How to change the background color of a UIButton while it"s highlighted?
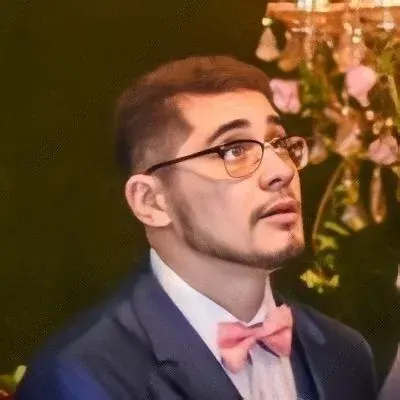
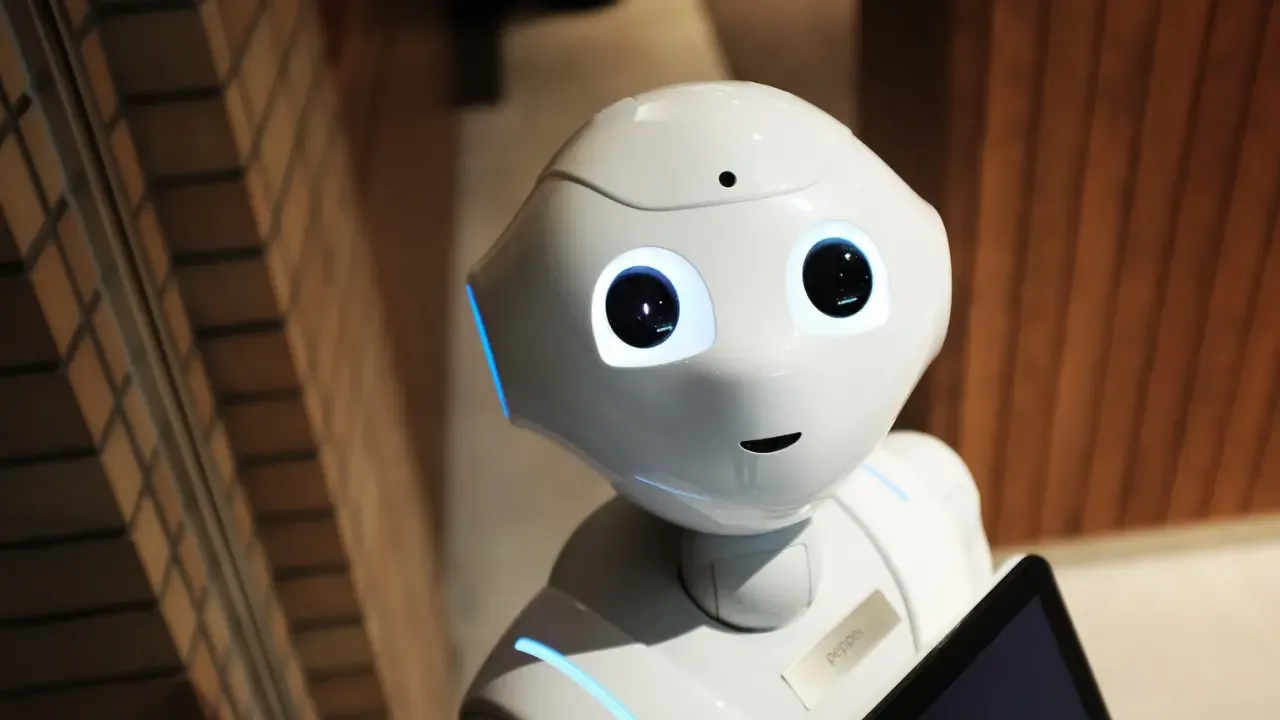
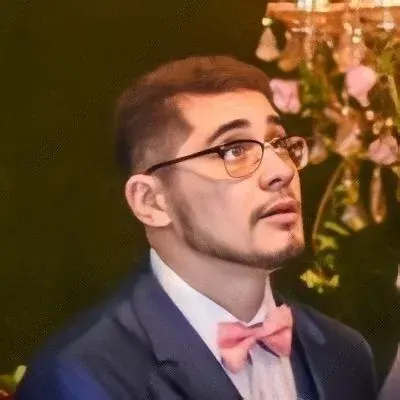
How to change the background color of a UIButton while it's highlighted?
Have you ever encountered the requirement to change the background color of a UIButton
when it's highlighted? 🤔 It may seem like a simple task, but it can be a bit tricky to get it working as expected. 😅 In this blog post, we'll address this common issue and provide you with easy and effective solutions. Let's get started! 💪
The Problem
Imagine you have a button in your app, and you want to change its background color as soon as the user touches and holds the button. However, when you try to set the background color of the button programmatically, it doesn't update as expected. 😫 Frustrating, right? But fret not, we'll help you resolve this issue step-by-step! 🚀
Solution 1: UIControlState
The most common way to change the background color of a UIButton
while it's highlighted is by using the UIControlState.highlighted
state. This state represents when the button is actively being touched. 🤏
To achieve the desired effect, you can use the following code snippet:
button.setBackgroundColor(.red, for: .highlighted)
👉 Make sure to replace .red
with your desired color.
This solution sets the background color for the .highlighted
state of the button. When the button is pressed and held, it will display the specified background color.
Solution 2: Button's Highlighted Property
Another way to change the background color of a UIButton
while it's highlighted is by leveraging the button's isHighlighted
property. 😎
Here's an example in Swift:
button.addTarget(self, action: #selector(onButtonTouchDown), for: .touchDown)
button.addTarget(self, action: #selector(onButtonTouchUp), for: .touchUpInside)
button.addTarget(self, action: #selector(onButtonTouchUp), for: .touchUpOutside)
@objc func onButtonTouchDown() {
button.backgroundColor = .red
}
@objc func onButtonTouchUp() {
button.backgroundColor = .clear
}
In this scenario, we programmatically change the background color to .red
when the button is touched down and revert it back to .clear
when the button is released or when the touch moves outside the button's bounds.
Solution 3: Subclass UIButton
Sometimes, the above solutions might not work due to complex button interactions or custom button subclasses. In such cases, you might need to subclass UIButton
to override its behavior and handle the background color changes manually. 🎨
Here's a basic example in Swift:
class HighlightableButton: UIButton {
override var isHighlighted: Bool {
didSet {
backgroundColor = isHighlighted ? .red : .clear
}
}
}
In this solution, we create a subclass HighlightableButton
that overrides the isHighlighted
property. Whenever the isHighlighted
value changes, we update the background color accordingly.
Conclusion
Changing the background color of a UIButton
while it's highlighted doesn't have to be a headache anymore! 🙌 Use the right approach based on your specific context:
Change the background color for the highlighted state using `setBackgroundCo