How to create NSIndexPath for TableView
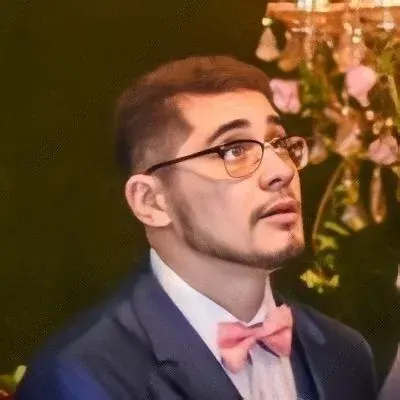
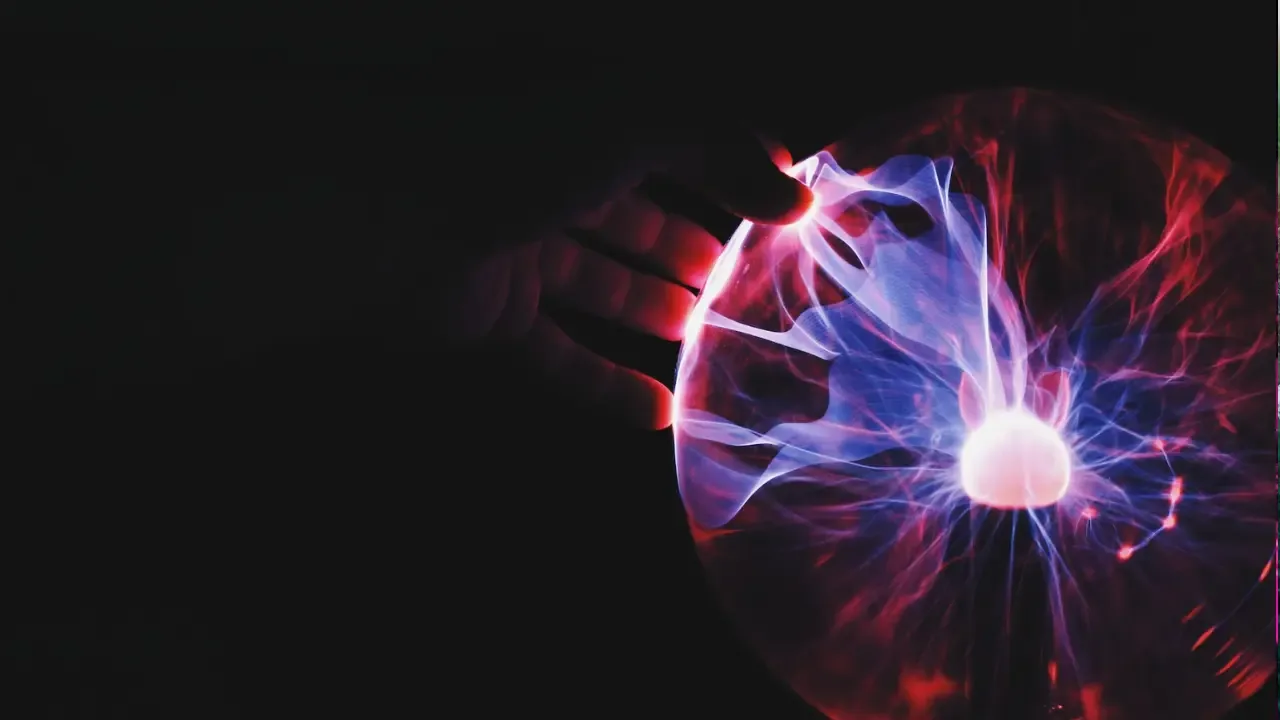
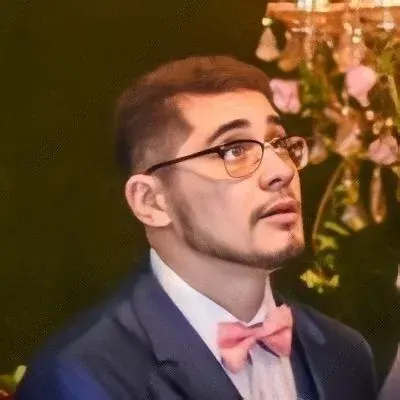
📝 Creating NSIndexPath for TableView: A Handy Guide
So you want to delete a row in your table, huh? But you're stuck because you need to use an NSIndexPath with a section and row defined for the deleteRowAtIndexPath. Fear not, my friend! I've got you covered with this easy guide on how to create the NSIndexPath you need.
🤔 The Problem
In order to use deleteRowAtIndexPath, you must pass an NSIndexPath that specifies the section and row of the cell you want to delete. But what if you only have the row information and no section? That's where the trouble begins.
❌ The Crash
You might have tried creating an NSIndexPath with an array that contains only the row number, like [1], but ended up with a crash. The NSLog message probably said something about the section needing to be defined as well. Annoying, right?
🔧 The Solution
To create the NSIndexPath you need, you can use the indexPathForRow:inSection: method. This handy method allows you to specify the row and section values, giving you a correctly formatted NSIndexPath.
✅ The Code
Let's break it down with an example using your code snippet:
NSIndexPath *myIP = [[NSIndexPath alloc] indexPathForRow:0 inSection:0];
NSArray *myArray = [[NSArray alloc] initWithObjects:myIP, nil];
[self.tableView deleteRowsAtIndexPaths:myArray withRowAnimation:UITableViewRowAnimationFade];
In this code, we first create an NSIndexPath using the indexPathForRow:inSection: method. The row is set to 0 and the section is also set to 0. Feel free to change these values to fit your needs.
Then, we create an NSArray called myArray and add the newly created NSIndexPath as its only member.
Finally, we call the deleteRowsAtIndexPaths method on our tableView, passing in myArray as the indexPaths to delete. You can also specify how the animation should be done with the UITableViewRowAnimation option.
🚀 Engaging Call-to-Action
And there you have it! Now you know how to create the NSIndexPath you need to delete a row in your table. Go ahead and give it a try in your code. If you have any further questions or run into any issues, feel free to leave a comment below. Happy coding! 💻🎉
Note: Make sure to uncomment the beginUpdates and endUpdates lines if you're performing multiple delete operations in a batch for smoother animation.