How to find topmost view controller on iOS
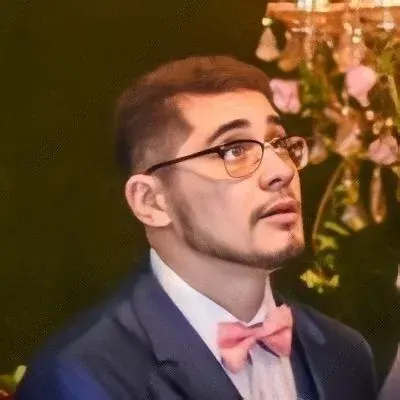
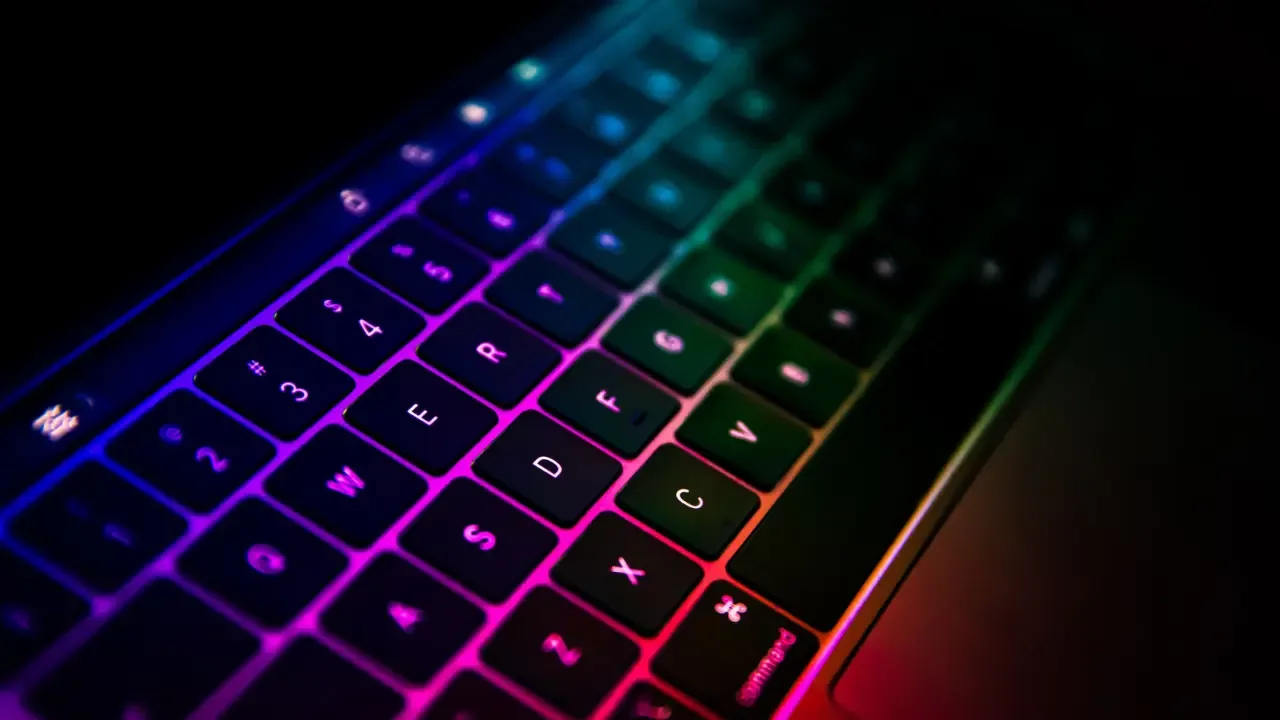
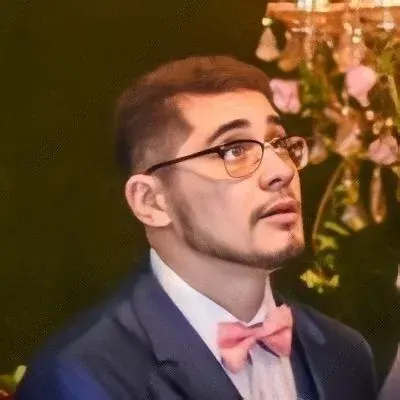
How to Find the š± Topmost View Controller on iOS
š Have you ever found yourself in a situation where you needed to access the topmost view controller in your iOS app, but couldn't figure out how to do it? This can be quite a challenge, especially if you are executing in a class that is not a view controller and do not have the address of an active view. But worry not, we've got you covered! In this blog post, we will explore different scenarios and provide easy solutions to help you find the topmost view controller or view on iOS. š
The Challenge šÆ
The challenge at hand is that you want to find the view controller that is responsible for the current view, without having direct access to it. This can happen when you are executing in a class that is not a view controller or a view, and you haven't been passed the address of the topmost view controller or the navigation controller. So, the main question is: how can we find that elusive topmost view controller or view? š¤
Solution 1: Key Window šŖ
One approach is to use the keyWindow
property of the UIApplication
class. The keyWindow
property represents the window that is currently receiving keyboard and other non-touch related events. By accessing the rootViewController
property of the keyWindow
, we can get the topmost view controller.
if let keyWindow = UIApplication.shared.keyWindow,
let rootViewController = keyWindow.rootViewController {
// rootViewController is the topmost view controller
// Use it to perform any actions or access its properties
}
This solution assumes that you have a single window in your app and that it has a root view controller set. Keep in mind that it might not work in complex app architectures or when you have multiple windows.
Solution 2: Key Window's Visible View Controller šš„
In some cases, the keyWindow
might not have the expected root view controller or it might not have been set up yet. In such scenarios, we can try another approach by accessing the visible view controller from the keyWindow
. This is useful when dealing with multi-window scenarios.
if let keyWindow = UIApplication.shared.keyWindow,
let topViewController = keyWindow.visibleViewController {
// topViewController is the topmost view controller
// Use it to perform any actions or access its properties
}
The visibleViewController
property returns the view controller that is currently visible on the topmost window.
Solution 3: Window Hierarchy š
Another approach to finding the topmost view controller is by traversing the window hierarchy. This method can be helpful if your app has multiple windows and you need to find the topmost view controller across all windows.
if let topViewController = UIApplication.shared.windows
.filter({ $0.isKeyWindow })
.first?
.rootViewController?
.topmostViewController {
// topViewController is the topmost view controller
// Use it to perform any actions or access its properties
}
Here, we use the windows
property of the UIApplication
class to get all the windows in the app. We filter the windows to find the key window and then retrieve its root view controller. Finally, we use an extension method or property called topmostViewController
to fetch the topmost view controller.
Solution 4: Delegate or Notification š£
In some cases, you might have the option to use a delegate pattern or a notification to pass the reference of the topmost view controller. This can be helpful when there are known entry points or specific actions in your app where the topmost view controller is updated.
For example, you could create a delegate protocol with a method like func topmostViewControllerDidChange(_ viewController: UIViewController)
or post a notification whenever the topmost view controller changes. This way, other classes or components can subscribe to the delegate method or notification to get the reference of the topmost view controller.
Solution 5: Revisit the Architecture š
If you find yourself consistently struggling with finding the topmost view controller or view, it might be worth revisiting the architecture of your app. Consider using design patterns like MVVM (Model-View-ViewModel) or coordinators to better manage your view controllers and their hierarchy.
These architectural approaches can provide clear separation of concerns and make it easier to access the topmost view controller when needed.
Conclusion š
Finding the topmost view controller or view on iOS can be a challenging task, especially when you don't have direct access to them. However, with the solutions mentioned above, you should be able to handle most scenarios successfully. Remember to choose the approach that best suits your app's architecture and requirements.
Now that you have learned how to find the topmost view controller, go ahead and implement it in your app. And if you come across any other obstacles or have additional tips to share, let us know in the comments below! š
š¢ Don't forget to share this blog post with your fellow iOS developers who might benefit from this handy guide. Happy coding! š