How to remove empty cells in UITableView?
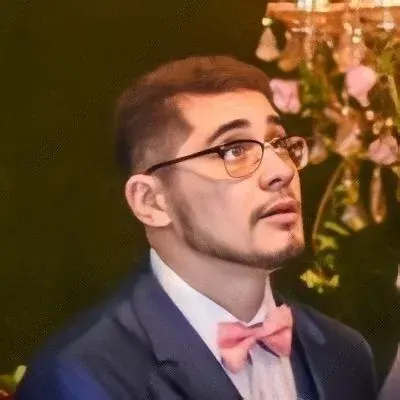
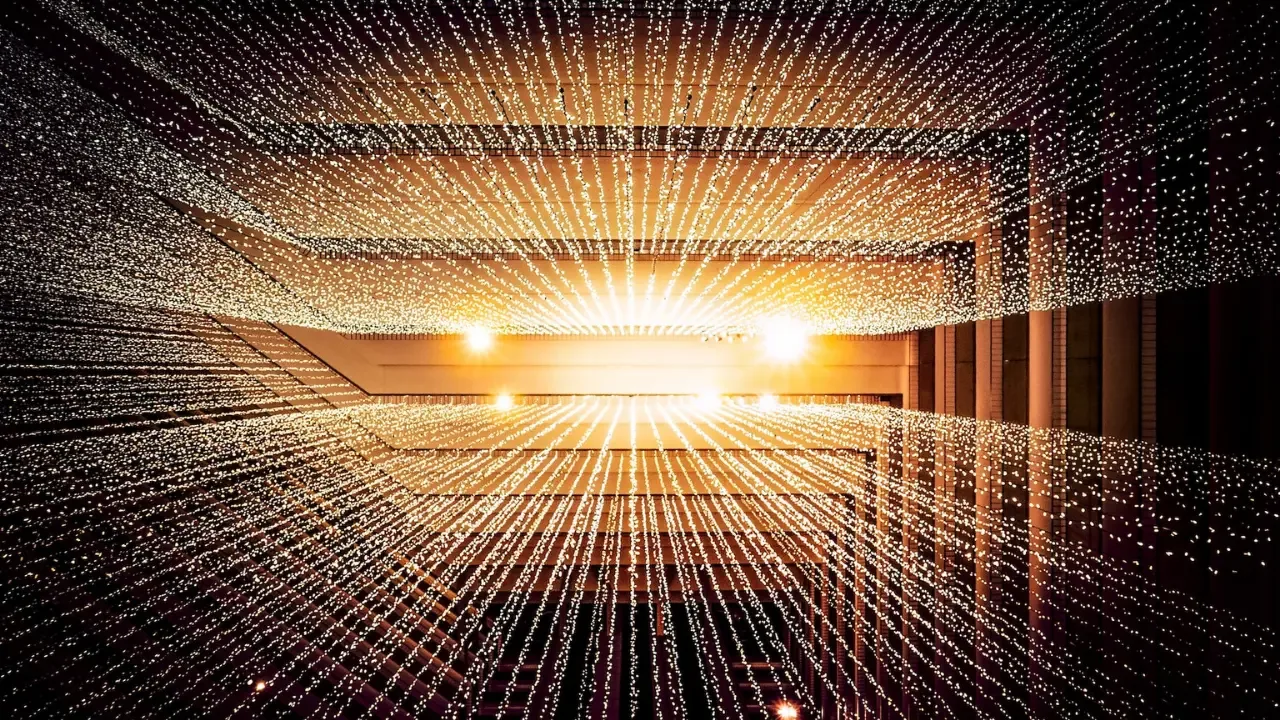
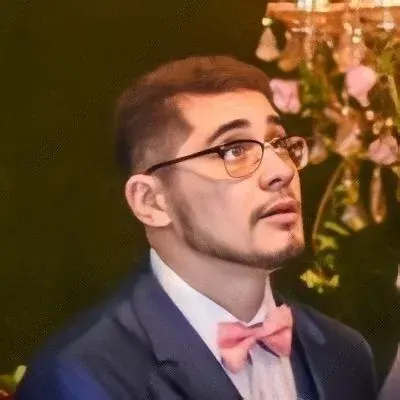
Remove Empty Cells in UITableView: A Comprehensive Guide 👀💪
Introduction 📖
Have you ever encountered empty cells at the end of your UITableView and wondered how to remove them? 🤔 Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide easy solutions for you to implement. Let's dive in! 🚀
The Problem Statement 🤷♀️
Our reader, who wants to display a simple UITableView with some data, has run into a predicament. They want to set a static height for the UITableView so that it doesn't display empty cells at the end of the table. They're seeking guidance on how to achieve this. 💡
The Code:
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
NSLog(@"%d", [arr count]);
return [arr count];
}
Solution 1: Adjust UITableView's Frame 📏
One way to remove those pesky empty cells is to adjust the UITableView's frame. By setting the frame's height to fit exactly the displayed cells, we eliminate any empty cells at the end. 📐
Here's an example of how you can achieve this:
// Get the height of each cell
CGFloat cellHeight = tableView.rowHeight;
// Calculate the total height of all cells
CGFloat totalHeight = cellHeight * [arr count];
// Create a new frame with an adjusted height
CGRect newFrame = tableView.frame;
newFrame.size.height = totalHeight;
// Set the new frame to the UITableView
tableView.frame = newFrame;
Solution 2: Use UITableView's Footer View 🦶
Another approach is to leverage the UITableView's footer view to hide any additional empty cells. By setting an empty view as the footer, we can effectively remove the display of extra cells. 🦶
Let's see how you can implement this solution:
// Create an empty UIView and set it as the footer view
UIView *footerView = [[UIView alloc] initWithFrame:CGRectZero];
tableView.tableFooterView = footerView;
Conclusion and Call-to-Action ✅🔥
Congratulations! You have successfully learned two easy ways to remove those annoying empty cells in your UITableView. Now go ahead and implement these solutions in your own projects. Say goodbye to empty cells and hello to a clean and polished user interface! 💃
If you found this guide helpful, be sure to share it with your fellow developers! And don't hesitate to leave a comment below if you have any questions or additional tips to share. We'd love to hear from you! 🗣️👇
Happy coding! 💻🎉