How to use NSURLConnection to connect with SSL for an untrusted cert?
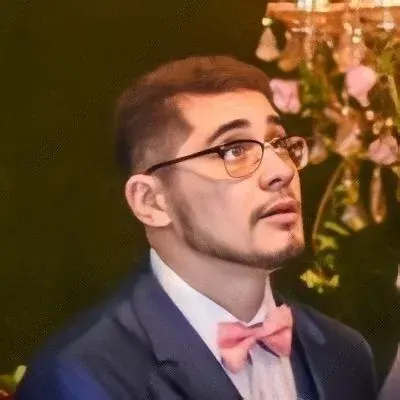
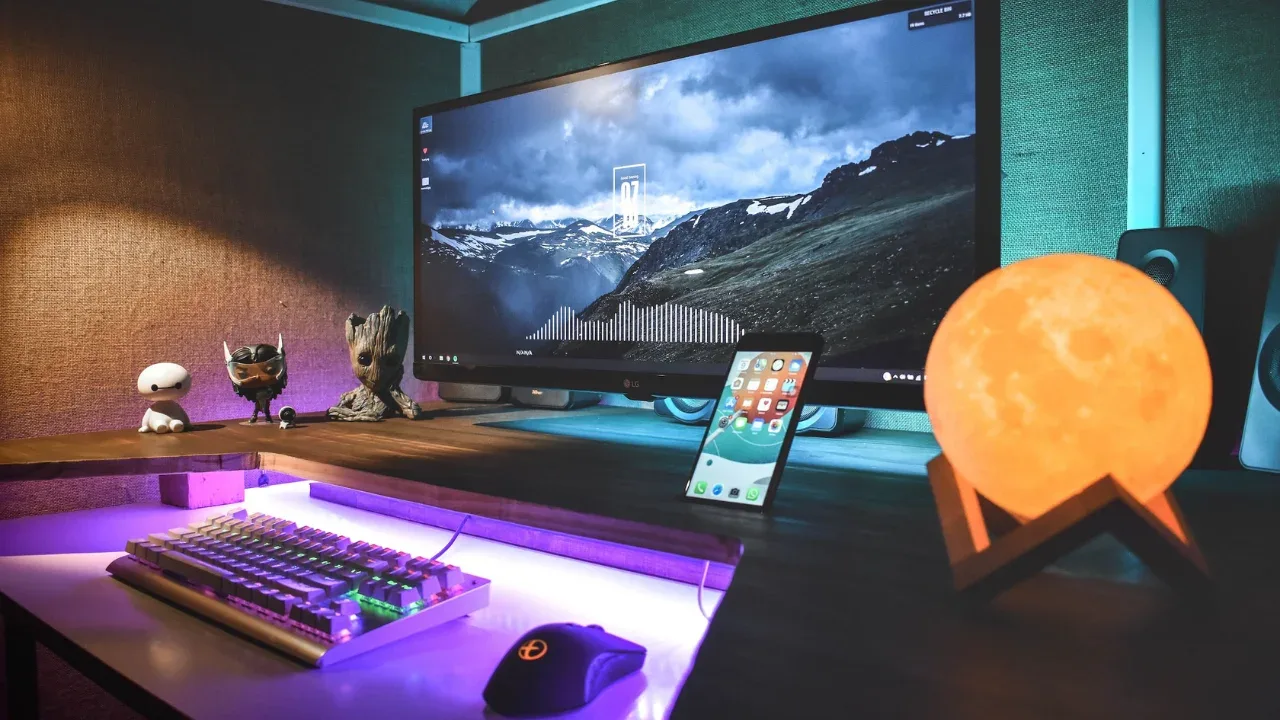
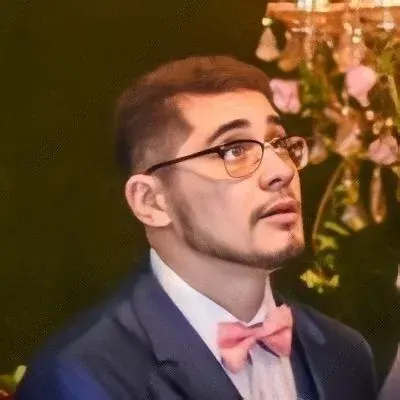
📝🔐 How to Use NSURLConnection to Connect with SSL for an Untrusted Cert
If you're an iOS developer working with NSURLConnection to establish SSL connections, you may have encountered an issue when it comes to self-signed certificates. The error message "untrusted server certificate" can be frustrating, but don't worry! In this guide, we'll show you how to handle this situation and still establish a secure connection.
🔍 Understanding the Problem The code snippet provided in the context is a simple way to establish a connection with a secure webpage. However, it doesn't handle situations where the server certificate is self-signed or untrusted. By default, NSURLConnection performs strict certificate validation, resulting in the error message mentioned.
💡 Finding a Solution To bypass this issue and accept untrusted certificates, you can use the NSURLConnectionDelegate methods. Let's look at the steps involved:
Step 1: Set the Delegate First, set the delegate for your NSURLConnection instance. You'll need to conform to the NSURLConnectionDelegate protocol and implement its methods.
Step 2: Implement Connection Authentication
In your delegate's implementation, you'll specifically handle the authentication challenge that occurs with an untrusted certificate. Use the following connection:canAuthenticateAgainstProtectionSpace:
method to return YES
:
- (BOOL)connection:(NSURLConnection *)connection canAuthenticateAgainstProtectionSpace:(NSURLProtectionSpace *)protectionSpace {
return [protectionSpace.authenticationMethod isEqualToString:NSURLAuthenticationMethodServerTrust];
}
Step 3: Handle Trust Evaluation
Next, implement the connection:didReceiveAuthenticationChallenge:
method to handle the trust evaluation:
- (void)connection:(NSURLConnection *)connection didReceiveAuthenticationChallenge:(NSURLAuthenticationChallenge *)challenge {
if ([challenge.protectionSpace.authenticationMethod isEqualToString:NSURLAuthenticationMethodServerTrust]) {
[challenge.sender useCredential:[NSURLCredential credentialForTrust:challenge.protectionSpace.serverTrust] forAuthenticationChallenge:challenge];
}
[challenge.sender continueWithoutCredentialForAuthenticationChallenge:challenge];
}
This code snippet uses a credential with the server trust obtained from the authentication challenge. By continuing without a credential after that, you override the strict certificate validation and accept untrusted certificates.
Step 4: Create and Start the Connection
Now that your delegate is set up, create your NSMutableURLRequest
and an instance of NSURLConnection
. Assign your delegate to the connection and start it:
NSMutableURLRequest *urlRequest = [NSMutableURLRequest requestWithURL:url];
NSURLConnection *connection = [[NSURLConnection alloc] initWithRequest:urlRequest delegate:self];
[connection start];
✅ The Result By following these steps, you should be able to establish a connection using NSURLConnection, even with an untrusted certificate. This technique is handy for testing purposes or when interacting with servers using self-signed certificates in specific scenarios.
🔔 Your Turn! We hope this guide helped you solve the "untrusted server certificate" issue while using NSURLConnection. Now it's your turn! Try implementing this solution in your project and let us know how it works for you. Have you encountered any other challenges related to SSL connections in iOS development? Share your experiences in the comments below! Let's learn from each other and make secure connections a breeze! 👍
➡️ And don't forget to share this article with your fellow iOS developers who might be facing a similar issue. Sharing is caring! 😊