How to use pull to refresh in Swift?
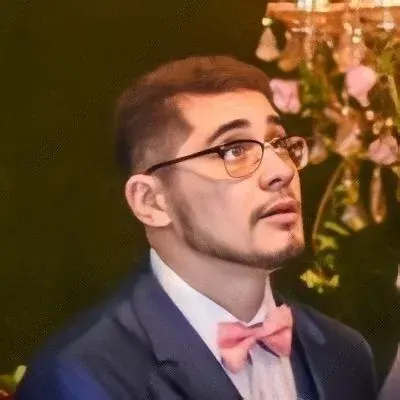
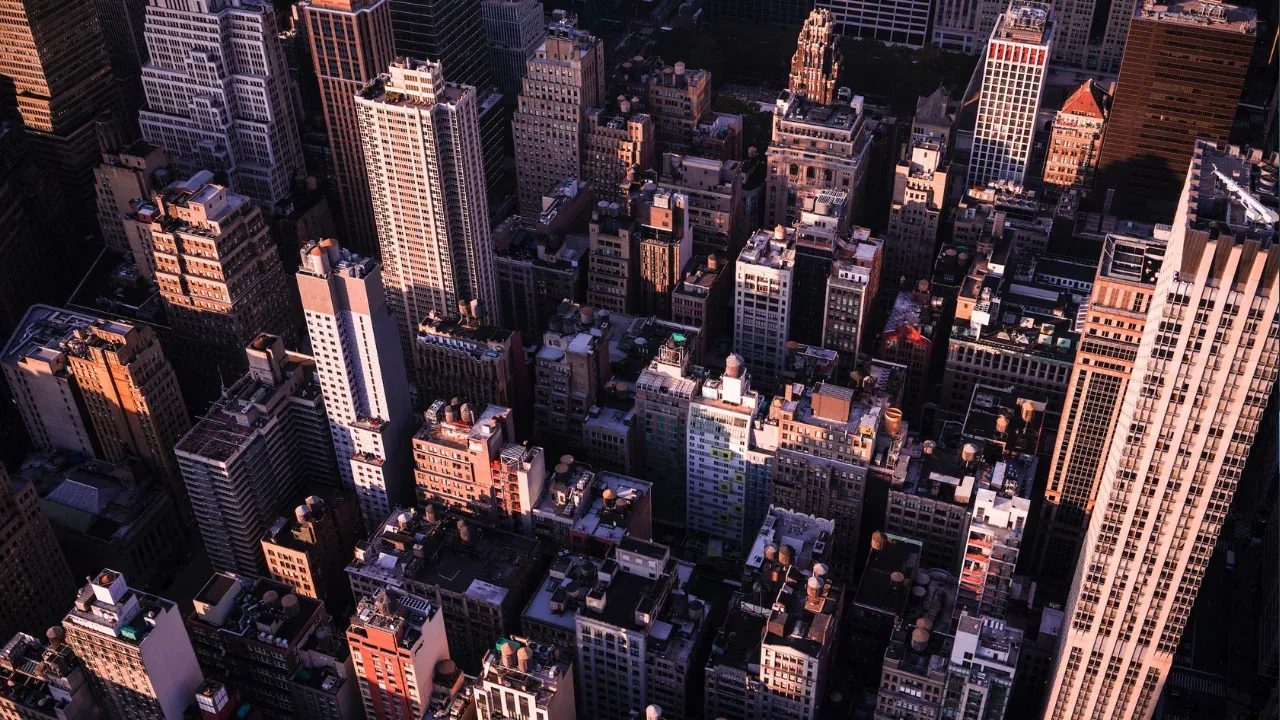
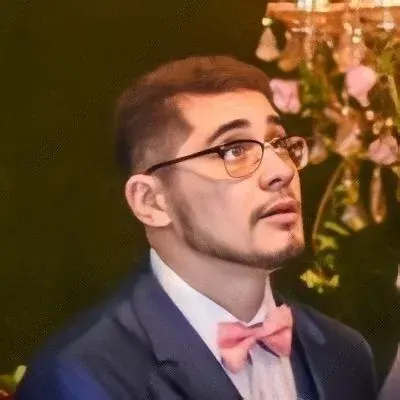
How to Use Pull-to-Refresh in Swift: A Guide for Swift Developers
Have you ever used an app that allows you to refresh the content by simply pulling down the screen? This gesture, known as "Pull-to-Refresh," provides a convenient way to update data in real-time. In this blog post, we will explore how to implement Pull-to-Refresh functionality in Swift for an RSS reader app. 📲💨
The Issue: Property 'self.refresh' Not Initialized
Before we dive into the implementation details, let's address the issue raised by our reader in the provided code snippet. The error message "Property 'self.refresh' not initialized at super.init call" occurs when a property is not properly initialized before it is used. In our case, the 'refresh' property, which represents the UIScreenEdgePanGestureRecognizer, needs to be properly initialized. 🚫💥
To resolve this issue, we need to add the initialization code for the 'refresh' property. Modify the code as shown below: 🛠️
<pre><code>@IBOutlet var refresh: UIScreenEdgePanGestureRecognizer = UIScreenEdgePanGestureRecognizer()</code></pre>
By initializing the 'refresh' property, we ensure that it is properly instantiated and ready to be used. With this modification, the error message should no longer occur.
Implementing Pull-to-Refresh Functionality
Now that we have resolved the initialization issue, let's focus on how to incorporate the Pull-to-Refresh functionality into our RSS reader app. The basic idea behind this feature is to refresh the content whenever the user pulls down the screen.
To achieve this, we'll utilize a UITableViewController and add the necessary code to enable the Pull-to-Refresh gesture. Follow the steps below: 🔄✨
Make sure you have imported the necessary frameworks at the top of your Swift file:
import UIKit
In your UITableViewController subclass, declare a UIRefreshControl property:
let refreshControl = UIRefreshControl()
This UIRefreshControl will handle the Pull-to-Refresh functionality.
In the viewDidLoad() method of your UITableViewController subclass, add the following code:
override func viewDidLoad() { super.viewDidLoad() // Set the Pull-to-Refresh control as the refreshControl of the table view tableView.refreshControl = refreshControl // Add a target-action pair to handle the refresh event refreshControl.addTarget(self, action: #selector(handleRefresh), for: .valueChanged) }
In this code snippet, we set the refreshControl property of the tableView to the UIRefreshControl we created earlier. We also assign a target-action pair to handle the refresh event.
Implement the handleRefresh() method:
@objc private func handleRefresh() { // Perform the necessary data update here // For example, you could fetch new RSS feeds or reload existing data // Once the update is complete, end the refreshing animation refreshControl.endRefreshing() }
In this method, you can update the data of your RSS reader app, such as fetching new RSS feeds or reloading the existing data. Once the update is complete, make sure to end the refreshing animation by calling refreshControl.endRefreshing().
That's it! You have successfully implemented the Pull-to-Refresh functionality in your RSS reader app. 🎉🔃
Now, whenever the user pulls down the screen, the handleRefresh() method will be triggered, allowing you to update the content in real-time.
Conclusion and Call-to-Action
Implementing Pull-to-Refresh functionality in Swift can greatly enhance the user experience of your app. By allowing users to refresh content with a simple gesture, you can provide them with the most up-to-date information effortlessly.
Remember, when encountering issues like the one we addressed in this blog post, the key is to understand the error message and find the appropriate solution. By following the steps outlined in this guide, you can successfully implement Pull-to-Refresh in your Swift apps.
So go ahead and try adding Pull-to-Refresh to your RSS reader app! Let us know in the comments section how it goes, and feel free to ask any questions or share your experiences. Happy coding! 🚀💻