How to dismiss ViewController in Swift?
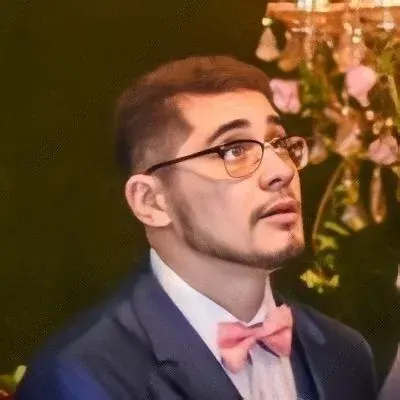
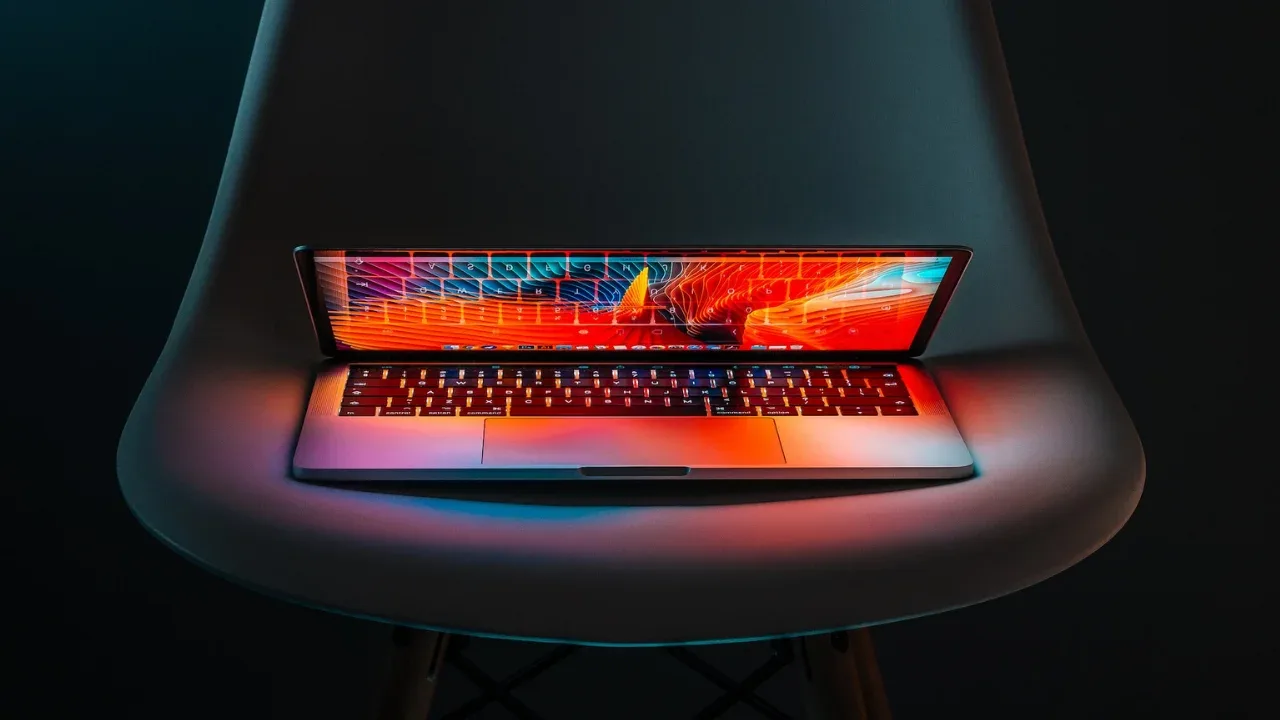
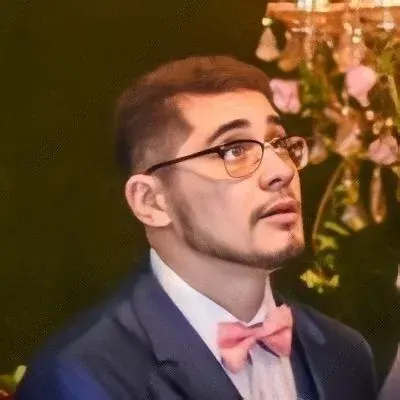
How to Dismiss ViewController in Swift? 🚀
If you're trying to dismiss a ViewController in Swift but it's not working as expected, don't worry! You're not alone. Many developers face this issue, but luckily, there's a simple solution.
The Problem ⚠️
In the code snippet you provided, you're using the dismissViewControllerAnimated
method to dismiss the ViewController when the cancel
and done
actions are triggered. However, even though you see the println message in the console output, the ViewController never gets dismissed.
The Solution 💡
The issue lies in passing false
as the first parameter to the dismissViewControllerAnimated
method. By passing false
, you're telling the system not to animate the dismissal.
To fix this problem, you have two options:
Option 1: Animated Dismissal ✨
If you want to dismiss the ViewController with an animation, set the first parameter to true
.
@IBAction func cancel(sender: AnyObject) {
self.dismissViewControllerAnimated(true, completion: nil)
print("cancel")
}
@IBAction func done(sender: AnyObject) {
self.dismissViewControllerAnimated(true, completion: nil)
print("done")
}
By setting the first parameter to true
, the ViewController will be dismissed with a smooth animation.
Option 2: Immediate Dismissal 🚫
If you want to dismiss the ViewController instantly without any animation, you can remove the completion block and call dismissViewControllerAnimated
directly with no parameters.
@IBAction func cancel(sender: AnyObject) {
self.dismissViewControllerAnimated()
print("cancel")
}
@IBAction func done(sender: AnyObject) {
self.dismissViewControllerAnimated()
print("done")
}
By removing the completion block and passing no parameters, the ViewController will be dismissed immediately, without any animation.
Takeaways 🎯
Remember to consider whether you want an animated or immediate dismissal when using the dismissViewControllerAnimated
method. By choosing the appropriate option and passing the correct parameters, you can successfully dismiss ViewControllers in Swift.
Still Having Issues? 🆘
If you're still facing problems with dismissing ViewControllers, feel free to leave a comment below. Our community is always here to help you out!
Was this guide helpful? Let us know in the comments below! If you found it useful, don't forget to share it with other developers who might be experiencing the same issue. Happy coding! 😄🚀