How to deselect a selected UITableView cell?
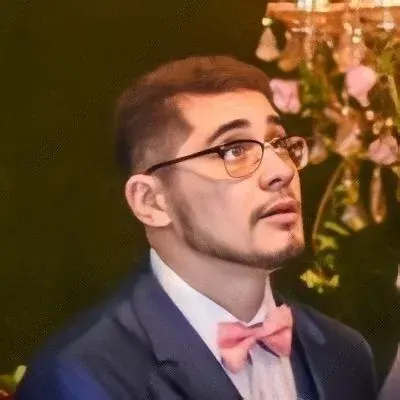
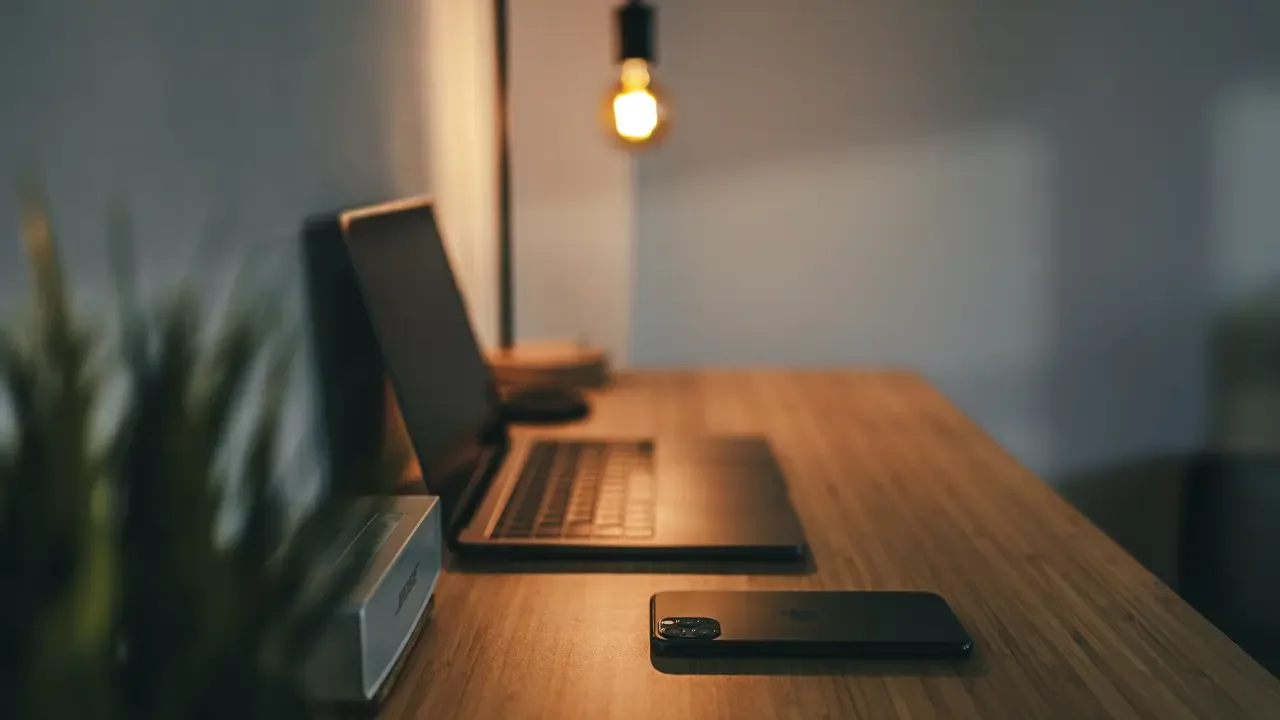
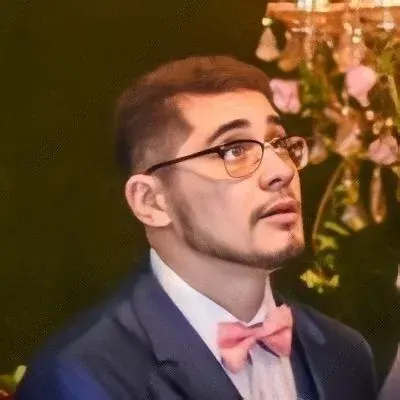
📱 How to Deselect a Selected UITableView Cell? 🔄
Are you trying to figure out how to deselect a selected cell in your UITableView? 🤔 Don't worry, we've got you covered! In this blog post, we'll address common issues and provide easy solutions to help you accomplish this task. Let's jump right in! 💪
Problem: Unable to Deselect a Preselected Cell
In the given context, the user wants to preselect a particular cell in their UITableView using -willDisplayCell
. However, they're facing difficulty in deselecting it when the user clicks on any other cell. This can be an interesting challenge, but fret not! We'll guide you through it step by step. 💡
Solution: Implementing didSelectRowAtIndexPath
The didSelectRowAtIndexPath
method is called when the user taps on a cell. To achieve the desired behavior, we need to modify this method. Follow the code snippet below to update your implementation: 👇
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
AppDelegate_iPad *appDelegate = (AppDelegate_iPad *)[[UIApplication sharedApplication] delegate];
NSIndexPath *previousIndexPath = appDelegate.indexPathDelegate;
appDelegate.indexPathDelegate = indexPath;
[tableView reloadRowsAtIndexPaths:@[previousIndexPath, indexPath] withRowAnimation:UITableViewRowAnimationNone];
}
Here's a breakdown of what the code does:
We retrieve the
AppDelegate
object and store the previously selected index path inpreviousIndexPath
.Next, we update the
indexPathDelegate
with the new index path.Finally, we reload both the previous and current index paths using
reloadRowsAtIndexPaths
to reflect the changes.
Make sure to replace tableView
with the actual name of your table view object. 😉
Extra Step: Deselecting the Cell
The code we provided will deselect the previously selected cell when the user taps on a new cell. However, to visually reflect the deselection, we need to update our willDisplayCell
method as well. Check out the updated code snippet below: 👇
- (void)tableView:(UITableView *)tableView willDisplayCell:(UITableViewCell *)cell forRowAtIndexPath:(NSIndexPath *)indexPath {
AppDelegate_iPad *appDelegate = (AppDelegate_iPad *)[[UIApplication sharedApplication] delegate];
if ([appDelegate.indexPathDelegate isEqual:indexPath]) {
[cell setSelected:YES animated:NO];
[tableView selectRowAtIndexPath:indexPath animated:NO scrollPosition:UITableViewScrollPositionNone];
} else {
[cell setSelected:NO animated:NO];
}
}
The modifications here:
We retrieve the
AppDelegate
object and check if the current cell's index path matches the selected index path stored inindexPathDelegate
.If there's a match, we select the cell and scroll to it if necessary using
selectRowAtIndexPath
.If the index paths don't match, we simply deselect the cell.
Voilà! 🎉 With these changes in place, you should now be able to deselect a preselected cell whenever a user taps on another cell.
Call to Action: Share Your Experience
We hope this guide was helpful in solving your issue! If you have any additional questions or thoughts, feel free to share them in the comments section below. We'd love to hear about your experience and how you applied this solution in your project. Happy coding! 💻😄
Note: Don't forget to replace "AppDelegate_iPad" with your actual AppDelegate class name if applicable.