How to use hex color values
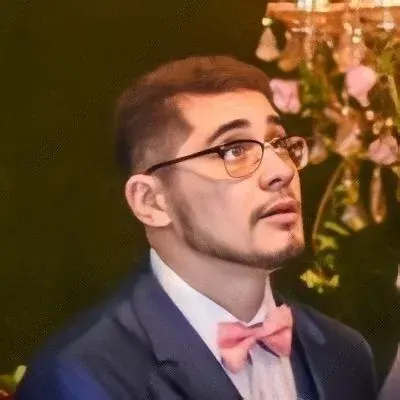
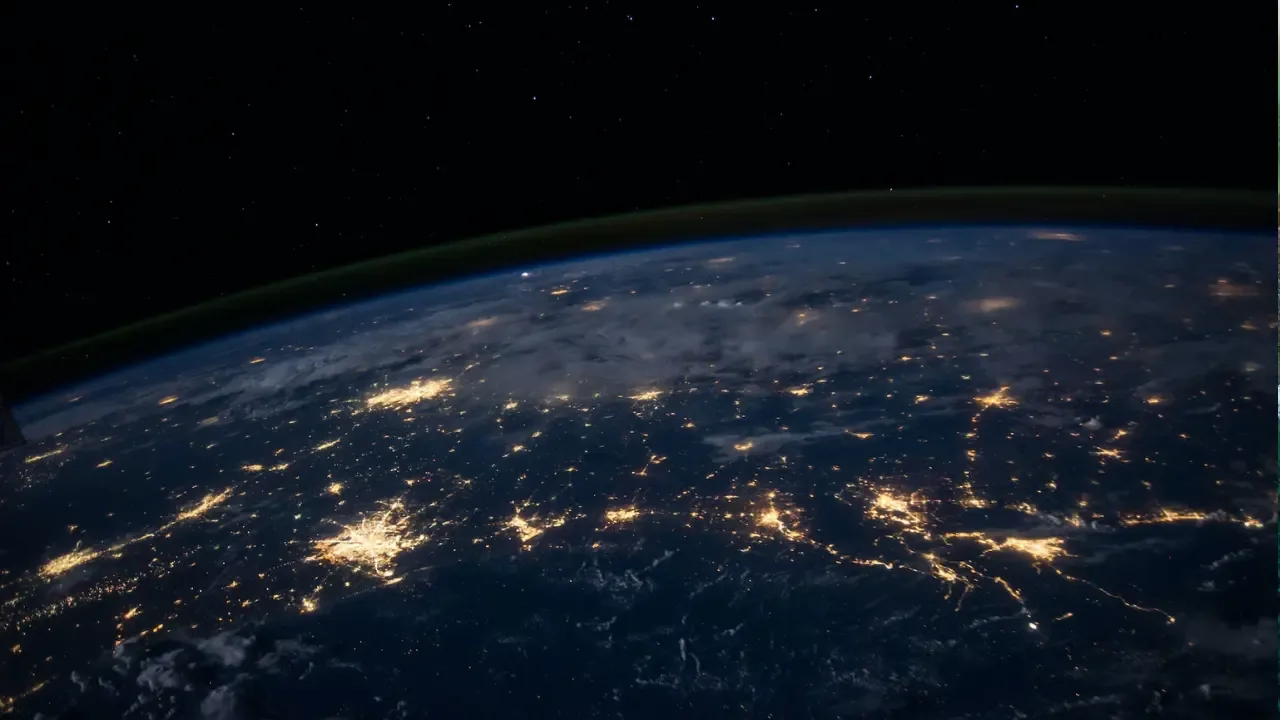
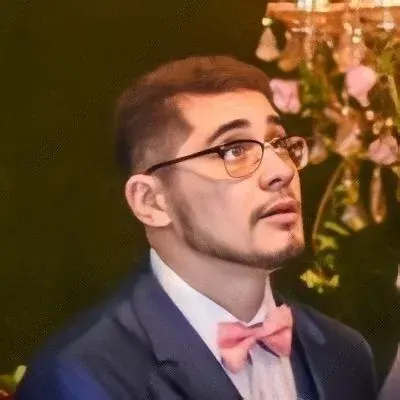
Easy Guide on Using Hex Color Values in Swift 😎💻🎨
So, you're tired of sticking to the limited set of colors that UIColor
offers, and you want to venture into the world of hex color values. Well, my tech-savvy friend, you've come to the right place! In this blog post, we'll walk you through how to use hex color values in Swift, and you'll be on your way to creating beautiful, custom color palettes in no time! 🌈
What is a Hex Color Value? 🎨🔢
Before we dive into the implementation, let's discuss what a hex color value is. Hexadecimal color codes are six-digit codes that represent different colors. Each digit can range from 0 to 9, or from A to F, and each pair of digits represents the intensity of red, green, and blue (RGB) colors respectively. For example, the hex code #ffffff
represents pure white, while #000000
represents black.
The Step-by-Step Guide 🚀📝
Now, let's get our hands dirty and walk through the process of using hex color values in Swift:
Create a UIColor extension:
extension UIColor {
}
Add a convenience initializer:
extension UIColor {
convenience init(hex: String) {
}
}
Parse the hex string:
extension UIColor {
convenience init(hex: String) {
var hexSanitized = hex.trimmingCharacters(in: CharacterSet.alphanumerics.inverted)
var rgbValue: UInt64 = 0
Scanner(string: hexSanitized).scanHexInt64(&rgbValue)
}
}
Create a UIColor object:
extension UIColor {
convenience init(hex: String) {
var hexSanitized = hex.trimmingCharacters(in: CharacterSet.alphanumerics.inverted)
var rgbValue: UInt64 = 0
Scanner(string: hexSanitized).scanHexInt64(&rgbValue)
let red = (rgbValue & 0xFF0000) >> 16
let green = (rgbValue & 0x00FF00) >> 8
let blue = rgbValue & 0x0000FF
self.init(
red: CGFloat(red) / 255.0,
green: CGFloat(green) / 255.0,
blue: CGFloat(blue) / 255.0,
alpha: 1.0
)
}
}
Use the hex color value:
let customColor = UIColor(hex: "#ffffff")
And there you have it! You've successfully used a hex color value in Swift! You can replace #ffffff
with any other hex color code to create the desired color. Feel free to experiment with different combinations and create your unique color palettes! 🎉
Troubleshooting Common Issues ⚠️🛠️
Sometimes, you might encounter a few bumps along the way. Here are a couple of common issues and their solutions:
Issue: Invalid Hex Color Value
If you pass an invalid hex color value to the UIColor
initializer, it will result in an unexpected color or a crash.
Solution: Ensure that the hex color value is valid and follows the six-digit format.
Issue: Incorrect RGB Mapping
If the colors are not mapped correctly, it might be due to incorrectly shifting the bits.
Solution: Double-check the bit shifts in the code and ensure they match the RGB positions.
If you encounter any other issues, don't hesitate to reach out to the developer community for assistance. We're all here to help each other! 💪🌍
Conclusion and Call-to-Action 📝🤝
Congratulations on unlocking the power of hex color values in Swift! You now have the freedom to explore an infinite range of colors. 🌈✨
Try implementing hex color values in your next project and see the difference it makes in your visual design. If you found this blog post helpful, share it with your fellow developers to spread the knowledge! Let's all paint our apps with vibrant colors and make the world a brighter place! 💖📱
Feel free to leave a comment below if you have any questions or want to share your own experiences using hex color values. Happy coding! 🚀💻