How to create dispatch queue in Swift 3
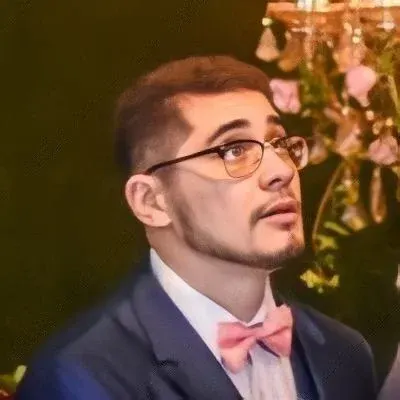
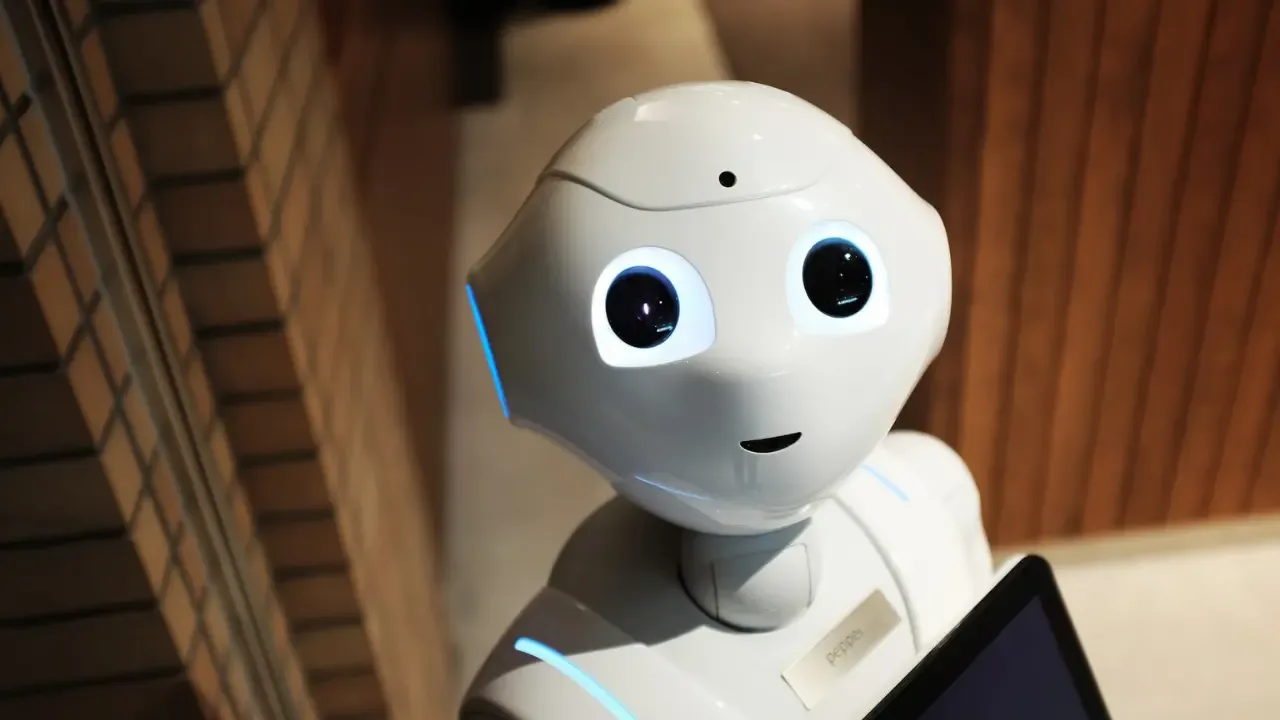
Creating a Dispatch Queue in Swift 3: A Comprehensive Guide 🚀
Are you experiencing issues creating a dispatch queue in Swift 3? Don't worry, we've got you covered! In this guide, we will address the common issues and provide easy solutions to create a dispatch queue effectively. Let's dive in! 💪
Understanding the Problem 🤔
In Swift 2, creating a dispatch queue was straightforward, using the dispatch_queue_create
function. However, with the release of Swift 3, this method became obsolete and no longer compiles. This can be frustrating, especially when you need to handle asynchronous tasks efficiently.
The Preferred Way in Swift 3 ⭐️
To create a dispatch queue in Swift 3, you need to use the DispatchQueue
class and its static property label
. The label
serves as the identifier for the dispatch queue and helps you control the execution of tasks more effectively. Here's the updated code:
let customQueue = DispatchQueue(label: "com.swift3.imageQueue", attributes: .concurrent)
In the example above, we created a custom dispatch queue named "com.swift3.imageQueue" with the .concurrent
attribute. The .concurrent
attribute allows multiple tasks to run simultaneously, making it useful for handling tasks that can be executed concurrently.
Understanding Attributes 🌟
When creating a dispatch queue, you have the flexibility to choose between different attributes. Here are three commonly used attributes:
.concurrent
: This attribute allows multiple tasks to run simultaneously on the dispatch queue..serial
: This attribute ensures that only one task can run at a time in a specific queue..main
: This attribute specifies the main dispatch queue, which is responsible for updating the user interface.
Choose the appropriate attribute based on your specific requirements to optimize the performance of your app.
Extra Tips to Enhance the Dispatch Queue Usage 💡
To better utilize dispatch queues, consider the following tips:
Use a DispatchQueue Extension: Create an extension that adds convenience methods to your queues for better readability and overall code cleanliness.
Dispatch Work Items: Use the
DispatchWorkItem
class to encapsulate your tasks. Work items provide additional control and monitoring features, such as the ability to cancel tasks or handle task dependencies.
Now that you have a clear understanding of how to create a dispatch queue in Swift 3, it's time to level up your app's performance and responsiveness! 💥
Have any questions or thoughts? Let us know in the comments below. We're here to help! 🙌
Happy coding! 👩💻👨💻
Note: This blog post assumes you have basic knowledge of Swift. If you're new to Swift, don't worry! There are plenty of resources available online to get you up to speed.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
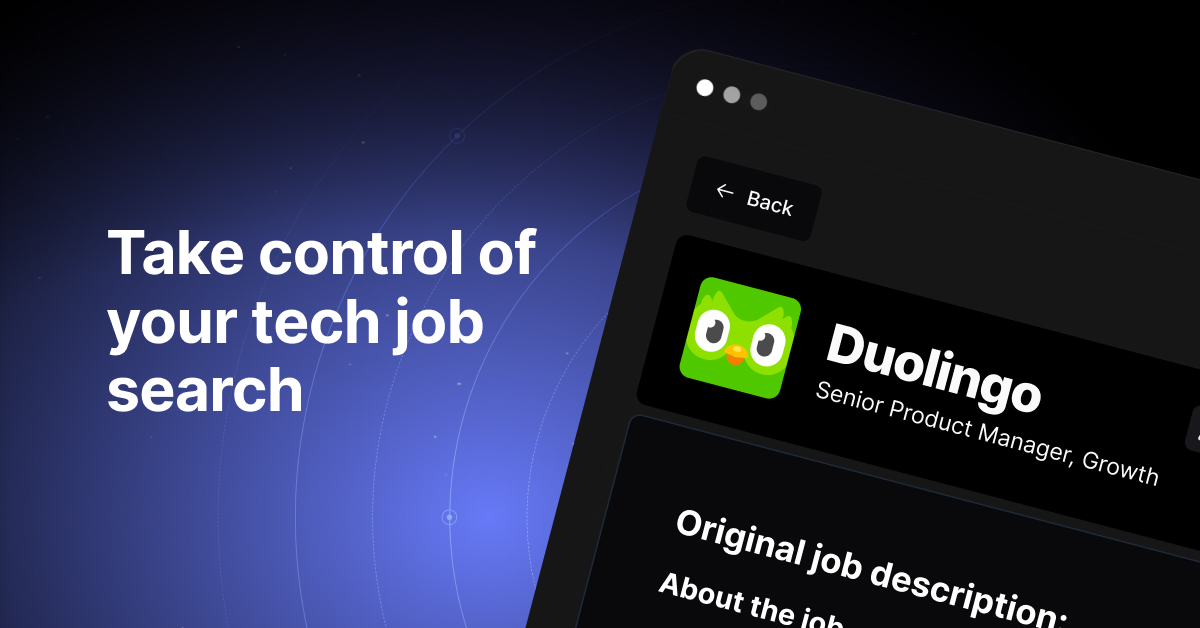