How to store custom objects in NSUserDefaults
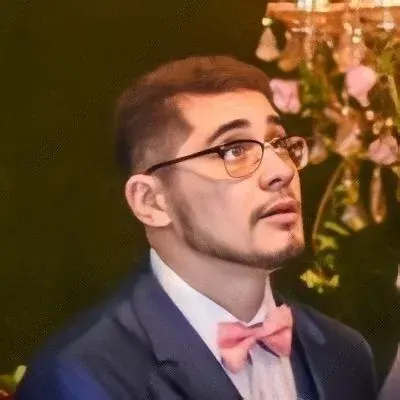
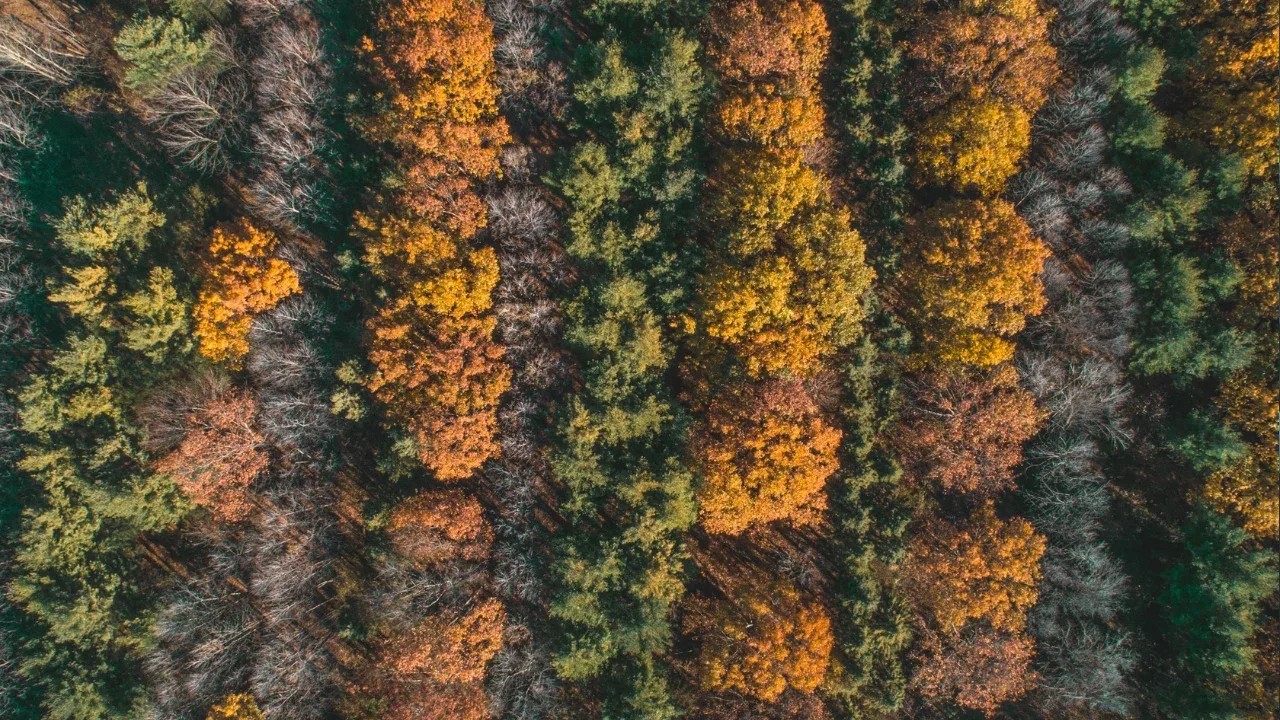
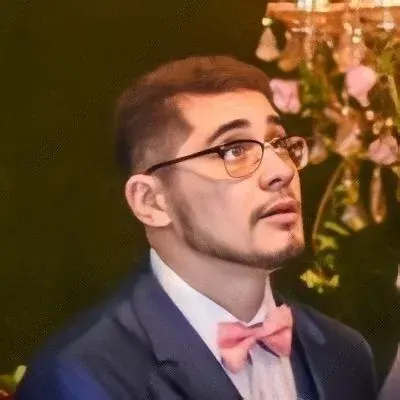
How to store custom objects in NSUserDefaults ๐๐
So you have a custom class in your iOS app and you want to store instances of it in NSUserDefaults, but you're getting an error and don't know how to fix it. Don't worry, we've got you covered! ๐
The Error Message ๐ซโ
The error message you're seeing:
-[NSUserDefaults setObject:forKey:]: Attempt to insert non-property value '<Player: 0x3b0cc90>' of class 'Player'.
This error occurs because NSUserDefaults can only store certain types of information, such as strings, numbers, and data objects. It doesn't directly support storing custom objects. ๐ โโ๏ธ
The Solution ๐กโ
To store custom objects in NSUserDefaults, you need to "encode" your objects before putting them in, and then "decode" them when retrieving them. Here's how you can do it:
Update your custom class to conform to the
NSCoding
protocol. This requires implementing two methods:encodeWithCoder:
to encode your object, andinitWithCoder:
to decode it. Here's an example implementation for yourPlayer
class:@interface Player : NSObject <NSCoding> // properties and methods @end
@implementation Player // property declarations - (void)encodeWithCoder:(NSCoder *)encoder { [encoder encodeObject:self.name forKey:@"name"]; [encoder encodeObject:self.life forKey:@"life"]; } - (id)initWithCoder:(NSCoder *)decoder { self = [super init]; if (self) { self.name = [decoder decodeObjectForKey:@"name"]; self.life = [decoder decodeObjectForKey:@"life"]; } return self; } // other methods and deallocation @end
In your AppDelegate (or wherever you need to store the custom objects), add two methods โ
saveCustomObject:
to store the object in NSUserDefaults, andloadCustomObjectWithKey:
to retrieve it. Here's an example implementation:- (void)saveCustomObject:(Player *)object { NSUserDefaults *defaults = [NSUserDefaults standardUserDefaults]; NSData *encodedObject = [NSKeyedArchiver archivedDataWithRootObject:object]; [defaults setObject:encodedObject forKey:@"customObjectKey"]; [defaults synchronize]; } - (Player *)loadCustomObjectWithKey:(NSString *)key { NSUserDefaults *defaults = [NSUserDefaults standardUserDefaults]; NSData *encodedObject = [defaults objectForKey:key]; Player *object = [NSKeyedUnarchiver unarchiveObjectWithData:encodedObject]; return object; }
When you need to save a custom object, call
saveCustomObject:
and pass the object you want to store. For example:Player *player = [[Player alloc] init]; player.name = @"John"; player.life = 20; [self saveCustomObject:player];
When you need to retrieve the custom object, call
loadCustomObjectWithKey:
and pass the corresponding key. For example:Player *player = [self loadCustomObjectWithKey:@"customObjectKey"]; NSLog(@"Name: %@, Life: %d", player.name, player.life);
That's it! Now you should be able to store and retrieve custom objects in NSUserDefaults without any errors. ๐๐
Help, It's Still Not Working! ๐ซ๐ง
If you're still facing issues, like crashes or unexpected behavior, here are a few things to check:
Make sure you've properly implemented the
encodeWithCoder:
andinitWithCoder:
methods in your custom class.Check if you're using the correct keys when encoding and decoding the objects.
Make sure you're calling the
saveCustomObject:
andloadCustomObjectWithKey:
methods at the right times and in the correct places.
If all else fails, feel free to reach out to us for further assistance. We're here to help! ๐ค๐ง
Conclusion and Call-to-action ๐๐ฃ
Storing custom objects in NSUserDefaults is possible, but it requires some additional steps. By properly encoding and decoding your custom objects, you can easily store them in NSUserDefaults and retrieve them whenever needed.
Try implementing the solutions mentioned in this article and let us know how it worked for you. If you have any questions or need further assistance, leave a comment below or contact us directly. And don't forget to share this article with your fellow developers who might find it helpful! ๐๐ป
Happy coding! ๐ช๐ฉโ๐ป๐จโ๐ป