How to hide keyboard in swift on pressing return key?
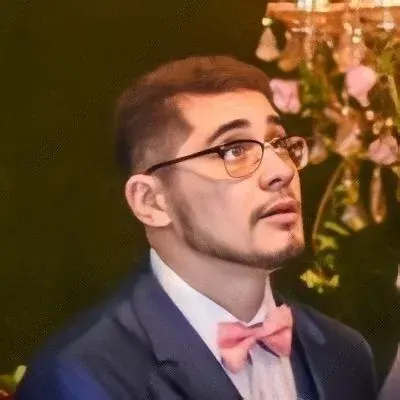
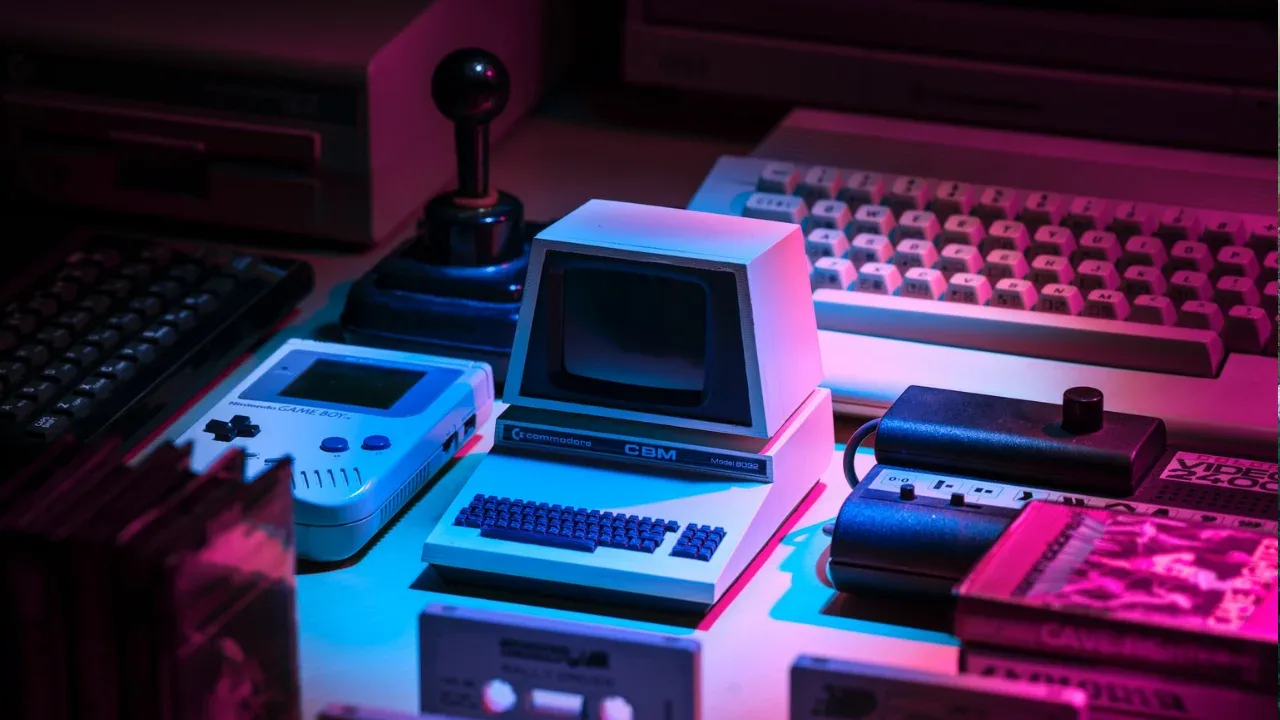
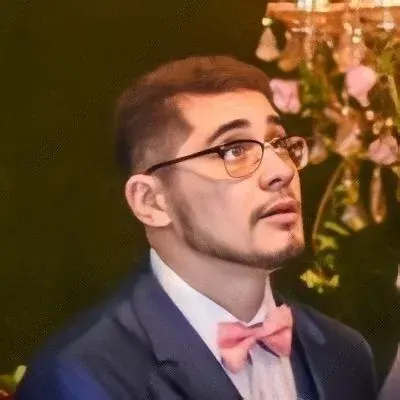
How to Hide Keyboard in Swift on Pressing Return Key?
Are you struggling with hiding the keyboard on pressing the return key in your Swift app? Don't worry, you're not alone! Many developers face this common issue while using UITextField
. But fear not, we've got you covered with some easy solutions. Let's dive in!
The Problem
So, you've implemented the textFieldShouldReturn
method in your code, expecting the keyboard to disappear when the user presses the return key. However, the resignFirstResponder
method is not being called, and the keyboard stubbornly stays on the screen. Frustrating, right?
The Solution
Fear not, my friend! We have a couple of solutions to tackle this problem. Let's discuss them one by one.
Solution 1: Implement UITextFieldDelegate
First things first, ensure that your class adopts the UITextFieldDelegate
. Without this, the textFieldShouldReturn
method won't be triggered. Here's how you should do it:
class YourViewController: UIViewController, UITextFieldDelegate {
// Your code here
}
By adopting the UITextFieldDelegate
, you'll now be able to override the necessary methods.
Solution 2: Set the Delegate
Make sure to set the delegate of your UITextField
to the current class (most likely, your view controller). This connection is essential for the delegate methods to be called. Here's an example:
yourTextField.delegate = self
Solution 3: Resign First Responder
To hide the keyboard when the return key is pressed, you need to call the resignFirstResponder
method on the active text field. Add the following code inside your textFieldShouldReturn
method:
func textFieldShouldReturn(_ textField: UITextField) -> Bool {
textField.resignFirstResponder()
return true
}
By resigning the first responder, you're telling the text field to let go of its focus and pass it on to something else, thus hiding the keyboard.
The Full Code
Here's what your code should look like once you've implemented the above solutions:
class YourViewController: UIViewController, UITextFieldDelegate {
@IBOutlet weak var yourTextField: UITextField!
override func viewDidLoad() {
super.viewDidLoad()
yourTextField.delegate = self
}
func textFieldShouldReturn(_ textField: UITextField) -> Bool {
textField.resignFirstResponder()
return true
}
}
Now, when the user presses the return key, the keyboard will disappear and your UI will be much cleaner.
Conclusion
Congratulations! You've learned how to hide the keyboard in Swift on pressing the return key. By implementing the UITextFieldDelegate
and setting the delegate for your text field, you're now able to call the resignFirstResponder
method and achieve the desired functionality.
If you found this guide helpful and want to learn more Swift tips and tricks, head over to our blog for more exciting content! Let us know in the comments below if you have any questions or other topics you'd like us to cover. Happy coding! 🚀