How to pass prepareForSegue: an object
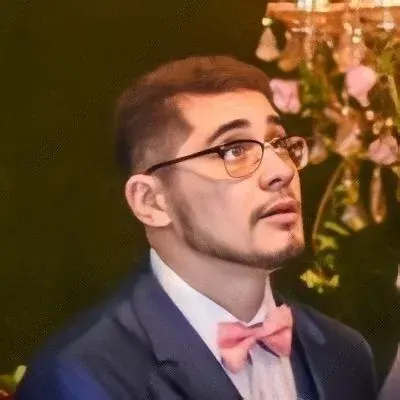
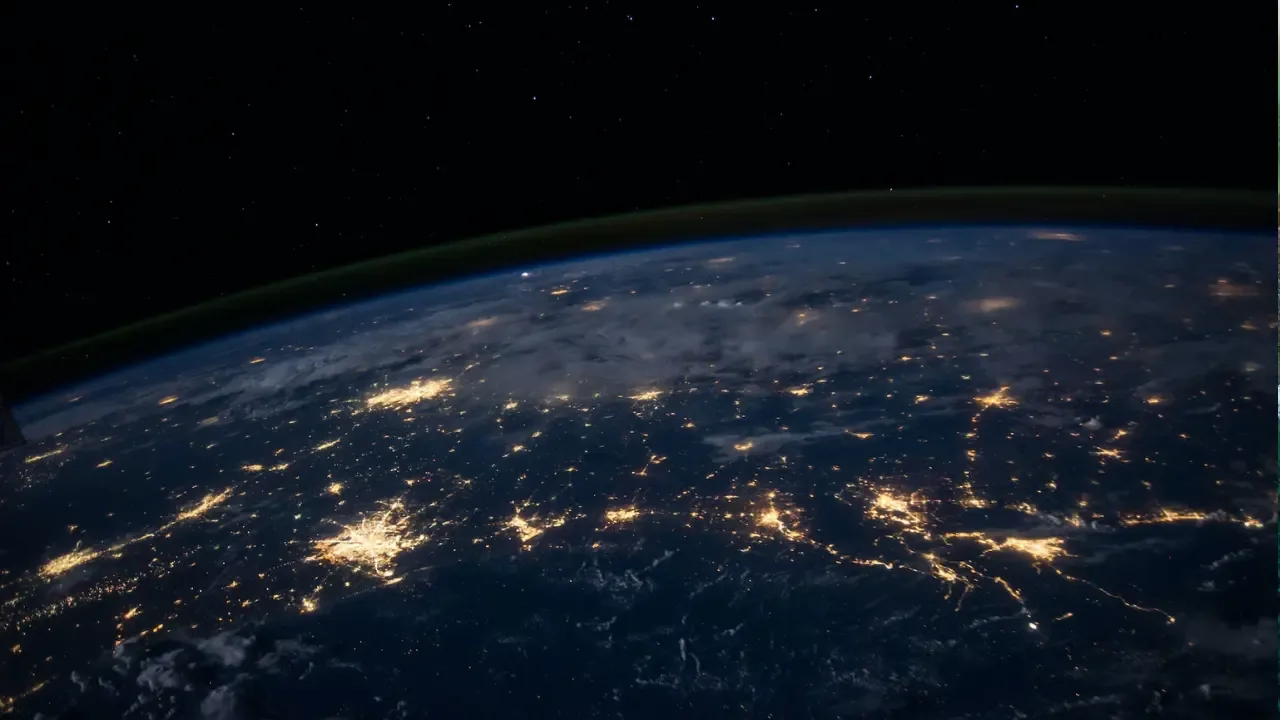
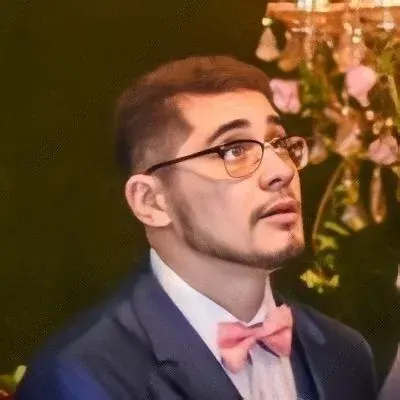
How to Pass an Object in prepareForSegue 💼
So you have a map view with annotations and you want to pass a different object to a table view based on which callout button was clicked. You've already figured out how to detect which button was clicked, but now you're stuck on how to pass that relevant data object using prepareForSegue
. Don't worry, we've got you covered! 👍
The Problem 🤔
When using prepareForSegue
to pass data to a destination view controller, you can't directly pass additional arguments to it. This can make it challenging to pass the relevant data object based on which callout button was clicked.
Solution 1: Using Properties 🏠
One elegant solution is to use properties in your destination view controller. Here's how you can do it:
In the destination view controller, create a property to hold the data object you want to pass. Let's call it
selectedDataObject
.In the source view controller, set the
selectedDataObject
property of the destination view controller based on which callout button was clicked.In the
prepareForSegue
method of the source view controller, assign the destination view controller to a variable, and then set theselectedDataObject
property of the destination view controller.
Let's see how this looks in code:
// DestinationViewController.swift
class DestinationViewController: UIViewController {
var selectedDataObject: DataObject?
// rest of your code
}
// SourceViewController.swift
class SourceViewController: UIViewController {
func didSelectCalloutButton() {
let destinationVC = storyboard?.instantiateViewController(withIdentifier: "DestinationViewController") as! DestinationViewController
destinationVC.selectedDataObject = // set the relevant data object
performSegue(withIdentifier: "YourSegueIdentifier", sender: self)
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "YourSegueIdentifier" {
let destinationVC = segue.destination as! DestinationViewController
destinationVC.selectedDataObject = destinationVC.selectedDataObject
}
}
}
Solution 2: Using a Delegate 👥
Another option is to use a delegate to pass the relevant data object. Here's how you can do it:
Create a protocol in your destination view controller that defines a method for passing the data object. Let's call it
DataObjectDelegate
.Make the source view controller conform to the
DataObjectDelegate
protocol, and implement the method for passing the data object.In the destination view controller, create a delegate property of type
DataObjectDelegate
.Set the delegate property of the destination view controller to the source view controller before triggering the segue.
Call the delegate method in the destination view controller when you want to pass the data object.
Here's a code example to illustrate this approach:
// DestinationViewController.swift
protocol DataObjectDelegate: AnyObject {
func passDataObject(_ dataObject: DataObject)
}
class DestinationViewController: UIViewController {
weak var delegate: DataObjectDelegate?
func didSelectCalloutButton() {
delegate?.passDataObject(/* set the relevant data object */)
performSegue(withIdentifier: "YourSegueIdentifier", sender: self)
}
}
// SourceViewController.swift
class SourceViewController: UIViewController, DataObjectDelegate {
func passDataObject(_ dataObject: DataObject) {
// handle the passed data object
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "YourSegueIdentifier" {
let destinationVC = segue.destination as! DestinationViewController
destinationVC.delegate = self
}
}
}
Conclusion 🎉
Passing an object in prepareForSegue
can be a bit tricky when you need to pass a dynamic data object based on user interactions. However, with these two solutions using properties or a delegate, you can easily achieve the desired effect. So go ahead and implement one of these solutions in your code and make your map view and table view work seamlessly together! Happy coding! 💻🚀
Have any other tips or ideas for passing objects in prepareForSegue
? Share them in the comments below! Let's learn from each other! ✨