How to segue programmatically in iOS using Swift
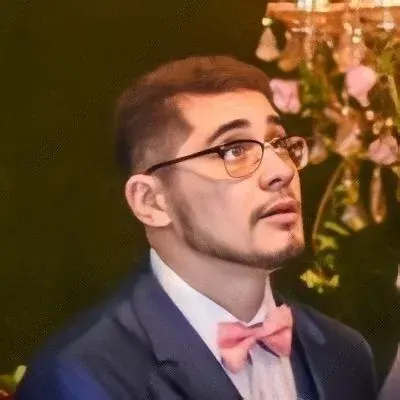
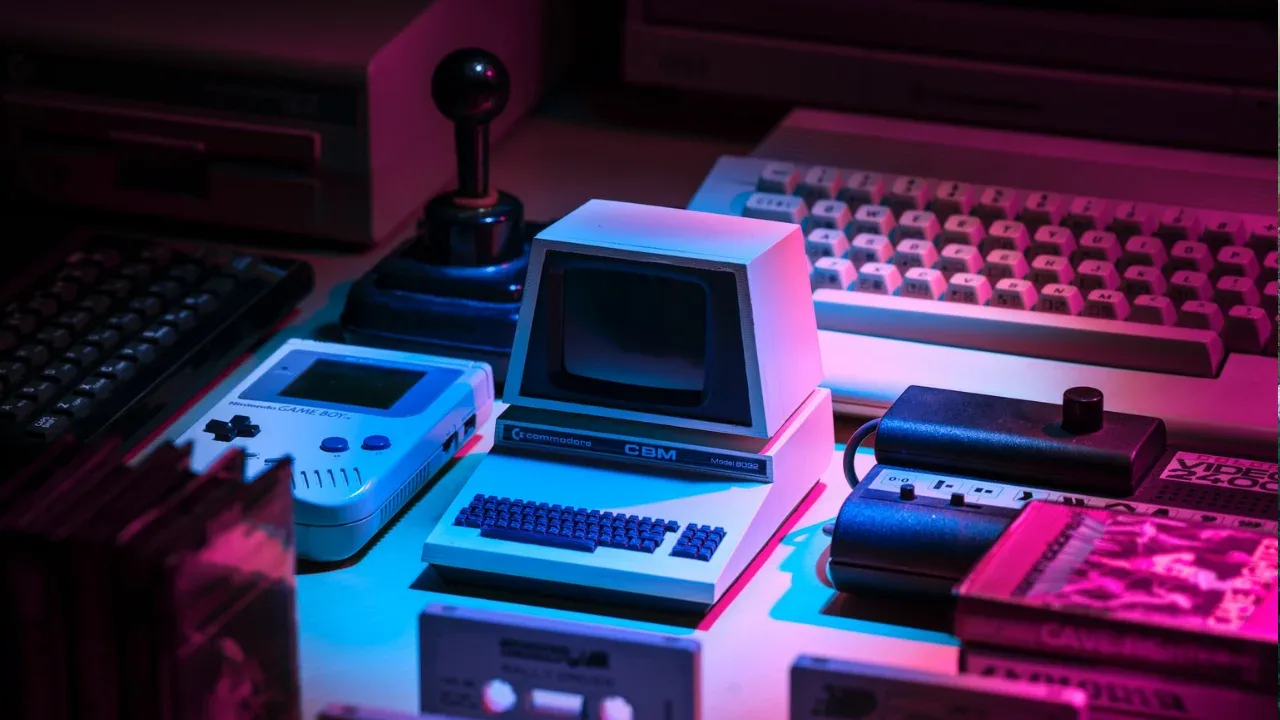
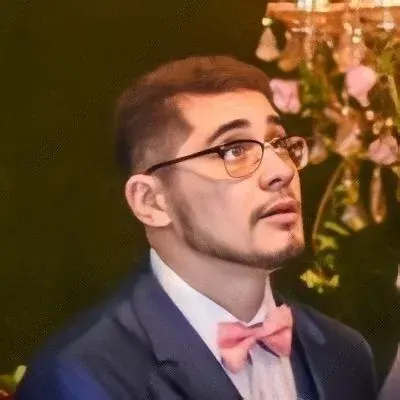
How to Segue Programmatically in iOS Using Swift 🚀
If you're working on an iOS app and have been wondering how to segue programmatically using Swift, you've come to the right place! 🎉 In this blog post, we'll dive into common issues and provide easy solutions so that you can seamlessly transition between views in your app. Let's get started! 💪
The Context 👀
Imagine you're building an app that uses the Facebook SDK to authenticate users. You've successfully written code to handle session state changes in a separate class called FBManager
. Here's a simplified version of your code:
import Foundation
class FBManager {
class func fbSessionStateChange(fbSession: FBSession!, fbSessionState: FBSessionState, error: NSError?) {
//.. handle all session states
FBRequestConnection.startForMeWithCompletionHandler {
(conn: FBRequestConnection!, result: AnyObject!, error: NSError!) -> Void in
println("Logged in user: \n\(result)")
let storyboard = UIStoryboard(name: "Main", bundle: NSBundle.mainBundle())
let loggedInView: UserViewController = storyboard.instantiateViewControllerWithIdentifier("loggedInView") as UserViewController
loggedInView.result = result
//todo: segue to the next view???
}
}
}
The Question ❓
You've successfully obtained the user's data, and now you want to segue to the next view from within your FBManager
class. But how do you achieve this when you're not in a view controller? 🤔
The Solution 💡
To perform a segue from a class that isn't a view controller, you'll need to access the current view controller and trigger the segue programmatically. Here's how you can do it:
Import the UIKit framework in your
FBManager
class to access its classes.
import Foundation
import UIKit
Create a
UIWindow
extension or utility function to find the current view controller. You can add the following extension to your code:
extension UIWindow {
func getCurrentViewController() -> UIViewController? {
if let rootViewController = self.rootViewController {
var currentViewController: UIViewController? = rootViewController
while let presentedViewController = currentViewController?.presentedViewController {
currentViewController = presentedViewController
}
return currentViewController
}
return nil
}
}
Use the
getCurrentViewController()
function to get the current view controller and perform the segue programmatically. Replace the//todo: segue to the next view???
line with the following code:
if let currentViewController = UIApplication.sharedApplication().keyWindow?.getCurrentViewController() {
currentViewController.performSegueWithIdentifier("yourSegueIdentifier", sender: nil)
}
Make sure to replace "yourSegueIdentifier"
with the actual identifier of the segue you've set up in your storyboard.
Time to Shine 💫
You're all set! With just a few lines of code, you can now segue programmatically from within your FBManager
class. 🎉
Remember, don't be afraid to experiment and explore different possibilities. Adding the ability to programmatically segue is a powerful tool that can enhance the user experience of your app.
Conclusion 🌟
In this blog post, we've learned how to segue programmatically in iOS using Swift. By accessing the current view controller and triggering the segue, we can seamlessly transition between views, even from a class that isn't a view controller. 💪
We hope this guide has been helpful to you! If you have any questions or need further assistance, please don't hesitate to leave a comment below. Happy coding! 😊✨
Did you find this blog post helpful? Share it with your fellow iOS developers and let's make app development even more awesome! 🚀