How to check if a file exists in Documents folder?
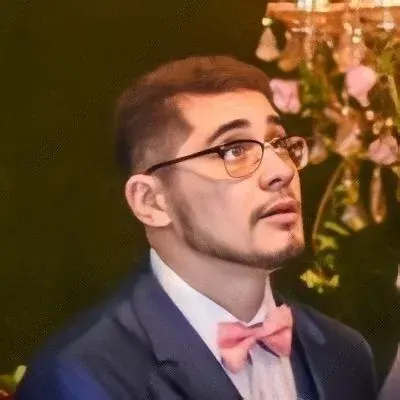
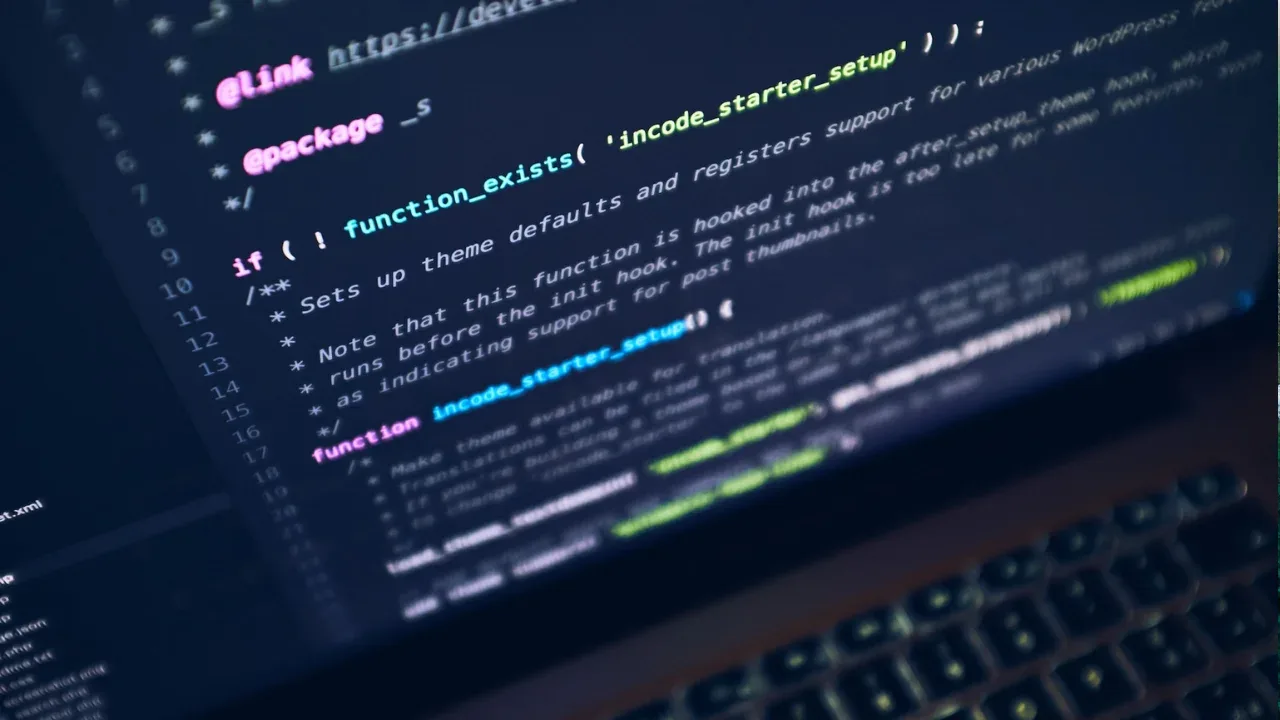
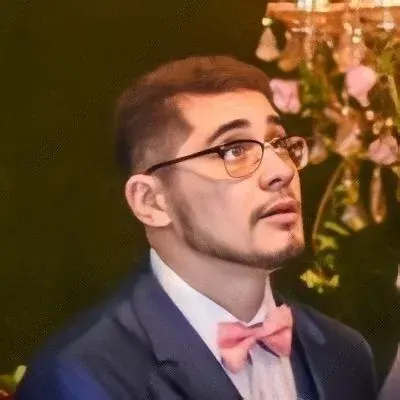
How to Check if a File Exists in Documents Folder? 📂📄
So, you have an application with In-App Purchase, and upon purchase, you download an HTML file into the Documents folder of your app. However, you need to check if this file exists before loading it, otherwise, you want to load your default HTML page. 📥💻
But wait! The NSFileManager
in iOS only lets you access files within the mainBundle
by default. So, how can you check if the file exists in the Documents folder? 🤔
Not to worry! In this guide, we'll walk you through the steps to determine if a file exists in the Documents folder and provide easy solutions to help you along the way. Let's get started! 🚀
Step 1: Get the Path to the Documents Folder 📂
To begin, we need to obtain the path to the Documents folder. The path will serve as the starting point for checking if the file exists. You can use the following code snippet to accomplish this:
guard let documentsDirectory = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first else {
// Handle the absence of the Documents folder
return
}
In the above code, we used FileManager.default.urls(for:in:)
to fetch an array of URLs for the specified search path domain mask. By using .documentDirectory
as the search path, we limit the results to the Documents folder. The guard
statement ensures that we have a valid URL to proceed.
Step 2: Check if the File Exists 🕵️♀️
With the path to the Documents folder in hand, we can proceed to check if the file exists. The most straightforward approach is to use the FileManager
's fileExists(atPath:)
method. Here's an example of how you can accomplish this:
let filePath = documentsDirectory.appendingPathComponent("yourFile.html").path
if FileManager.default.fileExists(atPath: filePath) {
// The file exists, load your HTML file
// Your code here
} else {
// The file doesn't exist, load your default HTML page
// Your code here
}
In the above code snippet, we utilize documentsDirectory.appendingPathComponent("yourFile.html").path
to get the full file path by appending the file name to the Documents directory path. We then use FileManager.default.fileExists(atPath:)
to determine if the file exists at the given path.
Step 3: Handle the Results ✅❌
Depending on whether the file exists or not, you can now load the appropriate HTML page. If the file exists, load your downloaded HTML file using your preferred method. If it doesn't exist, load your default HTML page.
For example, you can use a UIWebView
to load the HTML files:
let webView = UIWebView(frame: CGRect(x: 0, y: 0, width: view.frame.width, height: view.frame.height))
if FileManager.default.fileExists(atPath: filePath) {
let url = URL(fileURLWithPath: filePath)
let request = URLRequest(url: url)
webView.loadRequest(request)
} else {
let defaultURL = Bundle.main.url(forResource: "default", withExtension: "html")
let request = URLRequest(url: defaultURL)
webView.loadRequest(request)
}
view.addSubview(webView)
In the above example, we create a UIWebView
instance and conditionally load the appropriate HTML file based on whether the file exists or not.
Engage and Share! 📢🎉
Congratulations! Now you know how to check if a file exists in the Documents folder and load the appropriate HTML file in your iOS app. Feel free to experiment and adapt the code to fit your specific requirements.
Share your success with others by letting them know about this handy guide! If you found this blog post helpful, don't hesitate to share it with your fellow developers. Happy coding! 💻🙌
Do you have any other questions or need further assistance? Drop your queries in the comments below, and we'll be glad to help you out! Let's keep the conversation going. 🗣️💬