How to detect if app is being built for device or simulator in Swift
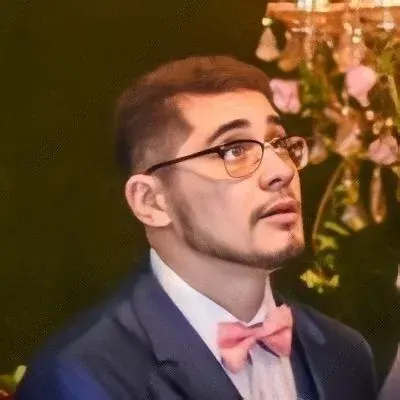
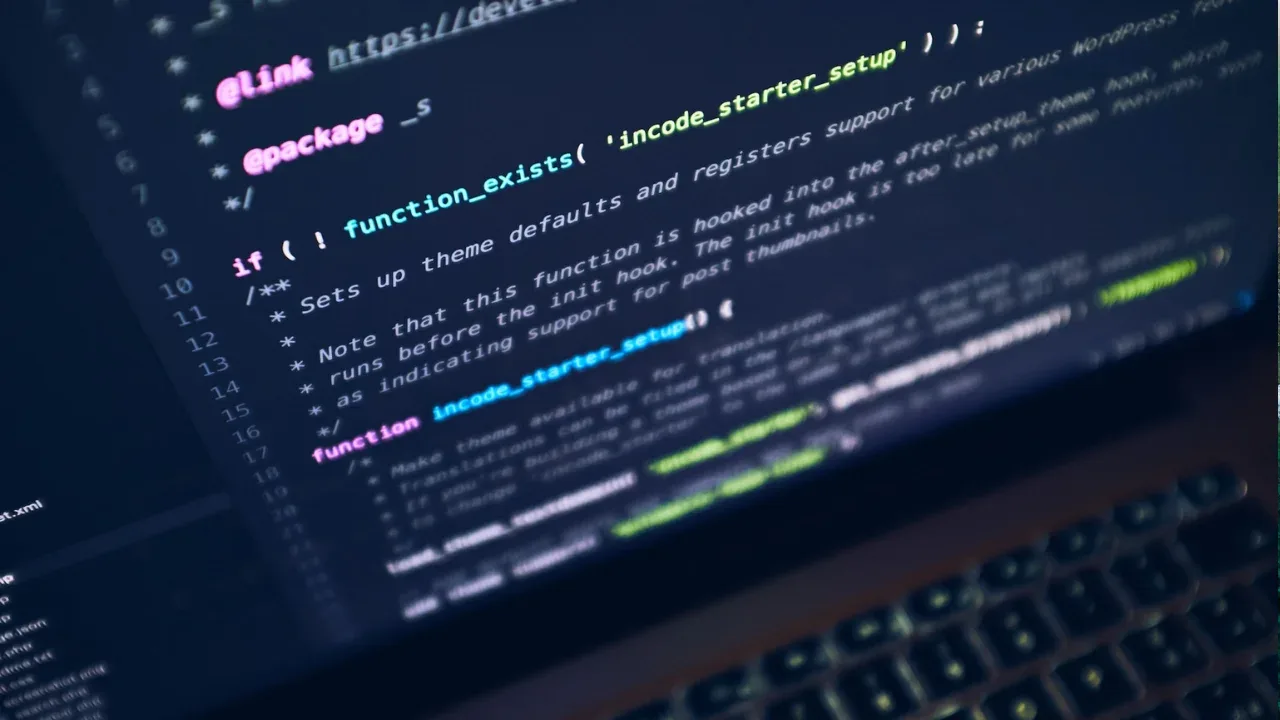
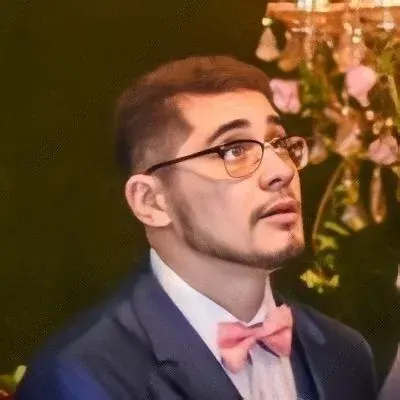
How to Detect if an app is Being Built for Device or Simulator in Swift ๐๐ฑ๐ป
Are you a Swift developer struggling to figure out if your app is being built for a device or a simulator? Look no further! In this guide, we'll explore different ways to solve this problem and give you quick and easy solutions. Let's dive in! ๐โโ๏ธ๐โโ๏ธ
The Objective-C Approach ๐งช
In Objective-C, developers can determine if an app is being built for a device or simulator by using macros. The approach looks like this:
#if TARGET_IPHONE_SIMULATOR
// Simulator
#else
// Device
#endif
These macros are evaluated at compile time and are not available at runtime. But don't worry, we have a solution for you in Swift! ๐๐ง
Solution 1: Using Runtime Checks and Environment Variables ๐ต๏ธโโ๏ธ๐
One way to detect if an app is being built for a device or simulator in Swift is by using runtime checks and environment variables. Here's how you can do it:
Create a new Swift file in your Xcode project, let's call it
EnvironmentChecker.swift
.In this file, define a static function named
isRunningOnSimulator
that returns aBool
indicating whether it's a simulator or not. Your code should look like this:
import Foundation
class EnvironmentChecker {
static var isRunningOnSimulator: Bool {
#if targetEnvironment(simulator)
return true
#else
return false
#endif
}
}
To check if the app is running on a simulator, you can call
EnvironmentChecker.isRunningOnSimulator
. For example:
if EnvironmentChecker.isRunningOnSimulator {
print("Running on a Simulator!")
} else {
print("Running on a Device!")
}
And voila! You now have a way to distinguish between a device and a simulator in your Swift app. ๐คฉ๐
Solution 2: Using UIDevice.current.userInterfaceIdiom ๐ฒ๐ฑ
Another approach to determine if your app is running on a device or simulator is by using the UIDevice
class. Here's how you can do it:
Access the
userInterfaceIdiom
property of theUIDevice.current
object.Compare it with the
.pad
or.phone
case to determine whether it's a simulator or a device. Here's an example:
if UIDevice.current.userInterfaceIdiom == .pad {
print("Running on an iPad Simulator or Device!")
} else if UIDevice.current.userInterfaceIdiom == .phone {
print("Running on an iPhone Simulator or Device!")
} else {
print("Running on an Unknown Device!")
}
This method is straightforward and does not require any additional setup. ๐๐
Conclusion and Action Time! ๐๐ค
In this blog post, we walked you through two simple solutions to detect if an app is being built for a device or simulator in Swift. Remember, you can use the runtime checks and environment variables method or the UIDevice.current.userInterfaceIdiom
property to get the job done.
Now it's your turn to try these solutions yourself! Implement them in your Swift projects and let us know which one worked best for you. Share your experiences and any other tips and tricks you have in the comments below. We'd love to hear from you! ๐ค๐ฌ
Happy coding! ๐๐ฉโ๐ป๐จโ๐ป