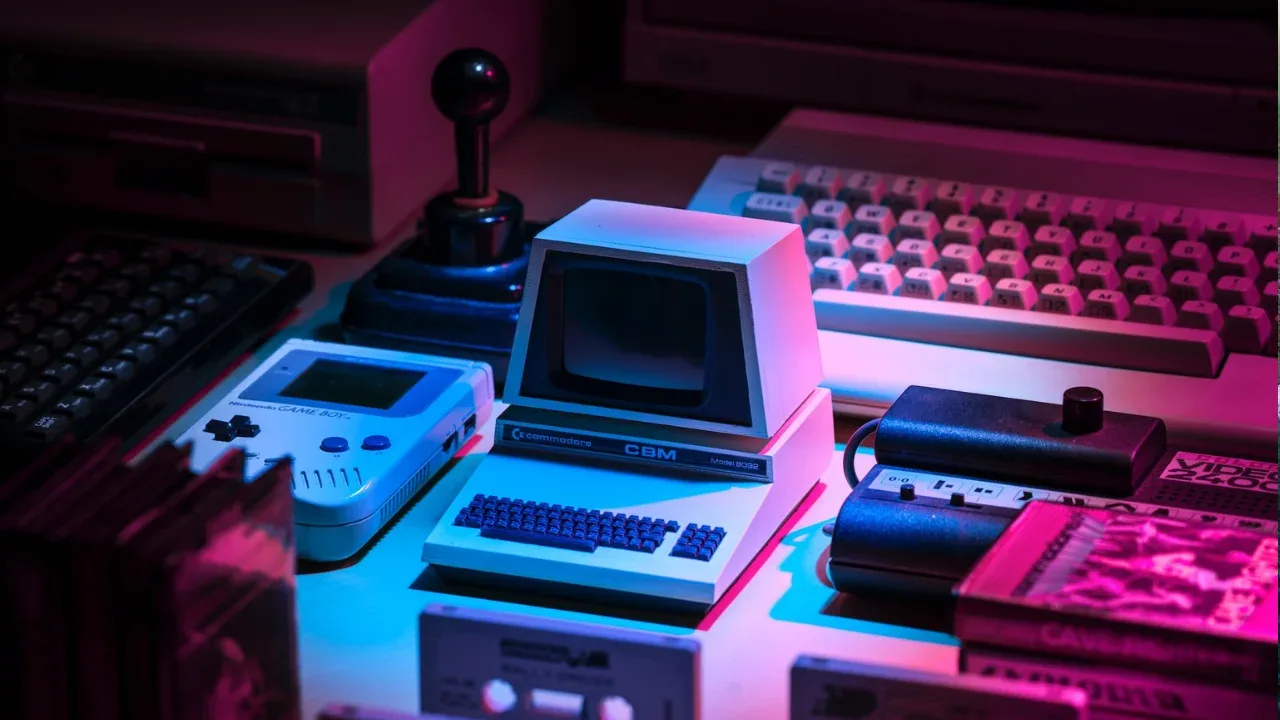
Instantiating and Presenting a viewController in Swift 🚀
So, you're diving into the world of Swift and encountered a little bump when it comes to instantiating and presenting a viewController from a specific UIStoryboard. Fear not! I'm here to guide you through this process with simple and easy solutions. 🎉
The Issue
Let's start by understanding the problem you faced. In Objective-C, you would typically do the following to instantiate and present a viewController:
UIStoryboard *storyboard = [UIStoryboard storyboardWithName:@"StoryboardName" bundle:nil];
UIViewController *viewController = [storyboard instantiateViewControllerWithIdentifier:@"ViewControllerID"];
[self presentViewController:viewController animated:YES completion:nil];
However, when you transition to Swift, the syntax and approach differ slightly. That's where I come in to help! 😎
The Solution
To achieve the same result in Swift, follow the steps outlined below:
Obtain a reference to the desired UIStoryboard:
let storyboard = UIStoryboard(name: "StoryboardName", bundle: nil)
Make sure to replace "StoryboardName" with the actual name of your storyboard file.
Instantiate the desired viewController using its identifier:
let viewController = storyboard.instantiateViewController(withIdentifier: "ViewControllerID")
Replace "ViewControllerID" with the identifier you assigned to your viewController in the storyboard.
Present the viewController on the screen:
self.present(viewController, animated: true, completion: nil)
And voilà! You have successfully instantiated and presented a viewController in Swift. 🎉
Example
Here's a complete example to illustrate the process:
let storyboard = UIStoryboard(name: "StoryboardName", bundle: nil)
let viewController = storyboard.instantiateViewController(withIdentifier: "ViewControllerID")
self.present(viewController, animated: true, completion: nil)
Remember to replace "StoryboardName" with the actual name of your storyboard file and "ViewControllerID" with the identifier of your desired viewController.
Conclusion and Call-to-Action
Congratulations! You have learned how to instantiate and present a viewController from a specific UIStoryboard in Swift. 🎉
Now, go ahead and try implementing this solution in your project. If you encounter any difficulties or have further questions, feel free to leave a comment below. Let's engage in a conversation and find the best possible solution together! 💬
Happy coding! ✨🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
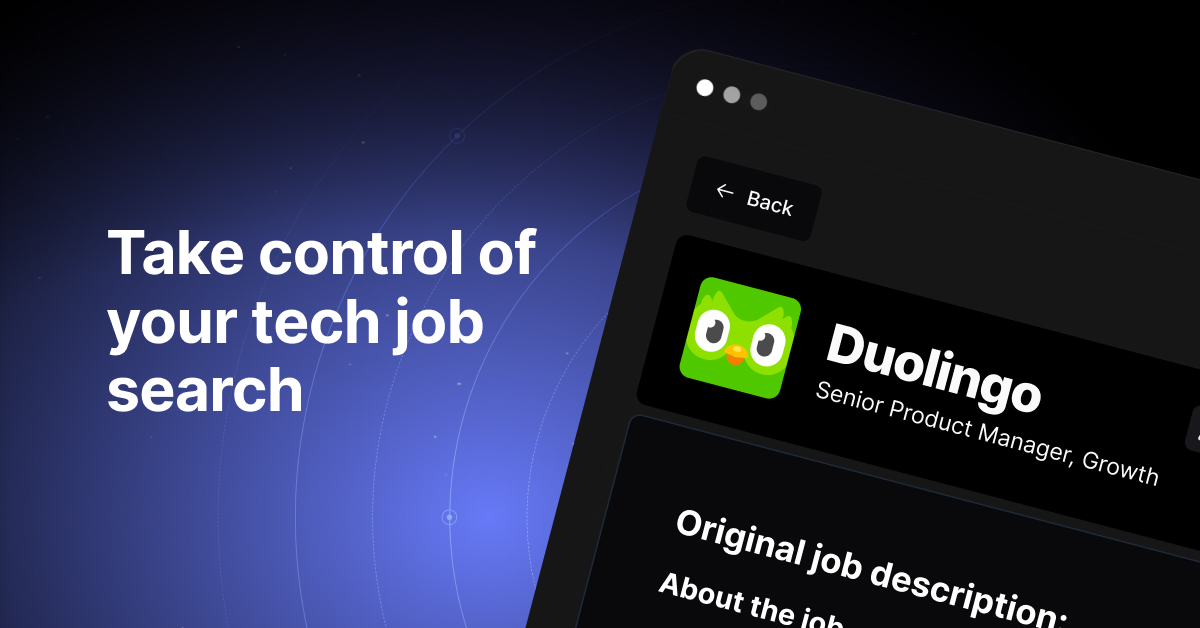