How to load local html file into UIWebView
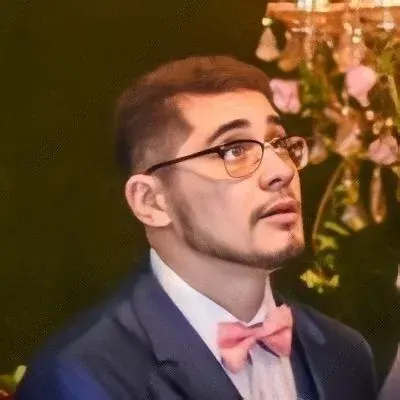
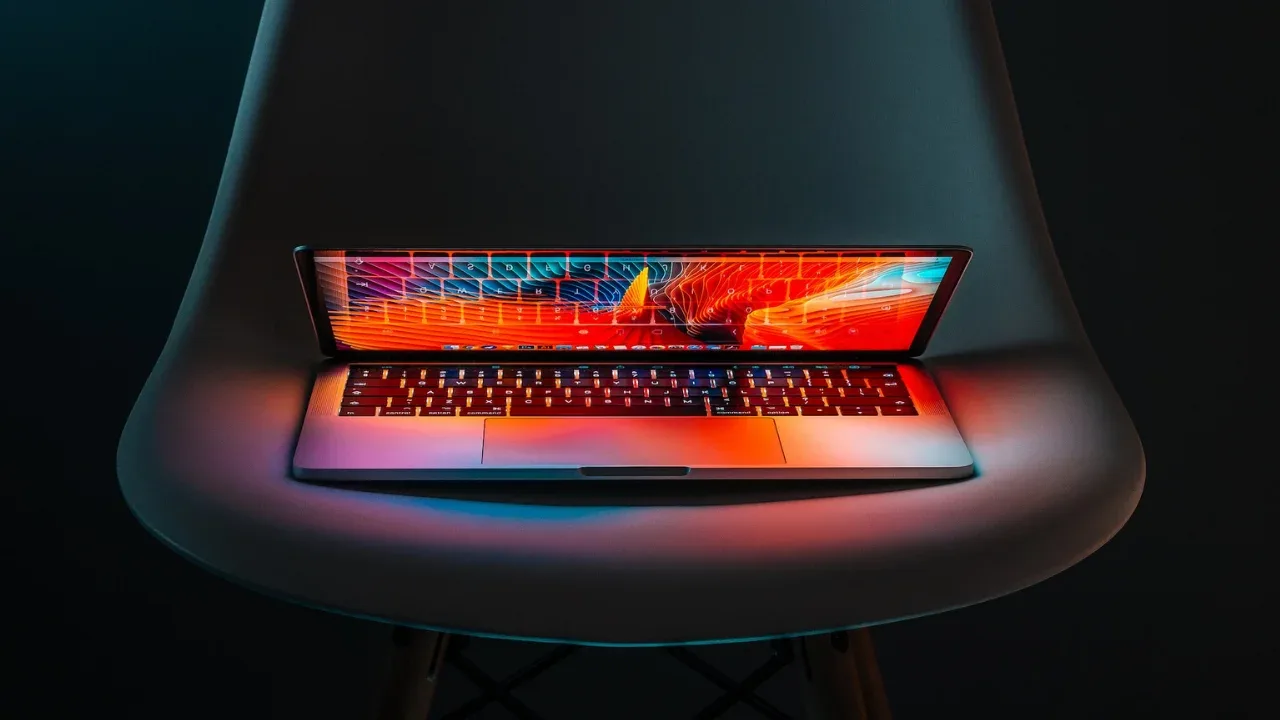
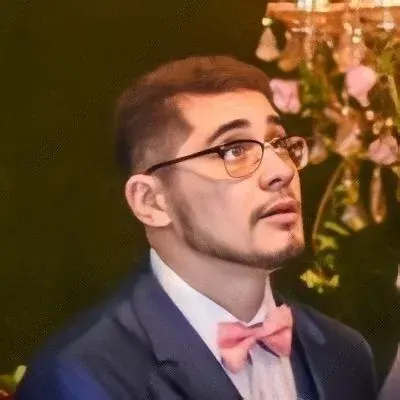
🔍Loading Local HTML File into UIWebView: A Simple Guide🔍
Are you facing trouble loading a local HTML file into your UIWebView? Don't worry, we've got you covered! In this guide, we'll address the common issues you might encounter and provide easy solutions to help you successfully load your HTML file. So, let's dive in! 💻🔌
💡Understanding the Problem
The code snippet you shared seems to be on the right track, but there might be a small oversight causing the UIWebView to stay blank. Let's break down the code and identify the potential issue.
🔎Possible Issue: Resource Path
One common mistake is incorrectly specifying the resource path when loading the HTML file. Let's ensure that the pathForResource method is pointing to the correct directory and HTML file.
NSString *htmlFile = [[NSBundle mainBundle] pathForResource:@"sample" ofType:@"html" inDirectory:@"html_files"];
✅Solution: Double-check Resource Path
Make sure that you have a folder named "html_files" in your project, containing the "sample.html" file. Check the spelling and capitalization of the folder name and HTML file. Keep in mind that both are case-sensitive.
🔎Possible Issue: Data Conversion
Another potential issue lies in how you're converting the HTML file data using NSData's dataWithContentsOfFile method.
NSData *htmlData = [NSData dataWithContentsOfFile:htmlFile];
✅Solution: Adjust Data Conversion
Try updating the data conversion method to treat the HTML file as a plain text file using the stringWithContentsOfFile method from NSString.
NSString *htmlString = [NSString stringWithContentsOfFile:htmlFile encoding:NSUTF8StringEncoding error:nil];
NSData *htmlData = [htmlString dataUsingEncoding:NSUTF8StringEncoding];
⚙️Loading HTML Data into UIWebView
Now that we've addressed potential issues, let's ensure that we load the HTML data into the UIWebView correctly.
[webView loadData:htmlData MIMEType:@"text/html" textEncodingName:@"UTF-8" baseURL:[NSURL URLWithString:@""]];
🔎Possible Issue: Base URL
The baseURL parameter in the loadData method should be set to nil or a valid URL representing the web page's base location. Leaving it empty might cause the UIWebView to display a blank page.
✅Solution: Set Base URL
Set the baseURL parameter to nil, as shown in the updated code below:
[webView loadData:htmlData MIMEType:@"text/html" textEncodingName:@"UTF-8" baseURL:nil];
🚀Ready for Blast Off!
With the adjustments made, your UIWebView should now load the local HTML file successfully. Enjoy the view! 🎉
📣Call-to-Action: Share Your Success Story!
We hope this guide helped you tackle the challenge of loading a local HTML file into your UIWebView. If you found this information valuable, share it with your fellow coders and tech enthusiasts! And don't forget to leave a comment below, sharing your experience or any questions you might have. Let's keep learning together! 🌟💬
Happy coding! 💻😊