How to create a delay in Swift?
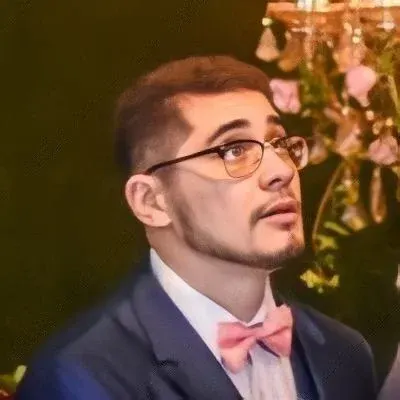
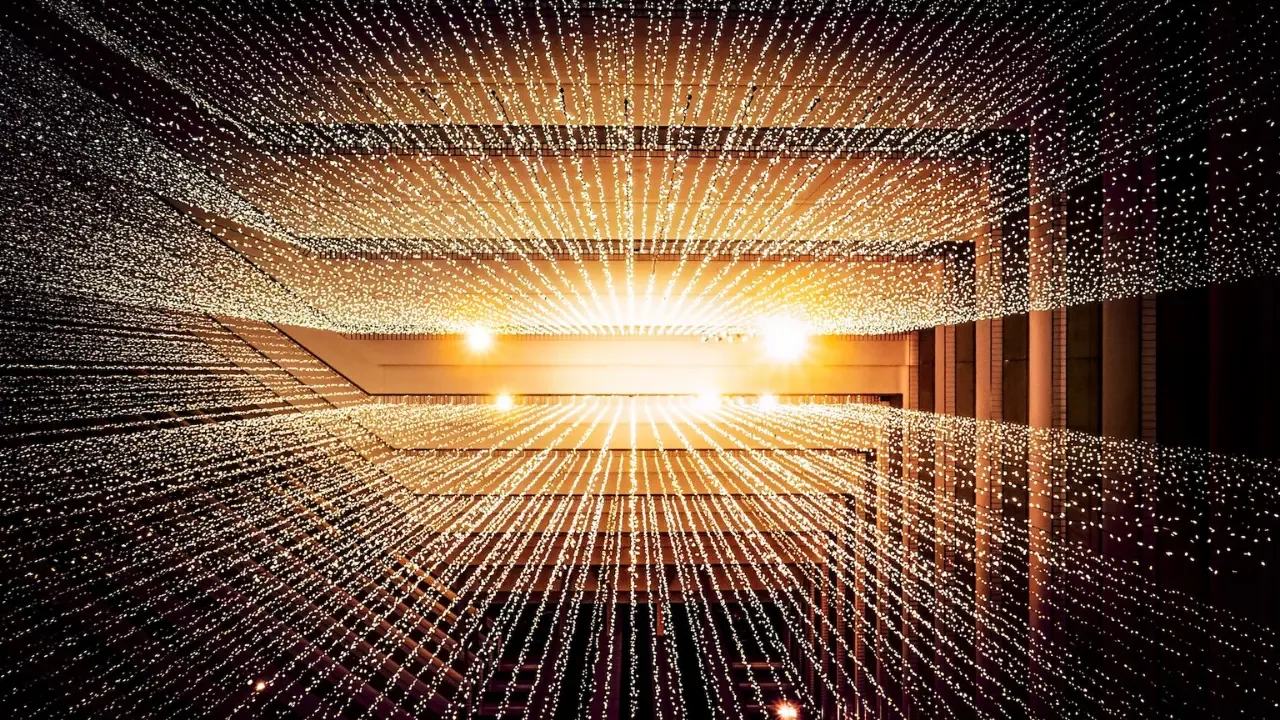
π How to Create a Delay in Swift? A Guide to Pausing Your App's Execution π
As a Swift developer, there may be times when you need to create a delay in your app's execution. Whether you want to show a loading indicator, perform a specific action after a certain amount of time, or create a smooth user experience, implementing a delay can be incredibly useful. In this guide, we'll explore different ways to create delays in Swift, so you can effectively control the flow of your app. Let's dive in! β±οΈ
The Problem: Pausing App Execution at a Certain Point βΈοΈ
Imagine you're building an app and you want to pause its execution at a specific point. For example, you want your app to execute a code block, then pause for 4 seconds, and continue with the rest of the code. How can you achieve this in Swift? π€
Solution 1: DispatchQueue.main.asyncAfter β³
One way to create a delay in Swift is by using DispatchQueue.main.asyncAfter
. This method allows us to schedule a block of code to be executed after a specified delay. Here's an example:
DispatchQueue.main.asyncAfter(deadline: .now() + 4) {
// Code to be executed after a 4-second delay
// This could be anything you want your app to do after the pause
}
In this example, we use DispatchQueue.main.asyncAfter
to schedule our code block to be executed after 4 seconds. The code you want to execute after the delay goes inside the closure.
Solution 2: Timer β²οΈ
Another way to create a delay in Swift is by utilizing the Timer
class. With Timer
, you can schedule a block of code to be executed periodically or just once, after a certain delay. Here's how you can use it to create a delay:
Timer.scheduledTimer(withTimeInterval: 4, repeats: false) { _ in
// Code to be executed after a 4-second delay
// Add your desired actions or functions here
}
In this example, we create a Timer
that will fire after 4 seconds. Once the timer fires, the code inside the closure will be executed. Setting repeats
to false
ensures that the timer fires only once.
Solution 3: DispatchSemaphore βοΈ
If you need more control over the timing of your delays, you can use a DispatchSemaphore
. A semaphore is a synchronization primitive that allows or prevents access to a resource depending on its count value. In our case, we can make use of a DispatchSemaphore
to create a custom delay. Here's an example:
let semaphore = DispatchSemaphore(value: 0)
// Perform any actions before the delay
// Pause execution for 4 seconds
_ = semaphore.wait(timeout: .now() + 4)
// Resume execution after the delay
// Code to be executed after a 4-second delay
In this example, we create a DispatchSemaphore
with an initial value of 0. We then use semaphore.wait(timeout:)
to pause execution for 4 seconds. Once the timeout expires, the code after semaphore.wait
is executed.
Conclusion and Call-to-Action βοΈ
Creating delays in Swift is essential for controlling the flow of your app and providing a smoother user experience. In this guide, we explored three different solutions: DispatchQueue.main.asyncAfter
, Timer
, and DispatchSemaphore
. Depending on your specific needs, you can choose the most appropriate method for your app.
Now it's your turn to try implementing delays in your Swift projects! Experiment with these solutions and see how they can enhance your app's functionality. Don't be afraid to get creative with the delays and incorporate them into your own unique features.
If you found this guide helpful, feel free to share it with your fellow Swift developers, and let me know your thoughts in the comments below. Happy coding! ππ»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
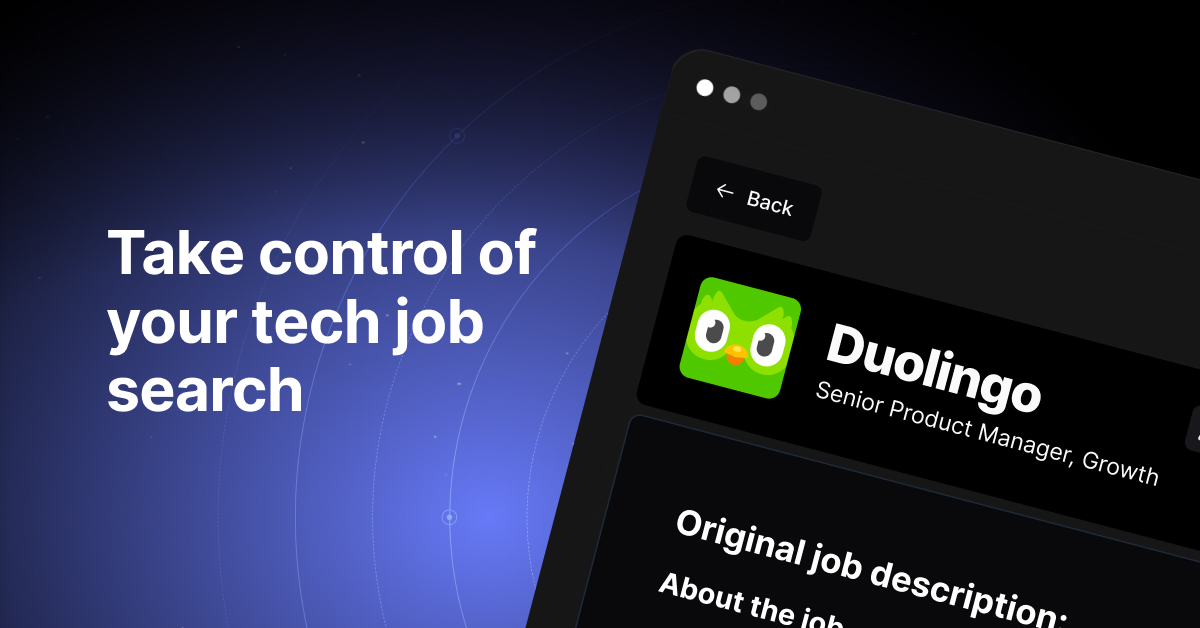